-
Notifications
You must be signed in to change notification settings - Fork 605
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
How does decode
method on Reader
works?
#1610
Comments
The |
Note that this line |
Guys I'm very thankful you for your responses! I have been stuck with this for a week now. It's great that you let newbies like me ask questions about your library in issues! @fintelia Thank you very much it helped me a lot! I'm pretty new to image processing too, so I'm wondering why @HeroicKatora Thank you, I didn't know about Can you give me a hint on where else I can ask questions and discuss with people image processing as whole and specifically in Rust and JS? I found that it's hard to find almost any information about this topic, I'm wondering it there any group or chat where people discuss such things? |
Yes, exactly.
That actually wouldn't help much. We return an array of |
@fintelia Yea, that makes sense. Thank you for that answer! But then can you, please, explain me why initial array (under xxx1 in logs) has different values and quantity of them in contrast to array that I get from |
The first array is a JPEG file represented as an array of bytes. Image files like JPEG consist of raw pixel data combined with metadata (things like the width and height) so that they can be easy exchanged over the internet or stored on disk. They usually also have some approach for compressing the pixel data so it takes up less space. In the case of JPEG, the technique used is quite complicated but luckily you can just use an implementation some else wrote. The problem with image formats is that you generally cannot quickly extract individual pixel values from them. The same compression algorithms that shrink the file size also drastically increase the complexity of figuring out individual pixel values. Thus, the typical approach is to immediately |
@fintelia Thank for your explanation! Am I right in thinking that to encode JPEG image I need to use |
Yep. Either that method, or a higher level one like save_buffer_with_format (which just calls into the encoder internally) |
Thank you! I'm closing this issue, you helped me a lot! |
Hello!
I'm pretty new to Rust, can someone please help me to understand how does
decode
method onReader
works?Overall, I'm trying to to pass image from JS to Rust, process it and return back from Rust to JS.
My JS code look like this:
And Rust
Image.new
andImage.get_image_blob
functions looks like this:When I'm trying to load and return jpeg image in browser console I get this:
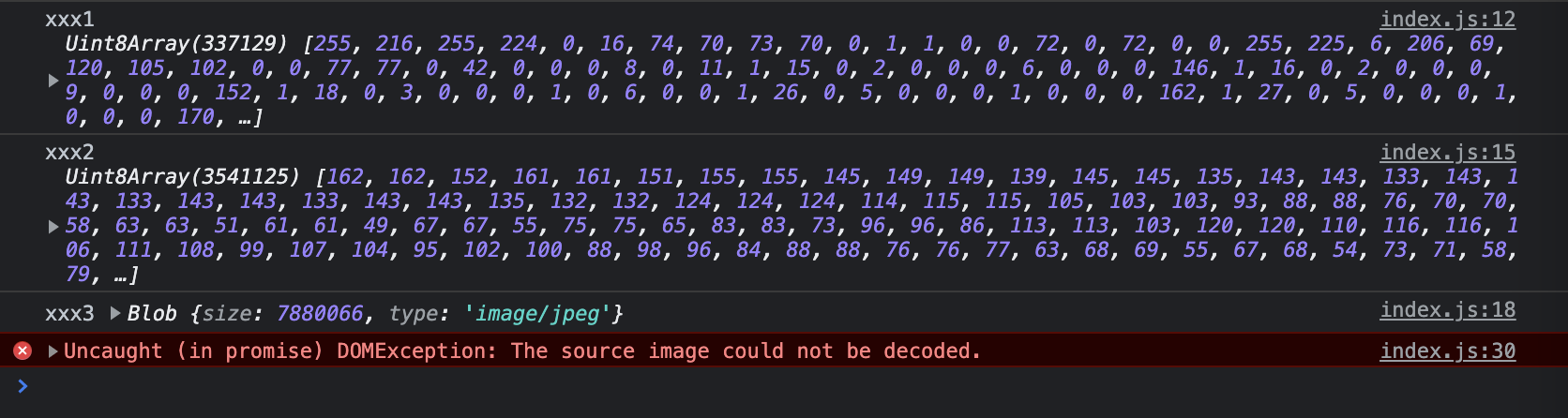
I was trying to find source of the problem and It's look like
decode
somehow changes blob content and I don't know why. Before I process my image with Rust it has right file signature for jpeg: [255, 216, 255, ...] and was 1,5 MB file. But after processing it became [162, 162, 152, ...] and became 15MB file. Can you help me and explain what I'm doing wrong?Thank you!
The text was updated successfully, but these errors were encountered: