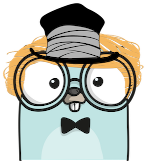
Edd WiSe - Event driven design over Web Socket
A tool to design uni or bi-directional event driven web applications.
You provide a simple description of your service within a yaml file, and you are able to generate documented code of both server (Golang) and client (Javascript). A dummy server/client implementations will be generated too, so you can fill them with business logic.
Speaking about events, it is natural to think how an event would influence the state of your application and of your remote clients.
Also, with the server code, the generation tool provides you with a "behave" interface that let you to naturally implement BDD scenarios thanks to a leveraging of goconvey around your defined events.
Download the latest version for your OS from releases or install it from go:
go install github.com/exelr/eddwise/cmd/edd
Define your design:
# design/pingpong.edd.yml
namespace: pingpong
structs:
ping:
id: uint
pong:
id: uint
channels:
pingpong:
client:
- ping
server:
- pong
View details
# design/pingpong.edd.yml
namespace: pingpong # the namespace of your generated code (packages for go)
structs:
ping: # ping is emitted from client
id: uint # the id of the ping
pong: # pong is sent from server after a ping
id: uint # the id of the pong, same as the id of the received ping
channels:
pingpong: # create a channel named pingpong
client: # define the events that can pass through the channel pingpong generated from client
- ping # set ping to be originated only from client
server: # define events generated from server
- pong # set pong to be originated only from server
# dual: # optional, you can define the events that are generated in both client and server
# - ping
# - pong
Generate the code:
edd design gen
Server:
// cmd/pingpong/main.go
package main
import (
"log"
"github.com/exelr/eddwise"
"pingpong/gen/pingpong"
)
type PingPongChannel struct {
pingpong.PingPong
}
func (ch *PingPongChannel) OnPing(ctx eddwise.Context, ping *pingpong.Ping) error {
return ch.SendPong(ctx.GetClient(), &pingpong.Pong{Id: ping.Id})
}
func main(){
var server = eddwise.NewServer()
var ch = &NewPingPongChannel{}
if err := server.Register(ch); err != nil {
log.Fatalln("unable to register service PingPong: ", err)
}
log.Fatalln(server.StartWS("/pingpong", 3000))
}
Client:
// web/pingpong/app.html
<script type="module">
import {EddClient} from '/pingpong/edd.js'
import {pingpongChannel} from '../../gen/pingpong/channel.js'
let client = new EddClient("ws://localhost:3000/pingpong")
let pingpong = new pingpongChannel()
client.register(pingpong)
pingpong.onpong((pong) => {
console.log("received pong with id", pong.id)
});
let pinginterval;
pingpong.connected(() => {
let id = 1;
pinginterval = setInterval(function () {
pingpong.sendping({id: id++})
}, 1000)
})
pinginterval.disconnected = function(){
clearInterval(pinginterval)
}
</script>
You can also generate skeleton code for client and server directly:
edd design skeleton
See Examples repo.
A full demo of a simple web game is available, see Filotto.
- GRPC over websocket
- AsyncAPI with websocket extension
- WAMP (for the ws not for the design)
- Flogo
Logo from gopherize.me