New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Katib Doesn't make Trials #926
Comments
Can you show us |
Here is my out I don't know What's Missing In my Eviroment, ttfob-example.yaml is Work!... What is Different? |
Sorry, my mistake. The command should be |
|
The experiment is created. Can you test
|
Is this not possible Parameter Type float? |
Yeah, we do not support float, we support double |
@gaocegege
|
Can you run
To see if it works? It seems that the command returns exit code 1 BTW, can you show us the logs of the pod? |
I think It has problem in Using Hangul...... |
@wjddyd66 Yeah I think so. Prefer English here. |
@gaocegege My Last Question is: Yout Eaxmplt .yaml File Here
And I Think Original Python Code Link is Here: https://github.com/kubeflow/tf-operator/blob/master/examples/v1/mnist_with_summaries/mnist_with_summaries.py What's mean accuracy_1???? |
It is the metric name in tfevent, I think. You can change it if there is another name in your TFEvent. |
@wjddyd66 It is the metric name which you can find when tf event file is parsed |
@johnugeorge Thank you I resolved that! It's very amazing |
/close |
@gaocegege: Closing this issue. In response to this:
Instructions for interacting with me using PR comments are available here. If you have questions or suggestions related to my behavior, please file an issue against the kubernetes/test-infra repository. |
/kind bug
Please check if I missed anything.
It's Work in Jupyter Notebook in Kubeflow
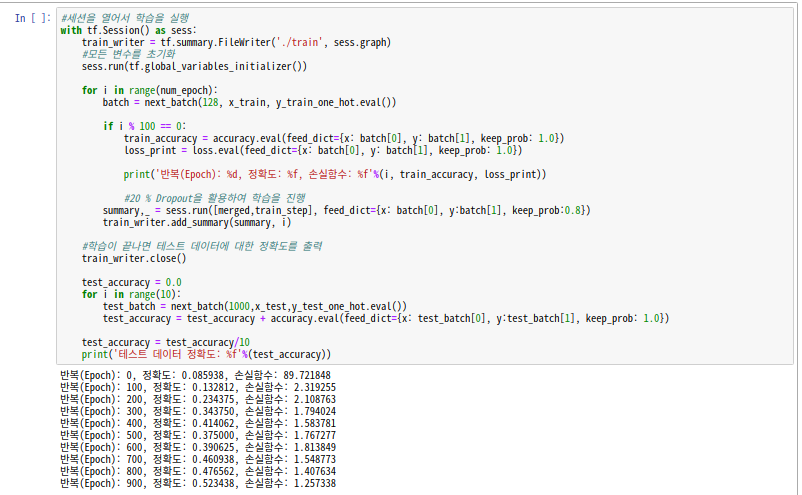
And It Makes tfevents File well in /train Folder
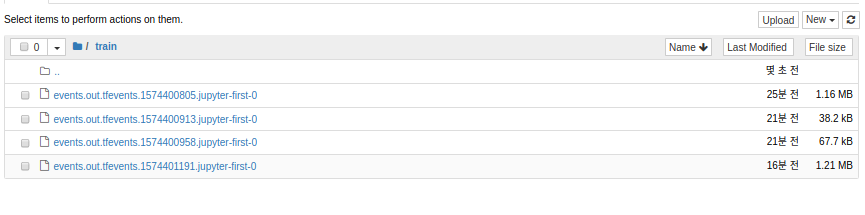
Dockerfile
But there is no Trials
I missed anything in this Step??? or Wrong .yaml file or python code?
The text was updated successfully, but these errors were encountered: