-
Notifications
You must be signed in to change notification settings - Fork 3.8k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat(iotevents): support SetVariable action (#19305)
This PR implememts `SetVariable` actions. This action allow to set variable to detector instanse. If use this action as following and deploy it: ```ts actions: [ new actions.IoteventsSetVariableAction( 'MyVariable', iotevents.Expression.inputAttribute(input, 'payload.temperature'), ), ], ``` And send message via cli as following: ``` aws iotevents-data batch-put-message --messages=messageId=(date | md5),inputName=test_input,payload=(echo '{"payload":{"deviceId":"000","temperature":18.5}}' | base64) ``` You can see the created detector instanse on web console: 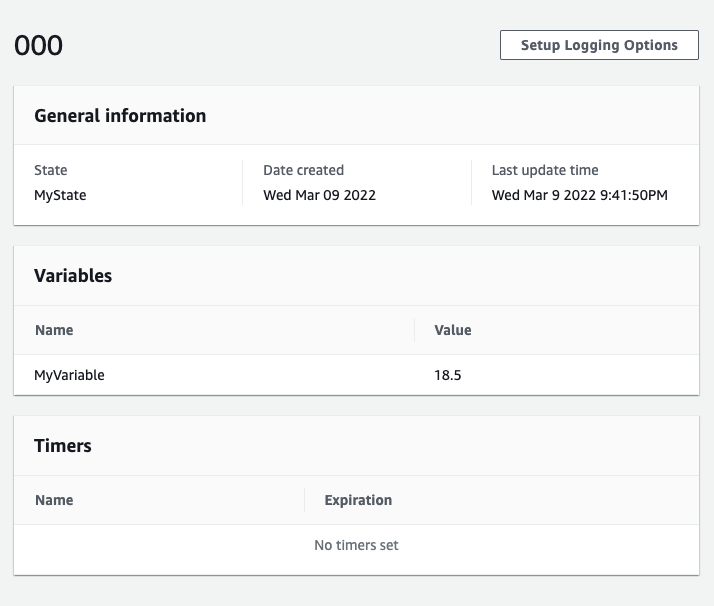 ---- *By submitting this pull request, I confirm that my contribution is made under the terms of the Apache-2.0 license*
- Loading branch information
Showing
6 changed files
with
235 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1 +1,2 @@ | ||
export * from './set-variable-action'; | ||
export * from './lambda-invoke-action'; |
25 changes: 25 additions & 0 deletions
25
packages/@aws-cdk/aws-iotevents-actions/lib/set-variable-action.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,25 @@ | ||
import * as iotevents from '@aws-cdk/aws-iotevents'; | ||
import { Construct } from 'constructs'; | ||
|
||
/** | ||
* The action to create a variable with a specified value. | ||
*/ | ||
export class SetVariableAction implements iotevents.IAction { | ||
/** | ||
* @param variableName the name of the variable | ||
* @param value the new value of the variable | ||
*/ | ||
constructor(private readonly variableName: string, private readonly value: iotevents.Expression) { | ||
} | ||
|
||
bind(_scope: Construct, _options: iotevents.ActionBindOptions): iotevents.ActionConfig { | ||
return { | ||
configuration: { | ||
setVariable: { | ||
variableName: this.variableName, | ||
value: this.value.evaluate(), | ||
}, | ||
}, | ||
}; | ||
} | ||
} |
95 changes: 95 additions & 0 deletions
95
packages/@aws-cdk/aws-iotevents-actions/test/iot/integ.set-variable-action.expected.json
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,95 @@ | ||
{ | ||
"Resources": { | ||
"MyInput08947B23": { | ||
"Type": "AWS::IoTEvents::Input", | ||
"Properties": { | ||
"InputDefinition": { | ||
"Attributes": [ | ||
{ | ||
"JsonPath": "payload.deviceId" | ||
}, | ||
{ | ||
"JsonPath": "payload.temperature" | ||
} | ||
] | ||
}, | ||
"InputName": "test_input" | ||
} | ||
}, | ||
"MyDetectorModelDetectorModelRoleF2FB4D88": { | ||
"Type": "AWS::IAM::Role", | ||
"Properties": { | ||
"AssumeRolePolicyDocument": { | ||
"Statement": [ | ||
{ | ||
"Action": "sts:AssumeRole", | ||
"Effect": "Allow", | ||
"Principal": { | ||
"Service": "iotevents.amazonaws.com" | ||
} | ||
} | ||
], | ||
"Version": "2012-10-17" | ||
} | ||
} | ||
}, | ||
"MyDetectorModel559C0B0E": { | ||
"Type": "AWS::IoTEvents::DetectorModel", | ||
"Properties": { | ||
"DetectorModelDefinition": { | ||
"InitialStateName": "MyState", | ||
"States": [ | ||
{ | ||
"OnEnter": { | ||
"Events": [ | ||
{ | ||
"Actions": [ | ||
{ | ||
"SetVariable": { | ||
"Value": { | ||
"Fn::Join": [ | ||
"", | ||
[ | ||
"$input.", | ||
{ | ||
"Ref": "MyInput08947B23" | ||
}, | ||
".payload.temperature" | ||
] | ||
] | ||
