-
Notifications
You must be signed in to change notification settings - Fork 19
How To
Table of contents:
-
HOW-TO Guide for Roadmapper
- How to use context manager 'with' syntax?
- How to display multiple tasks or activities on the same line?
- How to display milestone on tasks?
- How to change milestone text alignment?
- How to show generic dates instead of actual date?
- How to show current date marker 🔽?
- How to use the built-in colour scheme?
- How to create and use custom colour scheme?
- How to add sub title to the roadmap?
- How to let roadmapper auto adjust roadmap height?
- How to add logo to the roadmap?
- How to display non-English characters?
- How to change the timeline display format?
Refer to the following example:
with Roadmap(1200, 500, show_marker=False, auto_height=True, colour_theme="ORANGEPEEL") as my_roadmap:
my_roadmap.set_title("Context Manager Test Roadmap")
my_roadmap.set_timeline(TimelineMode.MONTHLY, start="2023-01-01")
# To create a group under the roadmap
with my_roadmap.add_group("Workstream 1") as group1:
# To create a task under the group
with group1.add_task("Task 1-A", "2023-01-01", "2023-04-30") as task1:
# To create a parallel task to the first task
with task1.add_parallel_task("Task 2-B", "2023-05-15", "2023-08-30") as parallel_task1:
# To add milestone to the parallel task
parallel_task1.add_milestone("Milestone 2", "2023-08-10")
# To add milestone to the task
task1.add_milestone("Milestone 1", "2023-04-01")
my_roadmap.draw()
my_roadmap.save("images/with_context_manager.png")
To display tasks on the same line as other tasks, call add_parallel_task()
method as shown below.
group = my_roadmap.add_group("Core Features")
task = group.add_task("Feature 1", "2022-12-01", "2023-02-10")
task.add_parallel_task("Feature 2", "2023-03-01", "2023-04-30")

Remember to use task
instance returned from add_task()
method to invoke add_parallel_task()
method. The code example basically shows "Feature 2" on the same parallel line as "Feature 1"
To display a milestone on task. call add_milestone() method.
task = group.add_task("Feature 4", "2023-01-21", "2023-05-30")
task.add_milestone("Milestone 1", "2023-05-31")

Remember to use 'task' instance returned from add_task() method to invoke add_milestone() method. The code example shows a milestone on 31 May 2023 with the label "Milestone 1"
To change the text alignment of milestones, use the text_alignment
parameter within the add_milestone()
method. This parameter can be a string in the format "direction" or "direction:offset", and utilizes the Alignment class to set the direction and offset for text alignment. Alignment directions are: left, right, center, or centre. Offset values can be a percentage of text width or units. If no offset value is given, the offset will be 1/2 (50%) of the text width. The type of units is determined by the Painter used during render time (png or svg for example.)
Here's an example of how to align milestone text:
group = roadmap.add_group("Milestone Alignment", text_alignment="left")
# Group containing each version of left-aligned milestone text
left_task = group.add_task(
"Alignment Example", start_date, "2023-12-31"
)
left_task.add_milestone(
"default left", "2023-05-01", text_alignment="left"
)
left_task.add_milestone(
"percent left", "2023-07-01", text_alignment="left:75%"
)
left_task.add_milestone(
"units left", "2023-11-01", text_alignment="left:10"
)
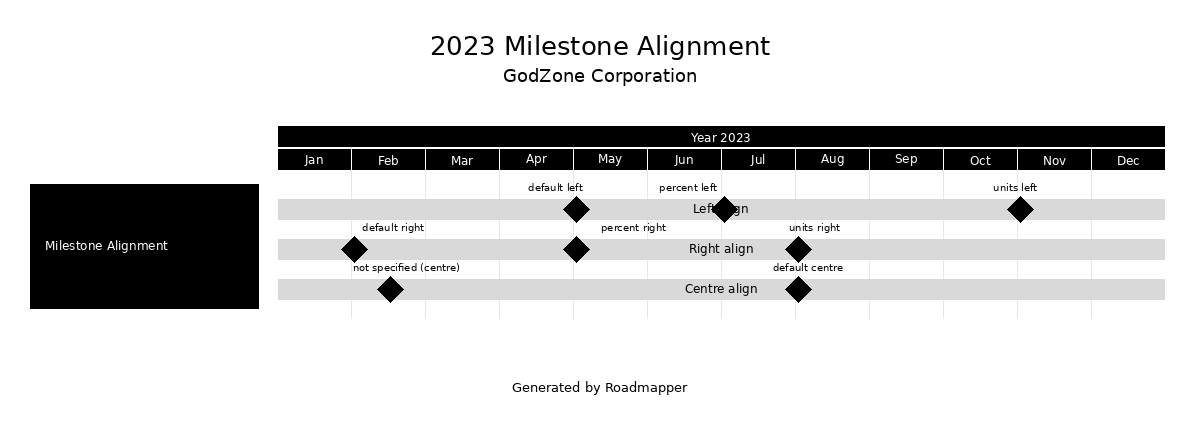
To show generic date such as 'Month 1, Month 2, etc", add show_generic_dates=True
to the set_timeline() method parameters.
my_roadmap.set_timeline(
mode=TimelineMode.MONTHLY,
start="2022-11-14",
number_of_items=6,
show_generic_dates=True
)
To display the current date marker, add show_marker=True
to the Roadmap() constructor parameters.
my_roadmap = Roadmap(4100, 2000, show_marker=True)
See the sample roadmap in the gallery
my_roadmap = Roadmap(4100, 2000, colour_theme="ORANGEPEEL")
There are five colour themes added into version 0.2.2:
- "DEFAULT"
- "BLUEMOUNTAIN"
- "GREENTURTLE"
- "ORANGEPEEL"
- "GREYWOOF"
See the sample roadmaps in the gallery
To create custom colour scheme, first create a .json file with the following content:
{
"theme": "RAINBOW",
"settings": {
"background": {
"background_fill_colour": "#FFFFFF"
},
"title": {
"title_font": "arial.ttf",
"title_font_size": 26,
"title_font_colour": "#000000",
"subtitle_font": "arial.ttf",
"subtitle_font_size": 18,
"subtitle_font_colour": "#000000"
},
"timeline": {
"timeline_year_font": "arial.ttf",
"timeline_year_font_size": 12,
"timeline_year_font_colour": "#FFFFFF",
"timeline_year_fill_colour": "#4C5B5C",
"timeline_item_font": "arial.ttf",
"timeline_item_font_size": 12,
"timeline_item_font_colour": "#FFFFFF",
"timeline_item_fill_colour": "#4C5B5C"
},
"marker": {
"marker_font": "arial.ttf",
"marker_font_size": 12,
"marker_font_colour": "#000000",
"marker_line_colour": "#000000"
},
"group": {
"group_font": "arial.ttf",
"group_font_size": 12,
"group_font_colour": "#FFFFFF",
"group_fill_colour": "#FCA311"
},
"task": {
"task_font": "arial.ttf",
"task_font_size": 12,
"task_font_colour": "#000000",
"task_fill_colour": "#8FBC94",
"task_style": "rectangle"
},
"milestone": {
"milestone_font": "arial.ttf",
"milestone_font_size": 12,
"milestone_font_colour": "#000000",
"milestone_fill_colour": "#3D6642"
},
"footer": {
"footer_font": "arial.ttf",
"footer_font_size": 12,
"footer_font_colour": "#000000"
}
}
}
- You can change font, font size, font colour, fill colour, and task style.
- Font colour and fill colour can be in hex format or in web colour name format. For example, "#FFFFFF" or "white".
Second, save the json file and give it a name. For example, "rainbow.json".
And finally, make a reference in your code as below:
my_roadmap = Roadmap(4100, 2000, colour_theme="rainbow.json")
Make sure your .py file and .json file are in the same folder.
To add sub title beneath the main title, just call the set_subtitle()
method.
my_roadmap.set_title("ROADMAP EXAMPLE 2022/2023")
my_roadmap.set_subtitle("This is a subtitle")
To let roadmapper to automatically resize the height, add auto_height=True
to the Roadmap constructor parameters.
my roadmap = Roadmap(
1200,
1400,
auto_height=True,
)
To add logo, call add_logo()
method and specify the display position.
my_roadmap.add_logo("matariki-tech-logo.png", position="top-right", width=50, height=50
-
position
can be one of the following values- top-left
- top-centre
- top-right
- bottom-left
- bottom-centre
- bottom-right
-
width
andheight
are used to resize the logo
To display non-English characters, follow the steps below:
- Download font file that supports unicode especially the one that support your language. For example, to display Traditional Chinese characters, you need to download a font that supports Traditional Chinese characters like this one HanSerifTC Font. Roadmapper uses Pillow library which support both TrueType and OpenType fonts.
- Place the font file in your designated folder relative to your .py file.
- Create a custom colour scheme .json file, change the font to the font file you downloaded in step 1. For example, if you downloaded the HanSerifTC font, you can change the font to "fonts/SourceHanSerifTC-Regular.otf". In my case, I store my font file in a folder called "fonts" relative to my .py file. The file is saved as
traditional-chinese.json
in the same folder as my .py file.
{
"theme": "TRADITIONAL-CHINESE",
"settings": {
"background": {
"background_fill_colour": "#FFFFFF"
},
"title": {
"title_font": "fonts/SourceHanSerifTC-Regular.otf",
"title_font_size": 26,
"title_font_colour": "#000000",
"subtitle_font": "fonts/SourceHanSerifTC-Regular.otf",
"subtitle_font_size": 18,
"subtitle_font_colour": "#000000"
},
"timeline": {
"timeline_year_font": "fonts/SourceHanSerifTC-Regular.otf",
"timeline_year_font_size": 12,
"timeline_year_font_colour": "#FFFFFF",
"timeline_year_fill_colour": "#4C5B5C",
"timeline_item_font": "fonts/SourceHanSerifTC-Regular.otf",
"timeline_item_font_size": 12,
"timeline_item_font_colour": "#FFFFFF",
"timeline_item_fill_colour": "#4C5B5C"
},
"marker": {
"marker_font": "fonts/SourceHanSerifTC-Regular.otf",
"marker_font_size": 12,
"marker_font_colour": "#000000",
"marker_line_colour": "#000000"
},
"group": {
"group_font": "fonts/SourceHanSerifTC-Regular.otf",
"group_font_size": 12,
"group_font_colour": "#FFFFFF",
"group_fill_colour": "#FCA311"
},
"task": {
"task_font": "fonts/SourceHanSerifTC-Regular.otf",
"task_font_size": 12,
"task_font_colour": "#000000",
"task_fill_colour": "#8FBC94",
"task_style": "rectangle"
},
"milestone": {
"milestone_font": "fonts/SourceHanSerifTC-Regular.otf",
"milestone_font_size": 12,
"milestone_font_colour": "#000000",
"milestone_fill_colour": "#4D8052"
},
"footer": {
"footer_font": "fonts/SourceHanSerifTC-Regular.otf",
"footer_font_size": 12,
"footer_font_colour": "#000000"
}
}
}
- Specify the custom colour scheme in the Roadmap constructor.
roadmap = Roadmap(800, 700, colour_theme="traditional-chinese.json")
If you are not happy on text display on the timeline particularly when you have a non-english roadmap. To change timeline text display format, follow the steps below:
- Create a timeline locale .json file like this one below.
You can change or customise the timeline text that you want to display. For example, if you want to display the year in Chinese, you can change the
year
text to{0} 年
. Just remember to keep the{0}
as it is. The following file is saved aszh_TW_timeline_settings.json
in the same folder as my .py file.
{
"locale": "zh_TW",
"settings": {
"year": {
"text": "{0} 年",
"generic_text": "第{0}年"
},
"half_year": {"text": "第{0}個半年"},
"quarter": {"text": "第{0}季度"},
"month": {
"text": "{0}",
"generic_text": "{0}月"
},
"week": {
"text": "{1}{0}",
"generic_text": "第{0}周"
}
}
}
- Please make sure the "locale" value you specified is supported by your machine. To get a list of support locale in your machine, run the following code:
import locale
locale.locale_alias
- Specify the timeline locale file in the
set_timeline()
method.
roadmap.set_timeline(
TimelineMode.QUARTERLY, "2023-01-01", 4, timeline_locale="zh_TW_timeline_settings.json"
)