Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
* update * inheriteance-java * Added inheritance in java
- Loading branch information
1 parent
2ff56a2
commit a4414dc
Showing
7 changed files
with
231 additions
and
4 deletions.
There are no files selected for viewing
File renamed without changes.
File renamed without changes.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,96 @@ | ||
class Person | ||
{ | ||
private String name; | ||
private long phno; | ||
|
||
public void read() | ||
{ | ||
name = "Akash"; | ||
phno = 928374993; | ||
} | ||
|
||
public void show() | ||
{ | ||
System.out.println("Name = " + name); | ||
System.out.println("Phone = " + phno); | ||
} | ||
} | ||
|
||
class Student extends Person | ||
{ | ||
private int rollno; | ||
private String course; | ||
|
||
public void read() | ||
{ | ||
super.read(); | ||
rollno = 007; | ||
course = "Computer Science"; | ||
} | ||
|
||
public void show() | ||
{ | ||
super.show(); | ||
System.out.println("Roll No. = " + rollno); | ||
System.out.println("Course = " + course); | ||
} | ||
} | ||
|
||
class Teacher extends Person | ||
{ | ||
|
||
private String dept_name; | ||
private String qual; | ||
|
||
public void read() | ||
{ | ||
super.read(); | ||
dept_name = "CSE"; | ||
qual = "PhD"; | ||
} | ||
|
||
public void show() | ||
{ | ||
super.show(); | ||
System.out.println("Departement = " + dept_name); | ||
System.out.println("Qualififcation = " + qual); | ||
} | ||
} | ||
|
||
class Hierarchical | ||
{ | ||
|
||
public static void main(String args[]) | ||
{ | ||
Student s1 = new Student(); | ||
|
||
s1.read(); | ||
System.out.println("******************** Displaying Student Information ********************"); | ||
|
||
s1.show(); | ||
|
||
Teacher t1 = new Teacher(); | ||
|
||
t1.read(); | ||
System.out.println("******************** Displaying Teacher Information ********************"); | ||
|
||
t1.show(); | ||
} | ||
} | ||
|
||
|
||
/* OUTPUT | ||
******************** Displaying Student Information ******************** | ||
Name = Akash | ||
Phone = 928374993 | ||
Roll No. = 7 | ||
Course = Computer Science | ||
******************** Displaying Teacher Information ******************** | ||
Name = Akash | ||
Phone = 928374993 | ||
Departement = CSE | ||
Qualififcation = PhD | ||
*/ |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,68 @@ | ||
class Father | ||
{ | ||
int age; | ||
|
||
public void setage() | ||
{ | ||
age = 75; | ||
} | ||
|
||
public int getage() | ||
{ | ||
return age; | ||
} | ||
} | ||
|
||
class Son extends Father | ||
{ | ||
int S_age; | ||
|
||
public void setage_S() | ||
{ | ||
S_age = 45; | ||
} | ||
|
||
public int getage_S() | ||
{ | ||
return S_age; | ||
} | ||
} | ||
|
||
class GrandSon extends Son | ||
{ | ||
int GS_age; | ||
|
||
public void setage_GS() | ||
{ | ||
GS_age = 15; | ||
} | ||
|
||
public int getage_GS() | ||
{ | ||
return GS_age; | ||
} | ||
} | ||
|
||
class Multi_Level | ||
{ | ||
public static void main(String args[]) | ||
{ | ||
GrandSon obj = new GrandSon(); | ||
|
||
obj.setage(); | ||
obj.setage_S(); | ||
obj.setage_GS(); | ||
|
||
System.out.println("Father's age : " + obj.getage()); | ||
System.out.println("Son's age : " + obj.getage_S()); | ||
System.out.println("Grandson's age : " + obj.getage_GS()); | ||
} | ||
} | ||
|
||
/* Output | ||
Father's age : 75 | ||
Son's age : 45 | ||
Grandson's age : 15 | ||
*/ |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
# Inheritance | ||
|
||
Inheritance is where *derived class* inherits the members (variables and functions) of *base class*. | ||
|
||
The benefit of inheritance is that the derived class does not have to redeclare all the members which it inherits from the base class. Hence, reusability is achieved this makes code cleaner. | ||
|
||
## Types of Inheritance | ||
|
||
We have **three** types of Inheritance in Java. Namely, | ||
|
||
1. **Single Inheritance** | ||
|
||
In this type of inheritance one derived class inherits from only one base class. It is the most simplest form of Inheritance. | ||
|
||
 | ||
|
||
2. **Hierarchical Inheritance** | ||
|
||
In this type of inheritance, multiple derived classes inherits from a single base class. | ||
|
||
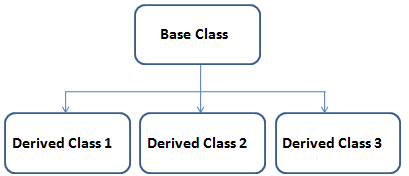 | ||
|
||
3. **Multilevel Inheritance** | ||
|
||
In this type of inheritance the derived class inherits from a class, which in turn inherits from some other class. The Super class for one, is sub class for the other. | ||
|
||
 | ||
|
||
### Note | ||
|
||
There is no concept of **Multiple Inheritance** and **Hybrid Inheritance** in Java. Derived class can have one and only one base class. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,35 @@ | ||
class Rectangle | ||
{ | ||
float length; | ||
float breadth; | ||
|
||
Rectangle() | ||
{ | ||
length = 5; | ||
breadth = 6; | ||
} | ||
} | ||
|
||
class Area extends Rectangle | ||
{ | ||
public float calculate() | ||
{ | ||
return length * breadth; | ||
} | ||
} | ||
|
||
class Single_Level | ||
{ | ||
public static void main(String args[]) | ||
{ | ||
Area a = new Area(); | ||
|
||
System.out.println("Area = " + a.calculate() + " square meters"); | ||
} | ||
} | ||
|
||
/* Output | ||
Area = 30 square meters | ||
*/ |