⭐️ Star this project on GitHub: it motivates me a lot!
This project was born from the absence of libraries that work decently with Mongoose. It's a NestJS library that provides classes and methods for easy CRUD operations with Mongoose models.
With this project, you can accept complex queries, paginate results, and select fields, all fully compatible with OpenAPI and Swagger generation.
This project was developed using NodeJS v20.9.0, NPM v10.1.0 or Yarn v1.22.22 and NestJS v10.0.0.
Project dependencies:
- @nestjs/common: ^10.0.0
- @nestjs/config: ^3.2.0
- @nestjs/core: ^10.0.0
- @nestjs/mongoose: ^10.0.4
- @nestjs/platform-express: ^10.0.0
- @nestjs/swagger: ^7.3.0
- class-transformer: ^0.5.1
- class-validator: ^0.14.1
- mongoose: ^8.2.3
I do not guarantee backward compatibility with previous versions of NodeJS and NPM. Any requests are welcome.
BEFORE INSTALLING: please read the prerequisites
Depending on the package manager you prefer, you can install the library with npm or yarn.
$ npm install -S nestjs-mongoose-service
Or if you prefer to use Yarn:
yarn add nestjs-mongoose-service
Controller
// ...
import { RequestQueryDto } from 'nestjs-mongoose-service';
// ...
export class ExampleController {
constructor(private _serviceName: ServiceName) {
}
// ...
async createOne(@Body() body: ExampleDto, @Query(new ValidationPipe({ transform: true })) query: RequestQueryDto) {
return this._serviceName.createOne(body, query);
}
// ...
}
// example.controller.ts
In your entity file (optional)
if you want use entity remember to add @UseInterceptors(ClassSerializerInterceptor)
before the controller method.
// ...
export class ExampleEntity {
// ...
fieldName: string;
@Exclude()
fieldName2: string;
// ...
constructor(partial: Partial<ExampleEntity>) {
Object.assign(this, partial);
}
// ...
}
// example.entity.ts
// ...
import {
IRequestQueryCtx,
IRequestQueryResponse,
RequestQueryClass,
} from 'nestjs-mongoose-service';
// ...
export class ExampleService {
private _requestQueryClass: RequestQueryClass<ExampleDocument>;
constructor(
@InjectModel(Example.name)
private _exampleModel: Model<ExampleDocument>
) {
this._requestQueryClass = RequestQueryClass.create<ExampleDocument>(
this._exampleModel,
);
}
// ...
async createOne(
data: ExampleDto,
opts: IRequestQueryCtx<ExampleEntity> = {},
): Promise<IRequestQueryResponse<ExampleEntity>> {
try {
return await this._requestQueryClass.createOne<
ExampleDto,
ExampleEntity
>(user, opts);
} catch (error) {
throw new BadRequestException();
}
}
// ...
}
The documentation is under development and incomplete, I'm sure that by looking at the swagger and source files you will be able to understand how to use the library. If you need help, please open an issue. When I realize that the library will have a following, I will commit to completing the documentation.
This work is distributed under a License Creative
Commons Attribution - Share Alike 4.0 International.
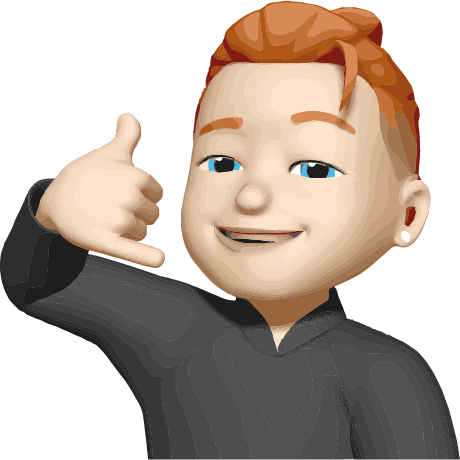