}, | ||
"VariableName": "MyVariable" | ||
} | ||
} | ||
], | ||
"Condition": { | ||
"Fn::Join": [ | ||
"", | ||
[ | ||
"currentInput(\"", | ||
{ | ||
"Ref": "MyInput08947B23" | ||
}, | ||
"\")" | ||
] | ||
] | ||
}, | ||
"EventName": "enter-event" | ||
} | ||
] | ||
}, | ||
"StateName": "MyState" | ||
} | ||
] | ||
}, | ||
"RoleArn": { | ||
"Fn::GetAtt": [ | ||
"MyDetectorModelDetectorModelRoleF2FB4D88", | ||
"Arn" | ||
] | ||
}, | ||
"Key": "payload.deviceId" | ||
} | ||
} | ||
} | ||
} |
42 changes: 42 additions & 0 deletions
42
packages/@aws-cdk/aws-iotevents-actions/test/iot/integ.set-variable-action.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,42 @@ | ||
/** | ||
* Stack verification steps: | ||
* * put a message | ||
* * aws iotevents-data batch-put-message --messages=messageId=(date | md5),inputName=test_input,payload=(echo '{"payload":{"temperature":31.9,"deviceId":"000"}}' | base64) | ||
*/ | ||
import * as iotevents from '@aws-cdk/aws-iotevents'; | ||
import * as cdk from '@aws-cdk/core'; | ||
import * as actions from '../../lib'; | ||
|
||
class TestStack extends cdk.Stack { | ||
constructor(scope: cdk.App, id: string, props?: cdk.StackProps) { | ||
super(scope, id, props); | ||
|
||
const input = new iotevents.Input(this, 'MyInput', { | ||
inputName: 'test_input', | ||
attributeJsonPaths: ['payload.deviceId', 'payload.temperature'], | ||
}); | ||
|
||
const state = new iotevents.State({ | ||
stateName: 'MyState', | ||
onEnter: [{ | ||
eventName: 'enter-event', | ||
condition: iotevents.Expression.currentInput(input), | ||
actions: [ | ||
new actions.SetVariableAction( | ||
'MyVariable', | ||
iotevents.Expression.inputAttribute(input, 'payload.temperature'), | ||
), | ||
], | ||
}], | ||
}); | ||
|
||
new iotevents.DetectorModel(this, 'MyDetectorModel', { | ||
detectorKey: 'payload.deviceId', | ||
initialState: state, | ||
}); | ||
} | ||
} | ||
|
||
const app = new cdk.App(); | ||
new TestStack(app, 'iotevents-set-variable-action-test-stack'); | ||
app.synth(); |
43 changes: 43 additions & 0 deletions
43
packages/@aws-cdk/aws-iotevents-actions/test/iot/set-variable-action.test.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,43 @@ | ||
import { Template } from '@aws-cdk/assertions'; | ||
import * as iotevents from '@aws-cdk/aws-iotevents'; | ||
import * as cdk from '@aws-cdk/core'; | ||
import * as actions from '../../lib'; | ||
|
||
let stack: cdk.Stack; | ||
let input: iotevents.IInput; | ||
beforeEach(() => { | ||
stack = new cdk.Stack(); | ||
input = iotevents.Input.fromInputName(stack, 'MyInput', 'test-input'); | ||
}); | ||
|
||
test('Default property', () => { | ||
// WHEN | ||
new iotevents.DetectorModel(stack, 'MyDetectorModel', { | ||
initialState: new iotevents.State({ | ||
stateName: 'test-state', | ||
onEnter: [{ | ||
eventName: 'test-eventName', | ||
condition: iotevents.Expression.currentInput(input), | ||
actions: [new actions.SetVariableAction('MyVariable', iotevents.Expression.fromString('foo'))], | ||
}], | ||
}), | ||
}); | ||
|
||
// THEN | ||
Template.fromStack(stack).hasResourceProperties('AWS::IoTEvents::DetectorModel', { | ||
DetectorModelDefinition: { | ||
States: [{ | ||
OnEnter: { | ||
Events: [{ | ||
Actions: [{ | ||
SetVariable: { | ||
VariableName: 'MyVariable', | ||
Value: 'foo', | ||
}, | ||
}], | ||
}], | ||
}, | ||
}], | ||
}, | ||
}); | ||
}); |