-
Notifications
You must be signed in to change notification settings - Fork 3.5k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
[Doc] add explanations for Avro schema in Python client (#12914)
- This PR adds doc for #12516 - Preview looks good: 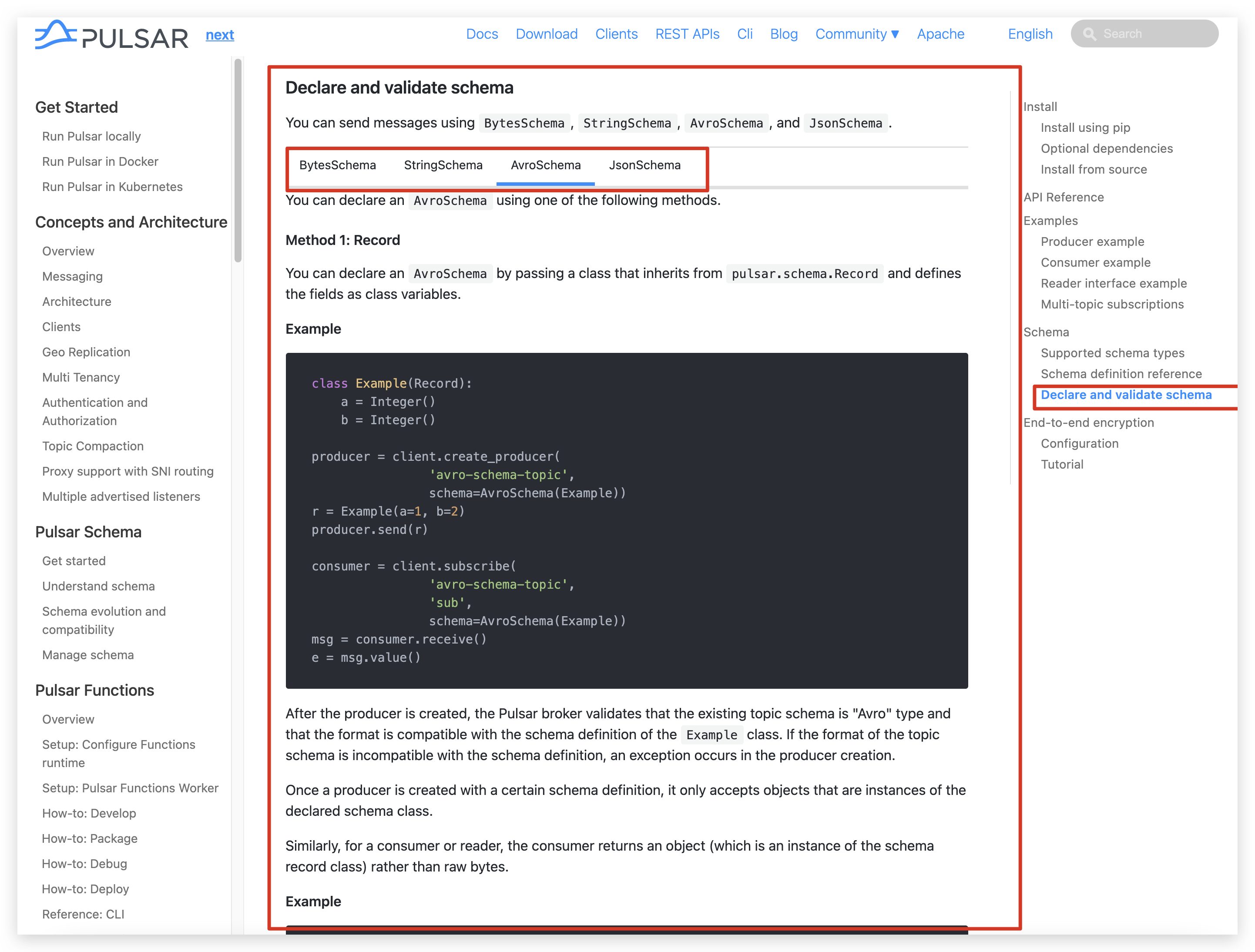
- Loading branch information
1 parent
32b697d
commit 093c230
Showing
1 changed file
with
192 additions
and
55 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters