-
Notifications
You must be signed in to change notification settings - Fork 1
Push Notifications
Push notifications allow the user to receive alerts about important events while the app is not running. The initial implementation will only support Apple's APNS notification service for iOS and Google's FCM notification service for Android. Notifications are sent from the Bisq desktop app to a Bisq notification node that acts as proxy to the push notification service.
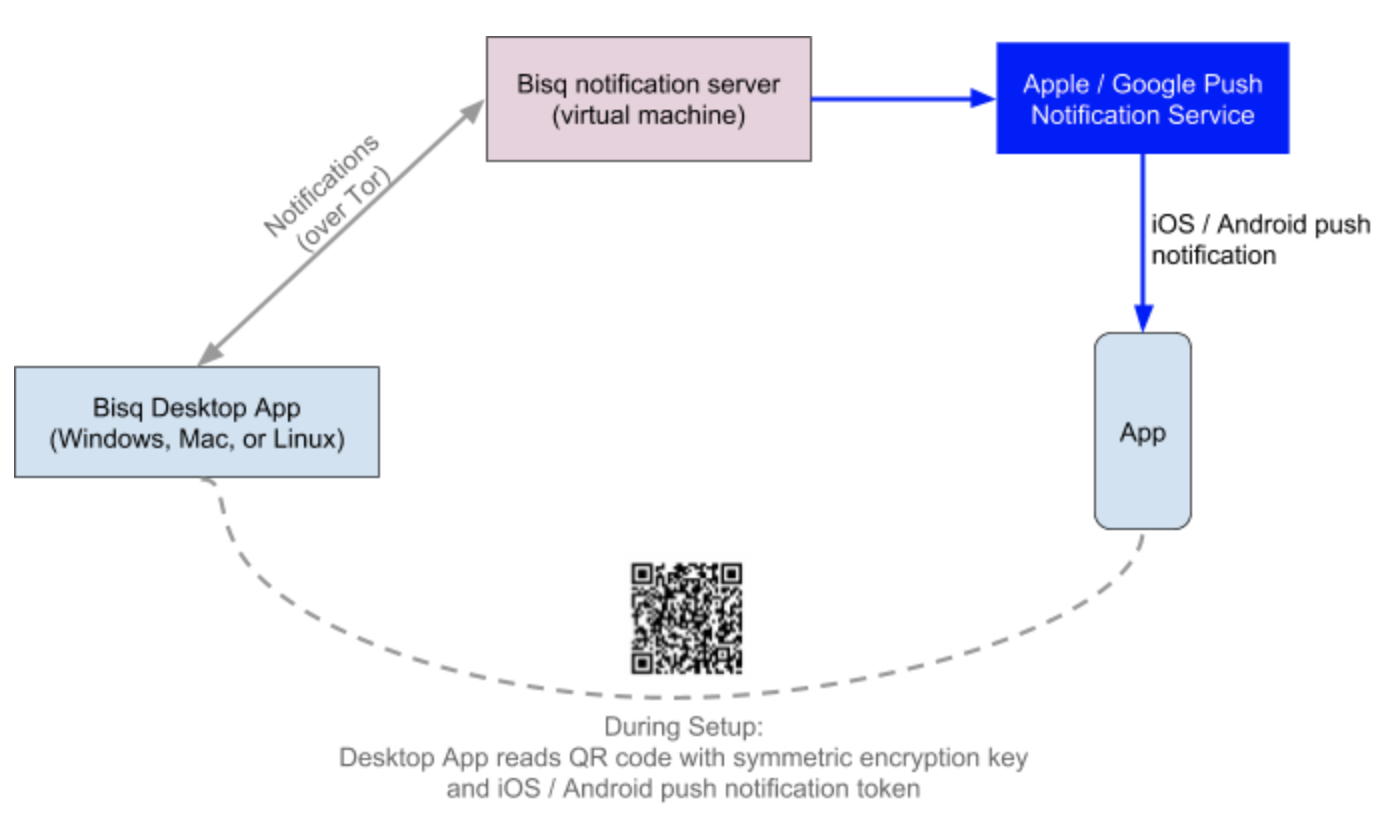
Figure 1: Push notification flow using the APNS or FCM notification service. Note, the original image is located here.
In order to pair the mobile app with the desktop app, an encryption key and device token must be exchanged. The initial implementation will have the mobile app create the encryption key and transfer it together with the device token which it gets from Apple or Google to the Bisq desktop app.
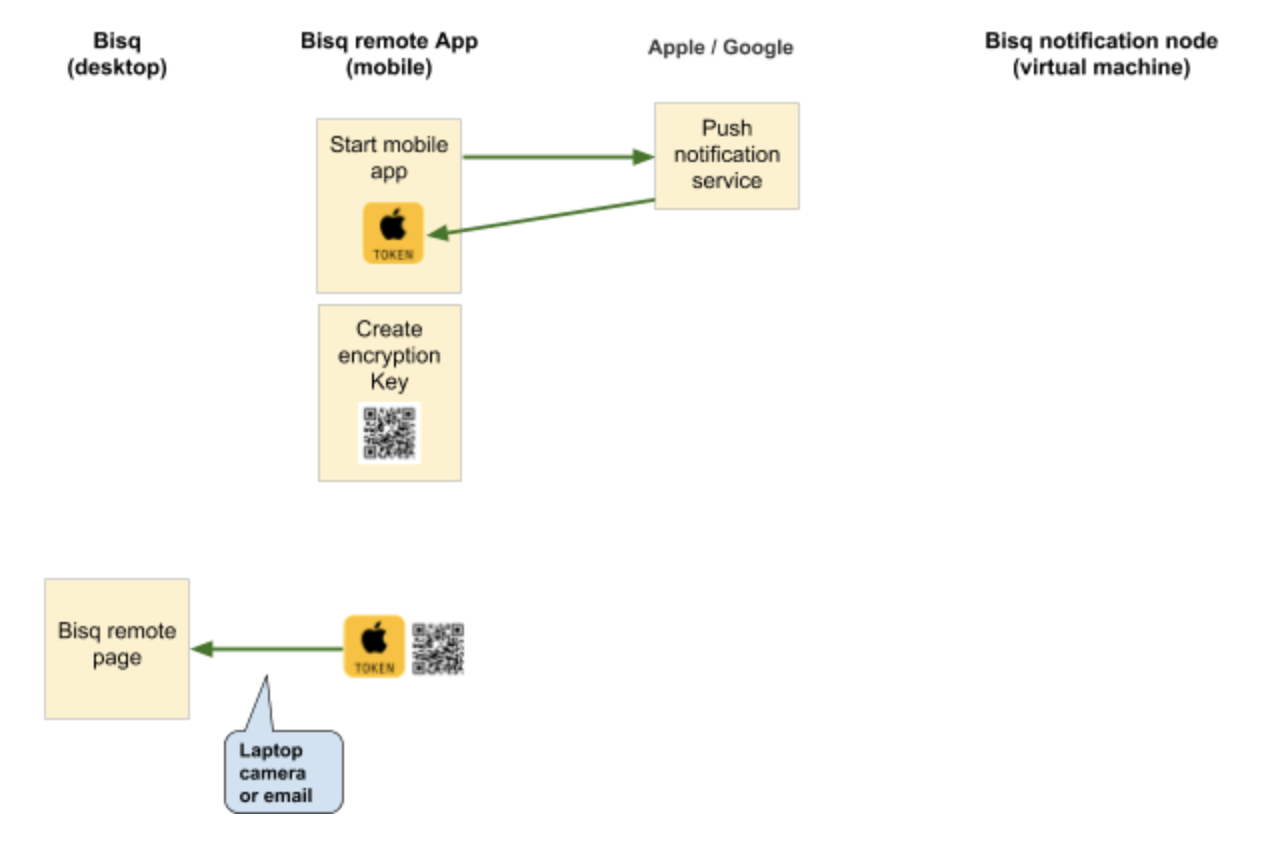
Figure 2: Pairing process during initial setup. Note, the original image is located here.
Steps:
- The mobile app registers with the Apple or Google notification service and receives a device token. The mobile app also creates an encryption key. This happens in the background without any user interaction.
- The app instructs the user to start the Bisq desktop app and navigate to the appropriate location in order to pair the app.
- The user pairs the app with the Bisq desktop app by transferring the device token and encryption key using one the following methods:
- Method 1: A QR code with the pairing token is created on the phone and scanned by the camera on the computer.
- Method 2: The user sends an email with the pairing token from his phone to himself. On the computer he copies the pairing token into the Bisq desktop app.
If the Pairing token is valid, a confirmation notification is automatically sent to the mobile app.
The Pairing token consists of four parts which are separated by the "|" character:
- A Magic, currently one of iOS, iOSDev or android
- A Phone descriptor, e.g., iPhone6
- A 32 byte cryptographic key for symmetric encryption. This key is generated by the phone and used in the Bisq desktop app to encrypt the content of the notification.
- A Notification token, either from Apple or Google.
Example:
BisqPhoneAndroid|
iPhone6|
f89e5160b3634ee6b51995e06af3e33e|
cEcb7vlj_SE:APA91bFYGqp_wsNv1OLHE3AeqUySkdUiNeuPv5yF
mgGuOWqEMVycQrwPom8oq1iFNPbp7raLbPxC4cxW99yFmhH8fiey
M2kXCyQxAG_y73hBha-TgtwR9r3MpQ852fljTVjw6zlOLD8t6Ufh
Qkx81lvhyaSLe2Q
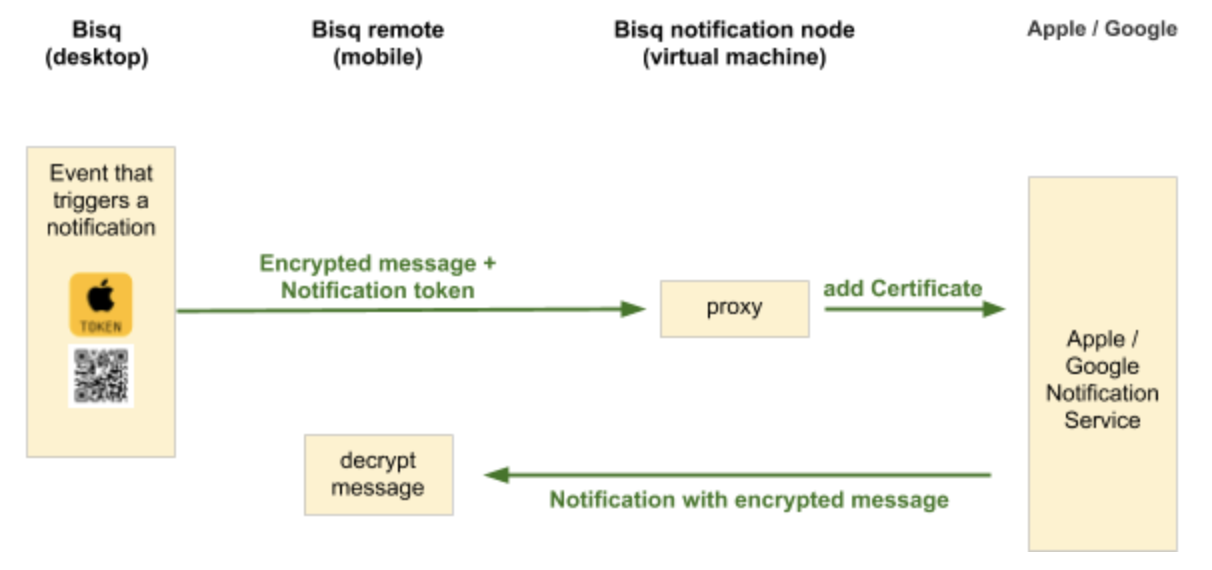
Figure 3: Notification flow - a notification is sent from the desktop app to the mobile app. Note, the original image is located here.
Steps:
- The Bisq desktop app sends a notification to the Bisq notification node via Tor. The notification consists of the device token and the encrypted message.
- The Bisq notification node forwards the encrypted message to the Apple or Google Push Notification Service using the device token via HTTP.
- The Apple/Google Push Notification service sends the notification to the iOS/Android device via HTTP.
- The phone notifies the user of a new Bisq message without displaying the content of the message.
- When the user opens the mobile app, it displays the decrypted notification message.
The Apple Push Notification Service has the advantage that the notifications reach the iOS device even if the app is not currently running. This convenience comes at the price that Apple knows when Bisq related notifications are sent to the user. However, since the notifications are encrypted, Apple does not know the content of the message.
The maximum notification payload size is 4 kilobytes (HTTP/2 API).
The pairing token contains a magic that allows for distinguishing between the production and development server:
iOS --> api.push.apple.com
iOSDev --> api.development.push.apple.com
Uses firebase.google.com.
The Bisq notification node acts as a proxy. This has the benefit that the Apple/Google certificate which is required to forward notifications to the Push Notification Service is not public. This makes it difficult to spam the Bisq notification node which would cause Apple or Google to block Bisq notifications.
The Bisq notification node is based on three components:
- A Server for the Apple or Google Push Notification service.
- A P2P network layer that allows to communicate to the Bisq nodes over Tor.
- An Apple or Google Certificate. Both are linked to a paid developer account.
We use the 128 bit AES/CBC/PKCS5Padding symmetric encryption algorithm. A fresh 16-character Initialization Vector is created for each notification. This Initialization Vector is be attached to the notification.
The notification payload contains an encrypted JSON string the describes the following object (in pseudo-code):
class BisqNotificationObject {
String timestampEvent
String transactionID
String title
String message
String notificationType
String actionRequired
int version
}
Specifically, the payload has three parts:
- A magic (BisqMessageiOS or BisqMessageAndroid)
- The 16-character Initialization Vector used in the encryption
- The encrypted message
Example:
BisqMessageiOS|
906aabd2d32f40d0|
0msuhNaWOSpIxL4/64kS8qjTc+tqmZ1wH9HLtfAUc4xNOZRVFAPX
JQ9gNoSfyZHN4oGbaefnjj6ym730d8hxkKXQB9F9vfmbtZk91Qr8
R+Qgu4AwzXMD39APacIIUXbsLnej0B461DQLcFnJA7kIhitEZov9
91Mhjo4CEnuA6VZOfcj/VvcGy0+mzxpTlsh8gy73+uyciNXq26Ew
7IlLo5GFpbTU3ku1yxmhM4SJNvEbJ0qt3L/smCNv3GgihNUz
The Bisq desktop allows to configure if the notifications sound an alarm when a notification arrived while the mobile app is in background.
The red badge on the app icon -- e.g., (1) -- is a bit complicated. The correct design is to send the badge number with the notification. However, this requires the Bisq notification server to count and manage the number of unread notifications. Instead, the mobile app sets the badge number. As a side-effect the badge number is not increased by iOS when the mobile app receives a notification while being in the background.
List of valid values for the field notificationType:
Type | example message | What happens in the phone |
---|---|---|
TRADE | Your offer has been accepted / trade completed, etc. | normal notification |
DISPUTE | Your bank transfer did not arrive | Warning notification |
FINANCIAL | An offer over x $ has been made | financial notification |
SETUP_CONFIRMATION | (no message) | setup terminated |
ERASE | (no message) | erases all settings and and all notifications |