New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[camera] Provide a way to record a video in landscape when device orientation is locked to portrait #109381
Labels
c: new feature
Nothing broken; request for a new capability
c: proposal
A detailed proposal for a change to Flutter
p: camera
The camera plugin
P3
Issues that are less important to the Flutter project
package
flutter/packages repository. See also p: labels.
team-ecosystem
Owned by Ecosystem team
triaged-ecosystem
Triaged by Ecosystem team
Comments
Hello @tda-thaovd. I am not sure if this is a valid feature or something that should be implemented by another plugin and/or method channels. Anyways, I will label it for further insights from the team. Thank you for filing this request |
I did it after customize CameraPreview and listen rotation from my app. Hope this help some one try to do that.
In the widget which has customcamerapreview
|
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Labels
c: new feature
Nothing broken; request for a new capability
c: proposal
A detailed proposal for a change to Flutter
p: camera
The camera plugin
P3
Issues that are less important to the Flutter project
package
flutter/packages repository. See also p: labels.
team-ecosystem
Owned by Ecosystem team
triaged-ecosystem
Triaged by Ecosystem team
Use case
i want to have more use case rotation in camera flutter.
Proposal
For example: Initially, device is in portrait mode . Then i rotate screen to horizontal (remember that the device rotation is set to locked) and record video. My expectation is the output video is in landscape mode but the actual result is that the video is still in portrait mode. For more detail you can see in the CameraX usecase rotation How to determine the target rotation example 1
Procedure:
(the status bar show that device is still in portrait mode)
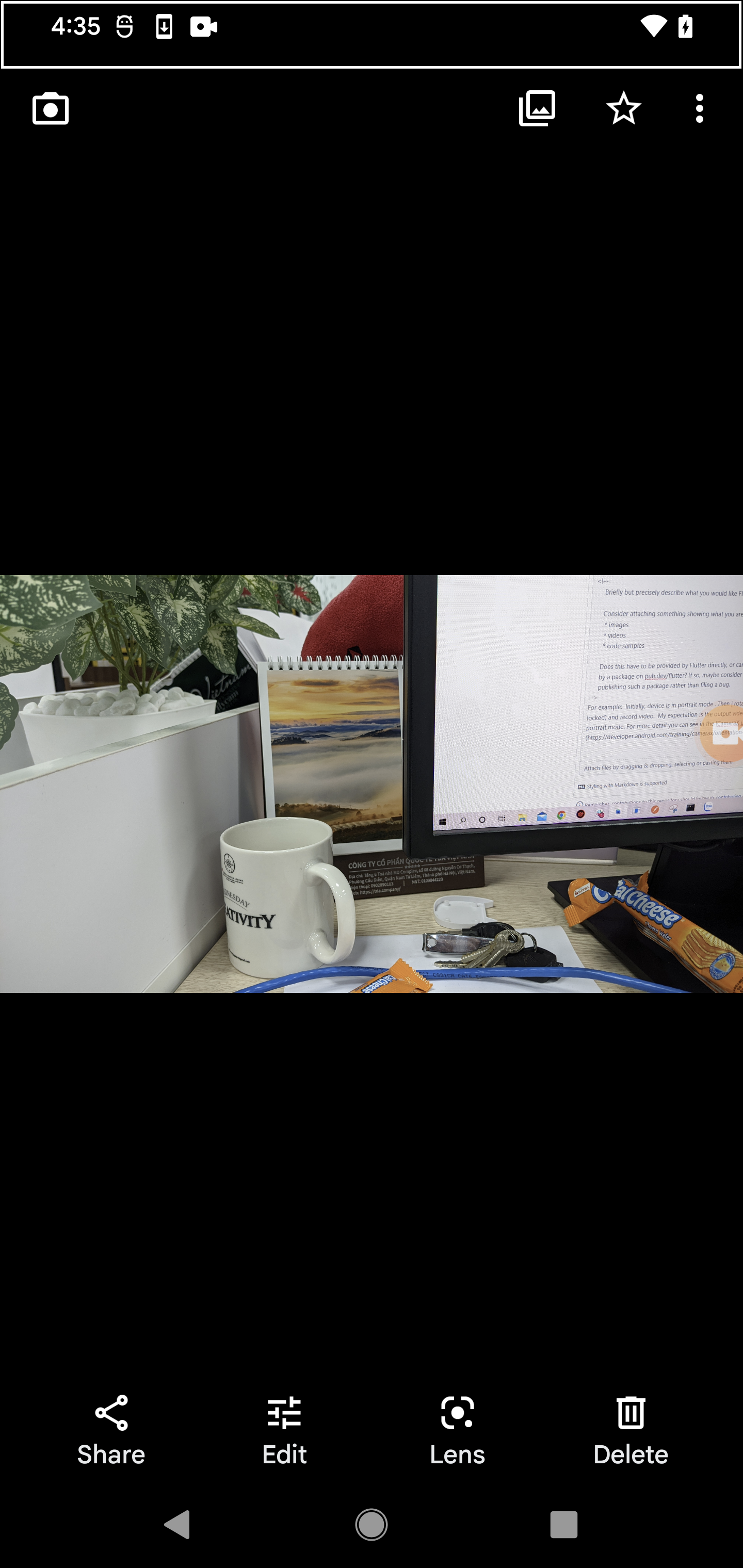
(The picture or video which is recorded should be in portrait mode).In android, they have some function like
Display.getRotation()
to get this device, we can use it to changerecordingRotation
before record video.The text was updated successfully, but these errors were encountered: