-
-
Notifications
You must be signed in to change notification settings - Fork 15
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Circular reference detection is too aggressive #11
Comments
Sorry, the error message wasn't good.
I feel this is the only way. e.g.
|
Sadly, this won't work because as you can See a bit down there, not all types use Operands, they can use different parameters |
You're right.
I think it has to be that way with a UI that uses different parameters. |
Maybe then add an option to disable circular reference detection? I will use code generation to automatically generate UI code, but I still need a way to avoid having circular reference error popping up in wrong places. Maybe switch type-based detection to instance-based detection? |
Yeah, now that I know about this case, I'll think about it. |
I was trying to make a simple boolean nodes calculator, but quickly ran into "Circular reference detected"
Code
This is the result I get in the UI
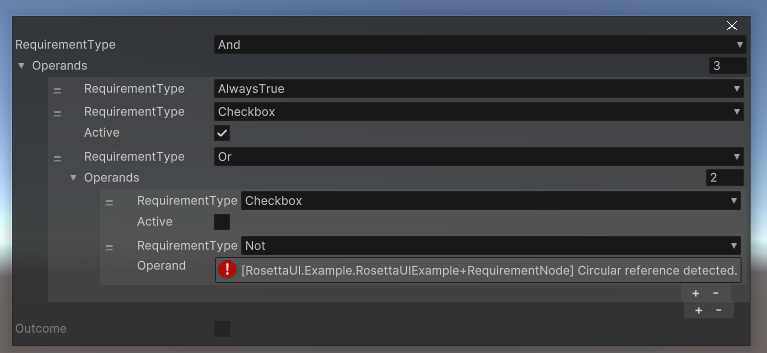
As you can see, all nodes are working normally, except for
Not
node that throws circular reference, even tho I clearly don't have any in my code.Also, I have an unrelated question: Is there a better way to make an editor for this kind of data structure? Aka one "holder" that have a
type
field, and abody
field that contains different classes depending ontype
. My current implementation requires to manually write UI controls for every possiblybody
class, and I'm concerned about performance implication of dozens ofDynamicElement
s performing checks ontype
field every tickThe text was updated successfully, but these errors were encountered: