-
Notifications
You must be signed in to change notification settings - Fork 2
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Add CSRF protection with remix-utils
- Loading branch information
1 parent
34d0194
commit 6f1254f
Showing
5 changed files
with
171 additions
and
52 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,48 +1,17 @@ | ||
<div align="center"> | ||
<h1 align="center"><a href="https://www.epicweb.dev/epic-stack">The Epic Stack 🚀</a></h1> | ||
<strong align="center"> | ||
Ditch analysis paralysis and start shipping Epic Web apps. | ||
</strong> | ||
<p> | ||
This is an opinionated project starter and reference that allows teams to | ||
ship their ideas to production faster and on a more stable foundation based | ||
on the experience of <a href="https://kentcdodds.com">Kent C. Dodds</a> and | ||
<a href="https://github.com/epicweb-dev/epic-stack/graphs/contributors">contributors</a>. | ||
</p> | ||
</div> | ||
|
||
```sh | ||
npx create-remix@latest --typescript --install --template epicweb-dev/epic-stack | ||
``` | ||
|
||
[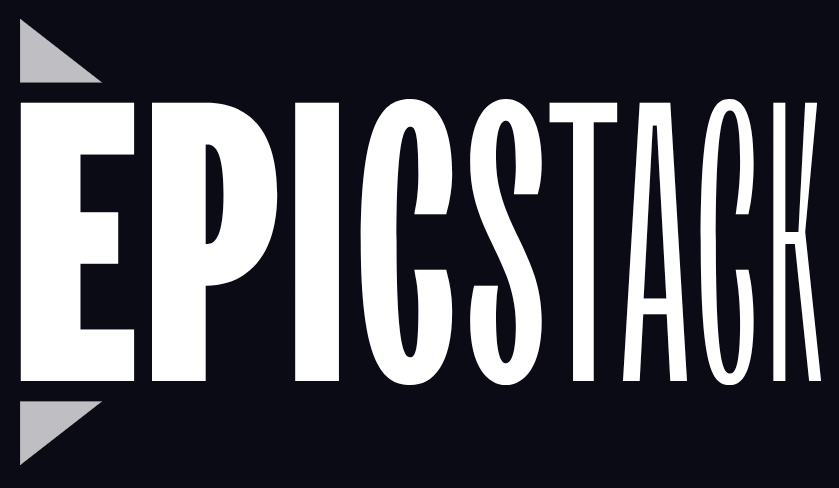](https://www.epicweb.dev/epic-stack) | ||
|
||
[The Epic Stack](https://www.epicweb.dev/epic-stack) | ||
|
||
<hr /> | ||
|
||
## Watch Kent's Introduction to The Epic Stack | ||
|
||
[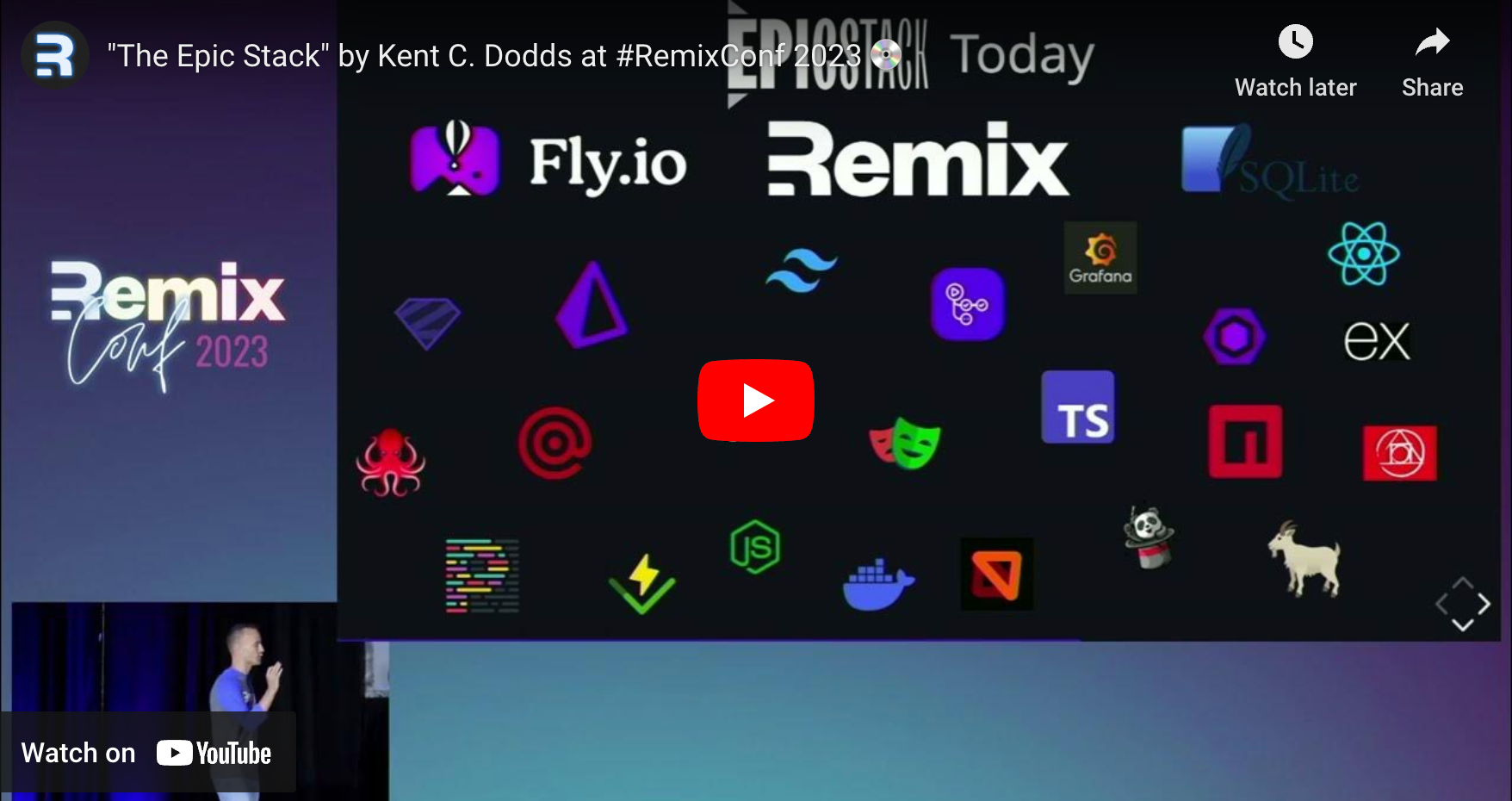](https://www.youtube.com/watch?v=yMK5SVRASxM) | ||
|
||
["The Epic Stack" by Kent C. Dodds at #RemixConf 2023 💿](https://www.youtube.com/watch?v=yMK5SVRASxM) | ||
|
||
## Docs | ||
|
||
[Read the docs](https://github.com/epicweb-dev/epic-stack/blob/main/docs) | ||
(please 🙏). | ||
|
||
## Support | ||
|
||
- 🆘 Join the | ||
[discussion on GitHub](https://github.com/epicweb-dev/epic-stack/discussions) | ||
and the [KCD Community on Discord](https://kcd.im/discord). | ||
- 💡 Create an | ||
[idea discussion](https://github.com/epicweb-dev/epic-stack/discussions/new?category=ideas) | ||
for suggestions. | ||
- 🐛 Open a [GitHub issue](https://github.com/epicweb-dev/epic-stack/issues) to | ||
report a bug. | ||
|
||
## Thanks | ||
|
||
You rock 🪨 | ||
# Epic Stack with CSRF Protection | ||
|
||
This is an example of how to integrate the | ||
[`remix-utils`](https://github.com/sergiodxa/remix-utils) package utilities for | ||
[Cross-Site Request Forgery (CSRF)](https://en.wikipedia.org/wiki/Cross-site_request_forgery) | ||
protection with the Epic Stack. The easiest way to explore the example is to | ||
pull up | ||
[the commit history](https://github.com/kentcdodds/epic-stack-with-csrf/commits/main). | ||
|
||
Following the steps laid out in the Remix Utils docs is sufficient for this: | ||
|
||
1. Install `remix-utils` | ||
2. Generate the authenticity token in the `root.tsx` loader (be certain to | ||
commit the session to set the cookie) | ||
3. Wrap the App in the `<AuthenticityTokenProvider />` and provide the token | ||
4. Render a Form with the `<AuthenticityTokenInput />` component | ||
5. Verify in the Action using `verifyAuthenticityToken` and the session. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Some generated files are not rendered by default. Learn more about how customized files appear on GitHub.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters