日本語の説明はここをクリックして展開
本プロジェクトは、旅行会社の予約管理システムのスタンドアロンアプリケーションであり、これはノースカロライナ州立大学交換留学時の 2019-Spring の CSC216(Programming Concept)のファイナルプロジェクトである。オブジェクト指向、エラーハンドリング、テスティング、デザインパターン(シングルトンパターン)、データ構造の理解を前提に、以下に取り組んだ。
- モデル層の実装(該当箇所はこちら)
- ArrayList, LinkedList の実装(該当箇所はこちら)
- これはデータ構造への理解を含めるという目的でスクラッチの実装を求められた
- 1 と 2 のユニットテスト(該当箇所はこちら)
このプロジェクトは github でコードを提出することを求められており、コード提出時に自動で Jenkins で CI テストが起動した。プロジェクトの完成条件は以下である。
- これは自らが書いた全てのメソッドをカバーし、尚且つコードカバレッジ 80%を満たすユニットテストが全て pass すること
- 1 が満たされた場合 TA によるユニットテストが起動する。これらが全て pass すること
- 実行可能ファイルを/TravelManager.jarからダウンロードする。
- ローカル環境で jar ファイルを起動する。
- 画面左上の File から、File>Load でデータをインポートする(初回時には File>New を選択)
- 使用後は左上の File から、File>Save でデータをアウトポートする。
このプロジェクトで実装されたクラスの Javadoc はこちらを参照してください。
プロジェクトの仕様は/CSC216P2P1.pdfで与えられた。想定されるユーザは旅行会社のスタッフであり、主要な仕様のいくつかは以下である。
- ユーザはホーム画面からは新しくツアーパッケージを登録できる
- 登録情報は、ツアー名/ツアータイプ/開始日時/日数/最大収容人数/価格である
- ツアータイプは LandTour, RiverCruise, EducationalTrip の3種類から選択する
- ユーザはホーム画面からクライアントを登録できる
- 登録情報は、氏名/連絡先である。
- ユーザはホーム画面から(1 で登録した)ツアーパッケージと(2 で登録した)クライアントを選択して、さらにそのクライアントのツアー参加人数を入力して、ツアーの予約ができる
- ユーザはホーム画面から(1 で登録した)パッケージの削除、(2 で登録した)クライアントの削除、(3 で登録した)予約のキャンセルができる
- ユーザはホーム画面のデータをローカルのテキストファイルにアウトポートすることで保存できる
- ユーザは現時点でのデータを保存/呼び出しすることができる。
- データの保存場所はローカルのテキストファイルである。
- 保存の際にはテキストファイルの名前を指定してデータを保存し、読み込みの際にはファイルの選択をしてデータを呼び出す。
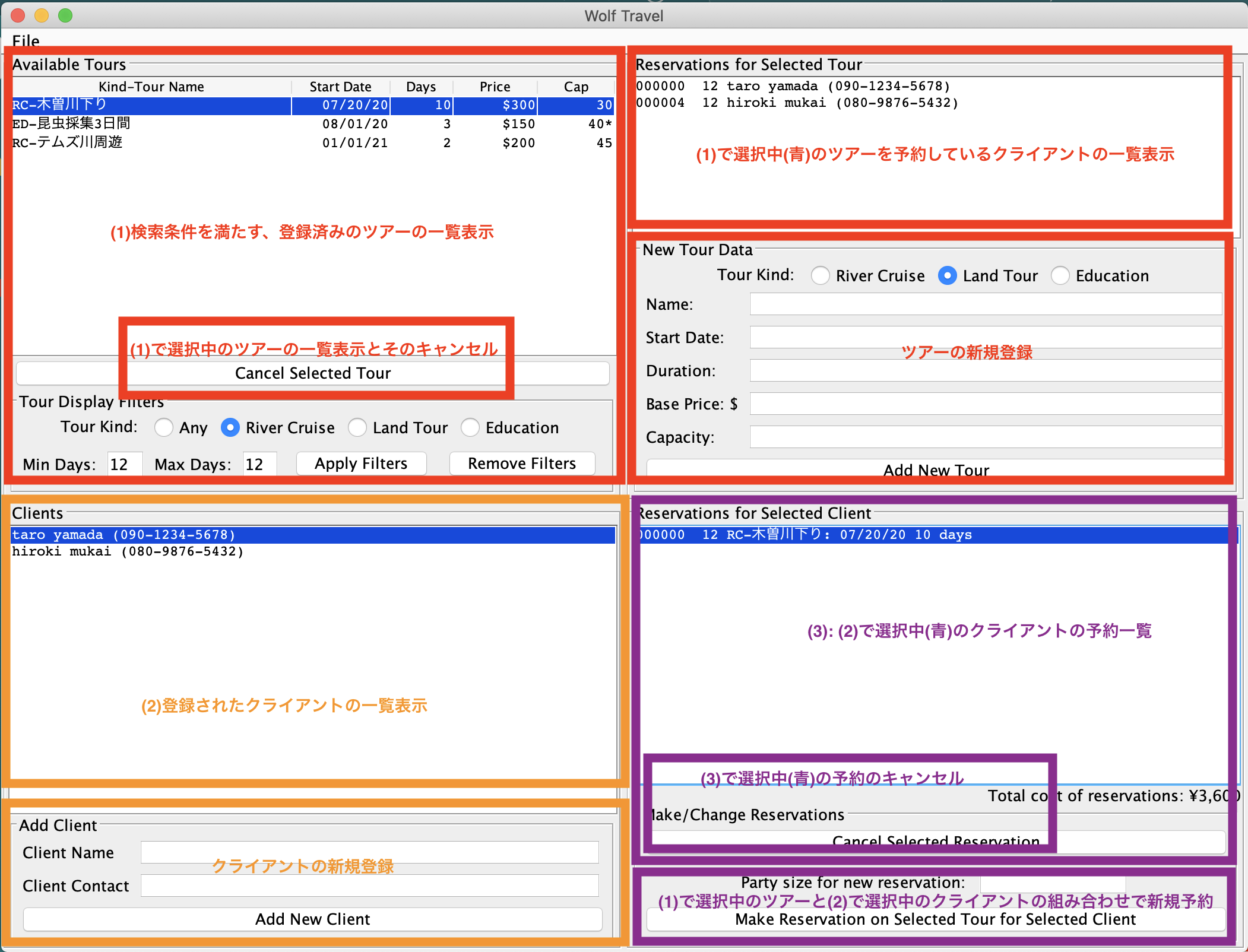
プロジェクトの設計およびその実装手順は/CSC216P2P2.pdfで与えられた。GUI ファイルに関しては既に完成したものが与えられており、Model 部分を以下のクラス図を元に eclipse で実装した(該当箇所はこちらである)。データの永続化はローカルのテキストファイルで行なっている。
以下特筆すべき点である。
- シングルトンパターンを採用している
- コンストラクターは private メソッドとして保持されており、TourCoordinator を外部から呼び出す際にはpublic static メソッドの getInstance()を使用する。
- この private メソッドのコンストラクターで初期化された TourCoordinator インスタンスはprivate static フィールドの instanceとして保持される。getInstance()メソッドを初めて呼び出す際には、この内部でコンストラクターが呼び出され、TourCoordinator インスタンスが初期化されて instance に収納される。二度目以降に getInstance()メソッドが呼び出された際には、instance を返す。
- この ArrayList はSimpleList インターフェイスを実装している。
- ArrayList は、初期化した状態では、内部的に長さ 12 の Array が使わている。要素を追加する中で Array のキャパシティーをオーバーするごとに 24→36→48→...と 12 ずつ長い Array に置き換えている(該当箇所はこちら)。
- この LinkedList はSortedList インターフェイスを実装している。この LinkedList は従来の LinkedList と比べると以下の二つの特徴を持つ。
- 複数の要素の重複を許さない
- 要素は、そのオブジェクトの compareTo メソッドを使用して、値が小さい順にソートされて収納される
- Cursorというネストされた private クラスを保持しており、これはSimpleListIterator インターフェイスを実装している。
- この Cursor はこの LinkedListIterator 内部のiterator()メソッド内で初期化されて返される。
- この Cursor はtoString()メソッドにおいて iterator()メソッドから呼び出された上で、この List を文字列で表現する際に使用される。
This is a Java standalone application for a staff of travel agencies to manage tour reservations of one's clients. I developed this system as a final project of 2019-Spring CSC216(Programming Concept) in North Carolina State University in my one year exchange program. In this project, I worked on implementation on the solid understandings of error handlings, testing, design patterns(e.g singleton pattern), data structure, etc.
- Implementation of model layers (See here)
- This includes I/O class of text files for saving data
- Implementation of ArrayList and LinkedList (See here)
- Full scratch implementation was required for deep understanding of these data structures.
