-
Notifications
You must be signed in to change notification settings - Fork 39
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Empty relationships returned as data: null. #41
Comments
@wesselvdv, sorry for having breaking something... I agree with you that To clarify all cases: 1. If relationship is present and is null it should output with const JSONAPISerializer = require('json-api-serializer');
const Serializer = new JSONAPISerializer();
// Schools type
Serializer.register('schools', {
relationships: {
students: {
type: 'students',
links: {
self: '/students'
}
}
}
});
// Students type
Serializer.register('students', {
relationships: {
grades: {
type: 'grades',
links: {
self: '/grades'
}
}
}
});
Serializer.register('grades');
// Input data for schools
const input = {
"id": "1",
"name": "School Name",
"students": {
id: 1,
},
"grades": null
};
const output = Serializer.serialize('schools', input);
console.log(JSON.stringify(output)); Output should have {
"jsonapi": {
"version": "1.0"
},
"data": {
"type": "schools",
"id": "1",
"attributes": {
"name": "School Name"
},
"relationships": {
"students": {
"links": {
"self": "/students"
},
"data": {
"type": "students",
"id": "1"
}
}
}
},
"included": [
{
"type": "students",
"id": "1",
"attributes": {},
"relationships": {
"grades": {
"links": {
"self": "/grades"
},
"data": null
}
}
}
]
} 2. Considering I revert to const JSONAPISerializer = require('json-api-serializer');
const Serializer = new JSONAPISerializer();
// Schools type
Serializer.register('schools', {
relationships: {
students: {
type: 'students',
links: {
self: '/students'
}
}
}
});
// Students type
Serializer.register('students', {
relationships: {
grades: {
type: 'grades',
links: {
self: '/grades'
}
}
}
});
Serializer.register('grades');
// Input data for schools
const input = {
"id": "1",
"name": "School Name",
"students": {
id: 1,
}
};
const output = Serializer.serialize('schools', input);
console.log(JSON.stringify(output)); Output should not have data for grades relationship: {
"jsonapi": {
"version": "1.0"
},
"data": {
"type": "schools",
"id": "1",
"attributes": {
"name": "School Name"
},
"relationships": {
"students": {
"links": {
"self": "/students"
},
"data": {
"type": "students",
"id": "1"
}
}
}
},
"included": [
{
"type": "students",
"id": "1",
"attributes": {},
"relationships": {
"grades": {
"links": {
"self": "/grades"
}
}
}
}
]
} 3. Considering I revert to const JSONAPISerializer = require('json-api-serializer');
const Serializer = new JSONAPISerializer();
// Schools type
Serializer.register('schools', {
relationships: {
students: {
type: 'students',
links: {
self: '/students'
}
}
}
});
// Students type
Serializer.register('students', {
relationships: {
grades: {
type: 'grades'
}
}
});
Serializer.register('grades');
// Input data for schools
const input = {
"id": "1",
"name": "School Name",
"students": {
id: 1,
}
}; Output will have empty object for grades relationship {
"jsonapi": {
"version": "1.0"
},
"data": {
"type": "schools",
"id": "1",
"attributes": {
"name": "School Name"
},
"relationships": {
"students": {
"links": {
"self": "/students"
},
"data": {
"type": "students",
"id": "1"
}
}
}
},
"included": [
{
"type": "students",
"id": "1",
"attributes": {},
"relationships": {
"grades": {}
}
}
]
} This case is not clear regarding the spec. I don't think
Is @Mattii any ideas ? |
@danivek I agree with the first two cases. Those appear to correspond to the json:api spec, and will make sure that the behaviour introduced by #38 is reverted.
I wouldn't introduce this behaviour, this will cause a lot of confusion if objects just magically start disappearing.
I wouldn't do this either, this will cause the same situation as we have now, if I understand you correctly. I suggest to introduce the following behaviour if a defined relationship is missing in an object that's serialised:
Unless I am missing something, the above should make sure that json-api-serializer always creates objects that are compliant. |
@danivek thanks for the quick fix!! |
@danivek I am seeing results that contradicts the second case in your previous comment. I registered a type with about 15 relationships. I fetched data without including any relationships, the resulting json includes all 15 relationships as
I would expect that if my data does not have any relationships defined, it should not attempt to serialize them even if they are registered with my type. |
@danivek, thoughts? |
@alechirsch, I think it breaks JSON:API specification https://jsonapi.org/format/#document-resource-object-linkage
What your input data looks like ? |
According to this, it should NOT include related resources that were not requested https://jsonapi.org/format/#fetching-includes
I think #35 might be related to this. In my case, I am requesting 1 relationship in the |
What your input data looks like ?
|
Here is an example of what I am talking about:
This produces:
If data has an empty object called
Also this statement mentions
|
I would expect the code to be like this, it still produces null or empty array relationships IF the relationships are defined as empty in the data object provided.
As opposed to this:
|
@alechirsch You're right, regarding the spec there's no reason for a missing relationship to be serialized as |
The 'solution' for issue #38 seems to have introduced a breaking change in json-api-serializer, and in my opinion is making sure that json-api-serializer does not follow the json:api spec. It also makes sure that the problem faced in #25 is now caused by json-api-serializer, and no longer by improper serialisation to JSON.
The issue:
output:
Explanation:
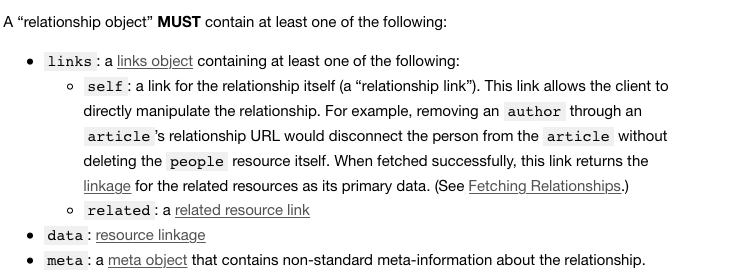
I know you think you're following the spec, but look at what it says:
It clearly states that ONE of those three has to be present. Not ALL three with a value of null. This literally broke my API.
My suggestion is to following the common convention regarding missing relationships. Keep data on undefined, so it'll be filtered out on stringify. Make sure that links are included for lazy loading, and if there's metadata present output that as well. If the relationship IS present, but has a value of null then it should be included with data is null.
I am downgrading to v1.9.1 where this change hasn't been included.
The text was updated successfully, but these errors were encountered: