Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Kelly Norton
committed
Nov 28, 2021
1 parent
37648f8
commit d37948b
Showing
4 changed files
with
170 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,44 @@ | ||
# 129. Sum Root to Leaf Numbers | ||
|
||
https://leetcode.com/problems/sum-root-to-leaf-numbers/ | ||
|
||
You are given the root of a binary tree containing digits from 0 to 9 only. | ||
|
||
Each root-to-leaf path in the tree represents a number. | ||
|
||
- For example, the root-to-leaf path `1 -> 2 -> 3` represents the number `123`. | ||
|
||
Return the total sum of all root-to-leaf numbers. Test cases are generated so that the answer will fit in a 32-bit integer. | ||
|
||
A leaf node is a node with no children. | ||
|
||
**Example 1:** | ||
|
||
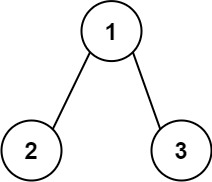 | ||
``` | ||
Input: root = [1,2,3] | ||
Output: 25 | ||
Explanation: | ||
The root-to-leaf path 1->2 represents the number 12. | ||
The root-to-leaf path 1->3 represents the number 13. | ||
Therefore, sum = 12 + 13 = 25. | ||
``` | ||
|
||
**Example 2:** | ||
|
||
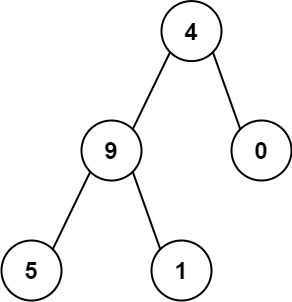 | ||
``` | ||
Input: root = [4,9,0,5,1] | ||
Output: 1026 | ||
Explanation: | ||
The root-to-leaf path 4->9->5 represents the number 495. | ||
The root-to-leaf path 4->9->1 represents the number 491. | ||
The root-to-leaf path 4->0 represents the number 40. | ||
Therefore, sum = 495 + 491 + 40 = 1026. | ||
``` | ||
|
||
**Constraints:** | ||
|
||
- The number of nodes in the tree is in the range `[1, 1000]`. | ||
- `0 <= Node.val <= 9` | ||
- The depth of the tree will not exceed `10`. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,38 @@ | ||
package p0129 | ||
|
||
/** | ||
* Definition for a binary tree node. | ||
* type TreeNode struct { | ||
* Val int | ||
* Left *TreeNode | ||
* Right *TreeNode | ||
* } | ||
*/ | ||
func sumNumbers(root *TreeNode) int { | ||
return sum(root, 0) | ||
} | ||
|
||
func sum(root *TreeNode, acc int) int { | ||
if root == nil { | ||
return 0 | ||
} | ||
|
||
r, l, v := root.Left, root.Right, root.Val | ||
|
||
if r == nil && l == nil { | ||
return acc + root.Val | ||
} | ||
|
||
res := (acc + v) * 10 | ||
var s int | ||
|
||
if l != nil { | ||
s += sum(l, res) | ||
} | ||
|
||
if r != nil { | ||
s += sum(r, res) | ||
} | ||
|
||
return s | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,24 @@ | ||
package p0129 | ||
|
||
import "testing" | ||
|
||
func TestSolution(t *testing.T) { | ||
tests := []struct { | ||
Input *TreeNode | ||
Expected int | ||
}{ | ||
{newTree(1, 2, 3), 25}, | ||
{newTree(4, 9, 0, 5, 1), 1026}, | ||
} | ||
|
||
for _, test := range tests { | ||
got := sumNumbers(test.Input) | ||
if got != test.Expected { | ||
t.Fatalf( | ||
"Input: %s, Expected: %d, Got: %d", | ||
test.Input.String(), | ||
test.Expected, | ||
got) | ||
} | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,64 @@ | ||
package p0129 | ||
|
||
import "fmt" | ||
|
||
type TreeNode struct { | ||
Val int | ||
Left, Right *TreeNode | ||
} | ||
|
||
func valueOf(v interface{}) *int { | ||
switch t := v.(type) { | ||
case int: | ||
return &t | ||
default: | ||
return nil | ||
} | ||
} | ||
|
||
func newTree(vals ...interface{}) *TreeNode { | ||
return newNode(vals, 0) | ||
} | ||
|
||
func newNode(vals []interface{}, ix int) *TreeNode { | ||
if ix >= len(vals) { | ||
return nil | ||
} | ||
|
||
v := valueOf(vals[ix]) | ||
if v == nil { | ||
return nil | ||
} | ||
|
||
return &TreeNode{ | ||
Val: *v, | ||
Left: newNode(vals, 2*ix+1), | ||
Right: newNode(vals, 2*ix+2), | ||
} | ||
} | ||
|
||
func (n *TreeNode) String() string { | ||
if n == nil { | ||
return "nil" | ||
} | ||
if n.Left == nil && n.Right == nil { | ||
return fmt.Sprintf("%d", n.Val) | ||
} | ||
|
||
return fmt.Sprintf( | ||
"(%d %s %s)", | ||
n.Val, n.Left.String(), | ||
n.Right.String()) | ||
} | ||
|
||
func nodesAreSame(a, b *TreeNode) bool { | ||
if a == nil { | ||
return b == nil | ||
} else if b == nil { | ||
return false | ||
} | ||
|
||
return a.Val == b.Val && | ||
nodesAreSame(a.Left, b.Left) && | ||
nodesAreSame(a.Right, b.Right) | ||
} |