- Unit test cases for 1 and 2 (See here)
Code was supposed to be pushed into GitHub, and CI unit testing were triggered automatically on Jenkins. To complete this project, I was expected to:
- Write test cases for all implemented methods, as well as the code coverage exceeding 80%.
- Pass all hidden test cases written by TA which is run in the CI process when 1 is met.
- Install executable file: /TravelManager.jar.
- Click and run the file in your local environment.
- Import saved data by clicking
File > Load
. (For the first time, clickFile > New
.) - When exiting, export saved data by clicking
File > Save
.
Javadoc of the classes implemented in this project is here.
Specifications of this project was given in /CSC216P2P1.pdf. Target users are staffs who are working for travel agencies. Some of the specifications are:
- User (= staff) can register new tour package from home page.
- Tour package has its tour name, tour type, start date, end date, capacity, and price.
- Tour type is one of LandTour, RiverCruise, EducationalTrip.
- User can register new client from home page.
- Client has one's name, phone number.
- User can create new reservation by selecting a tour package(1) and a client(2), as well as entering number of participants.
- User can delete tour packages(1), clients(2), reservations(3)
- User can import saved data from local text file and save data from text file.
- When importing from / exporting to a text file, user can specify the name of the file.
Design of this project and its implementation process was given in /CSC216P2P2.pdf. GUI files were given, and I was responsible for implementing classes of model layer. I used eclipse for implementation.
I will list some of the designs patterns where I learned a lot.
Singleton pattern is used.
- The constructor method is hold as a private method.
- When constructing TourCoordinator object, a public static method #getInstance is to be called. When you call #getInstance for the first time, its constructor method is called and the object is stored as its static field. When #getInstance is called after the second time, object stored as a field is returned.
This ArrayList implements SimpleList interface.
Internally, this ArrayList holds array whose length is 12. When number of elements exceeds the capacity, a whole new array which is 12 longer than original one is instantiated. (i.e. length would be 12→24→36→48,,,,). See here
This LinkedList implements SortedList interface. This class has these additional specification over conventional LinkedList.
- Duplication of elements are not allowed
- Elements are sorted in an ascending order. (Overridden #comparedTo method is used for deciding elements' ordering.)
- #toString method returns string representing its elements in the form of
[element_1.toString(), element_2.toString(),,, element_n.toString()]
For implementation of the third one, SortedLinkedListWithIterator class has an inner private class Cursor and a Cursor object is constructed in the method SortedLinkedListWithIterator#iterator. This cursor is constructed and used every time SortedLinkedListWithIterator#toString is called to traverse all the nodes.