-
Notifications
You must be signed in to change notification settings - Fork 253
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
SUBMARINE-442. Support get job's log in submarine-server REST API
### What is this PR for? Now we have the "jobs" resource in REST which can do CRUD. We also need a "logs" API to get the job's log output. The URI could be "api/v1/logs" It should accept parameters like "jobid". Initially, the logs could be aggregated logs of all containers. Streaming is preferred so that the python client can enable a fancy way for the end-user to check logs ### What type of PR is it? Feature ### Todos * [x] - get logs so far ### What is the Jira issue? https://issues.apache.org/jira/projects/SUBMARINE/issues/SUBMARINE-442 ### How should this be tested? Create a job Visit /api/v1/logs or /api/v1/logs/{jobid} with a browser ### Screenshots (if appropriate) http://127.0.0.1:8080/api/v1/jobs/logs 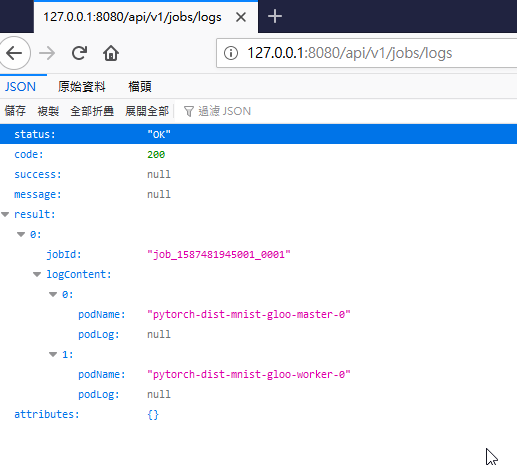 http://127.0.0.1:8080/api/v1/jobs/logs/job_1587481945001_0001 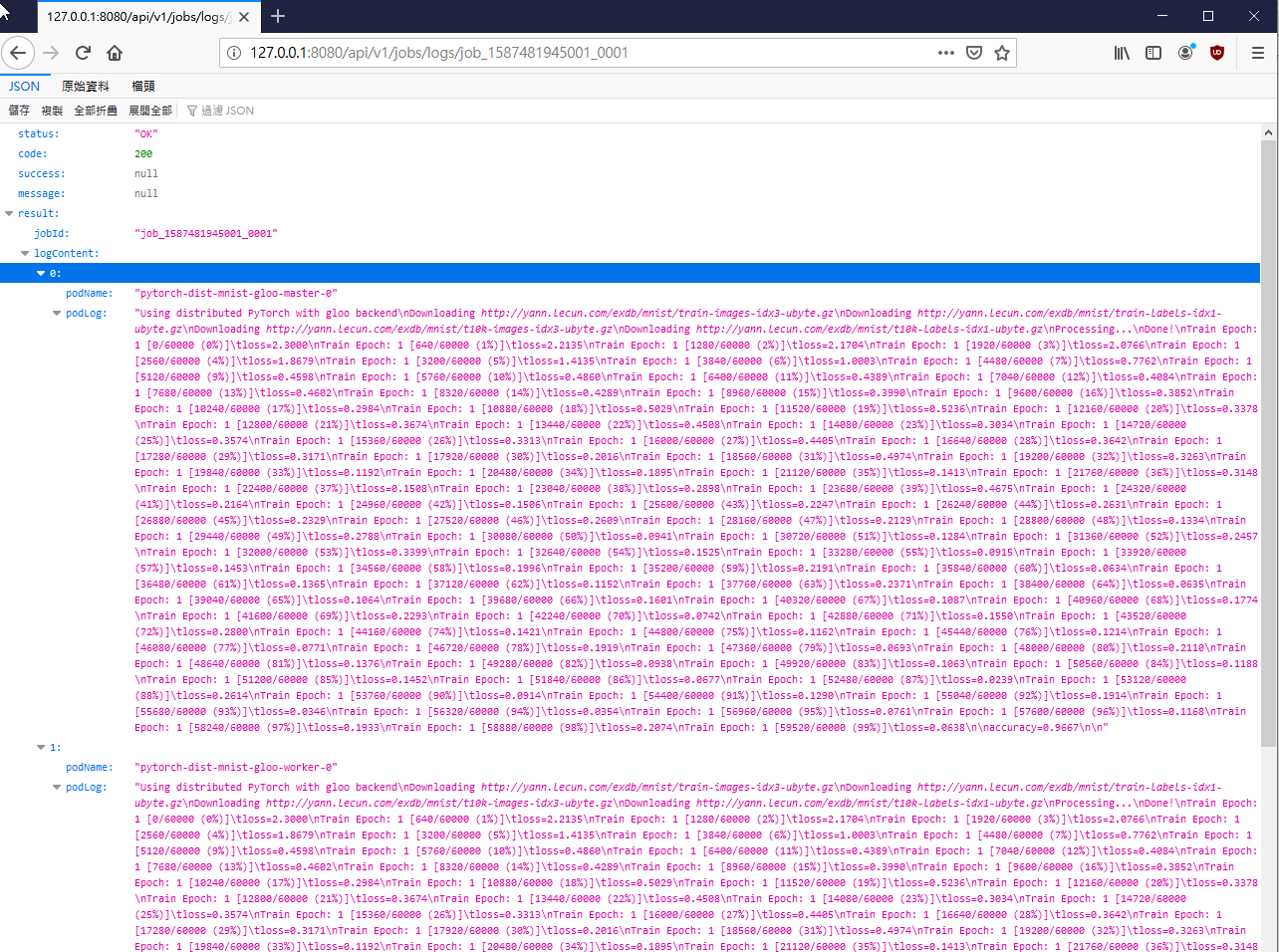 ### Questions: * Does the licenses files need update? No * Is there breaking changes for older versions? No * Does this needs documentation? No Author: JohnTing <jot.johnting@gmail.com> Closes #263 from JohnTing/SUBMARINE-442 and squashes the following commits: 7195a77 [JohnTing] test12 1bb806f [JohnTing] test12 319222c [JohnTing] SUBMARINE-442
- Loading branch information
Showing
8 changed files
with
234 additions
and
1 deletion.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
57 changes: 57 additions & 0 deletions
57
submarine-server/server-api/src/main/java/org/apache/submarine/server/api/job/JobLog.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,57 @@ | ||
/* | ||
* Licensed to the Apache Software Foundation (ASF) under one | ||
* or more contributor license agreements. See the NOTICE file | ||
* distributed with this work for additional information | ||
* regarding copyright ownership. The ASF licenses this file | ||
* to you under the Apache License, Version 2.0 (the | ||
* "License"); you may not use this file except in compliance | ||
* with the License. You may obtain a copy of the License at | ||
* | ||
* http://www.apache.org/licenses/LICENSE-2.0 | ||
* | ||
* Unless required by applicable law or agreed to in writing, | ||
* software distributed under the License is distributed on an | ||
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY | ||
* KIND, either express or implied. See the License for the | ||
* specific language governing permissions and limitations | ||
* under the License. | ||
*/ | ||
|
||
package org.apache.submarine.server.api.job; | ||
|
||
import java.util.ArrayList; | ||
import java.util.List; | ||
|
||
public class JobLog { | ||
private String jobId; | ||
private List<podLog> logContent; | ||
|
||
class podLog { | ||
String podName; | ||
String podLog; | ||
podLog(String podName, String podLog) { | ||
this.podName = podName; | ||
this.podLog = podLog; | ||
} | ||
} | ||
|
||
public JobLog() { | ||
logContent = new ArrayList<podLog>(); | ||
} | ||
|
||
public void setJobId(String jobId) { | ||
this.jobId = jobId; | ||
} | ||
|
||
public String getJobId() { | ||
return jobId; | ||
} | ||
|
||
public void addPodLog(String name, String log) { | ||
logContent.add(new podLog(name, log)); | ||
} | ||
|
||
public void clearPodLog() { | ||
logContent.clear(); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters