-
Notifications
You must be signed in to change notification settings - Fork 5
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
ac2bdf6
commit 8c60f3a
Showing
3 changed files
with
122 additions
and
3 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,5 +1,66 @@ | ||
# libnoise | ||
|
||
   [](https://coveralls.io/github/cookiephone/libnoise-rs?branch=master) | ||
 | ||
 | ||
 | ||
[](https://coveralls.io/github/cookiephone/libnoise-rs?branch=master) | ||
|
||
WIP - based on libnoise for C++ | ||
A simple, performant, and customizable procedural noise generation library | ||
inspired by [libnoise for C++](https://libnoise.sourceforge.net/). | ||
|
||
Libnoise provides utilities to generate coherent noise and customize them | ||
by applying a variety of operations which modify and combine generators. | ||
With a focus on customizability, the library allows users to create custom | ||
generators and modifiers. | ||
|
||
Most immediately relevant documentation can be found in `Source` and | ||
`Generator` docs. | ||
|
||
# Quickstart | ||
|
||
To get started easily, create a source generator using one of the many | ||
sources found in `Source`, and apply adapters documented in `Generator`. | ||
|
||
|
||
```rs | ||
use libnoise::prelude::*; | ||
|
||
// build a simplex noise generator seeded with 42 | ||
let generator = Source::simplex(42); | ||
|
||
// sample the generator for input point [0.2, 0.5] | ||
let value = generator.sample([0.2, 0.5]); | ||
``` | ||
|
||
Note how the dimensionality, which is internally represented as a constant | ||
generic argument, is automatically inferred by sampling the generator with | ||
a 2-dimensional input point. | ||
|
||
Naturally, we can create more interesting complex generators: | ||
|
||
```rs | ||
use libnoise::prelude::*; | ||
|
||
// build a generator | ||
let generator = Source::simplex(42) // start with simplex noise | ||
.fbm(5, 0.013, 2.0, 0.5) // apply fractal brownian motion | ||
.blend( // apply blending... | ||
Source::worley(43).scale([0.05, 0.05]), // ...with scaled worley noise | ||
Source::worley(44).scale([0.02, 0.02])) // ...controlled by other worley noise | ||
.lambda(|f| (f * 2.0).sin() * 0.3 + f * 0.7 ); // apply a closure to the noise | ||
|
||
// sample the generator for input point [0.2, 0.5] | ||
let value = generator.sample([0.2, 0.5]); | ||
``` | ||
|
||
We can also use `NoiseBuffer` for efficiently filling n-dimensional arrays | ||
with noise, and `Visualizer` to get a visual representation of a given | ||
generator. The above generator produces the following image, when sampled for | ||
every pixel position: | ||
|
||
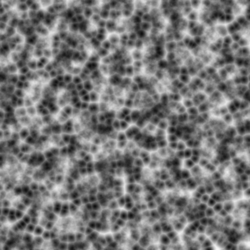 | ||
|
||
It is common to interpret the 3rd or 4th dimension as time, allowing us to | ||
produce space-time noise such as: | ||
|
||
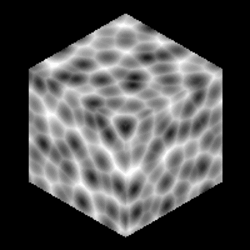 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters