New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Animation: camera setting lost while rotating multiple mesh objects #504
Comments
I will check, my first guess is that rotate() rotates around the origin (0,0,0) that's why everything goes off scale (you have LARGE scales!)
this is actually a bug, needs fixing..
see #505
this is probably the ffmpeg encoder.... you can still save screenshot series and then join them (i use a lot https://ezgif.com/) |
Thanks very much for the comment.
I guess I will just use the command-line ffmpeg. I was thinking of generating a series of screenshots but I decided to just use the Animation class but it did not work. I've already created a rotation video using just a single mesh object and recorded the screen while the movie is playing in the GUI. I think I can just use that one for the current task. I am happy to learn new tricks using vedo. Thanks very much for your help. |
Hi @marcomusy, I just updated to 2021.0.7 and I don't see the video is played inside the GUI anymore? |
..what GUI, you mean the rendering window? |
Yes. Previously I can watch it playing in that window but now nothing shows up! |
what is the output of |
vedo version : 2021.0.7 https://vedo.embl.es |
Are you sure it is still woking with vedo.2021.0.6 ? I noticed that on mac Monterrey it behaves differently.. from vedo import settings
settings.allowInteraction=True |
That solved the problem! |
That only solved the problem of showing things in the GUI but still the mp4 file cannot be viewed. |
I guess this is a problem of ffmpeg... cannot do much on that, you may use |
@marcomusy I am also having an issue animating multiple objects. For example I would like to show in parallel the two meshes from here #567 (comment) rotating but when I give:
I am getting the following:
Then I added the (ignore the yellow shade, it is an effect from the ffmpeg transformation of the video to .gif) Any idea? Also is the Also would be possible to have two sub-windows like in:
|
Hi @marcomusy, Ok, I've managed to have the different meshes in different sub-windows buy using the
but I have two questions, 1) do you know why I am getting these kind of flashing artifacts on some of the pieces and 2) how I can save this directly as a .gif or .mp4 file? I couldn't find something on the Plotter class. |
Hi
|
@marcomusy I am trying to save directly to According to this and this it is due to the transparency but passing the command:
doesn't really help much. Then I tried to set the
|
Uhm sorry I'm not an expert on this... if nothing works you can still save the separate png screenshots and merge them. |
Fair enough, no worries. Actually after a lot of trial and error I managed to have the following working and which also gives a good quality
Also I would suggest to comment/remove this line Line 1909 in 444b759
.mp4 extension to the output file.
Moreover, another thing I want to ask you is whether it is possible to individually and/or automatically adjust the camera zoom per window depending on the object's size. For example in the following output the first object is bigger that the other two so it appears closer to the camera (thus you also have the clipping) while the other two appear fine. The |
Looks great thanks for that.
Yes - removed
Either you share the same camera or have separate ones of each renderer. This is controlled by |
👍 possibly it could be connected also with the issue #210
👍
|
Hi @marcomusy,
I am playing with the Animation class and I found that my camera setting got lost while I try to rotate multiple mesh objects.
The code I used is something like this:
Here is the data required for this script to work:
final_mesh.vtk.zip
While I can try to just plot with one mesh object, it would still be interesting to make the above script work to add some more flexibility. I am not entirely sure whether I am doing something wrong or this is just the default behaviour. When you get a chance, could you please take a look at this?
Here is what things look like before they disappear (very last frame generated in the call to faceIn function):
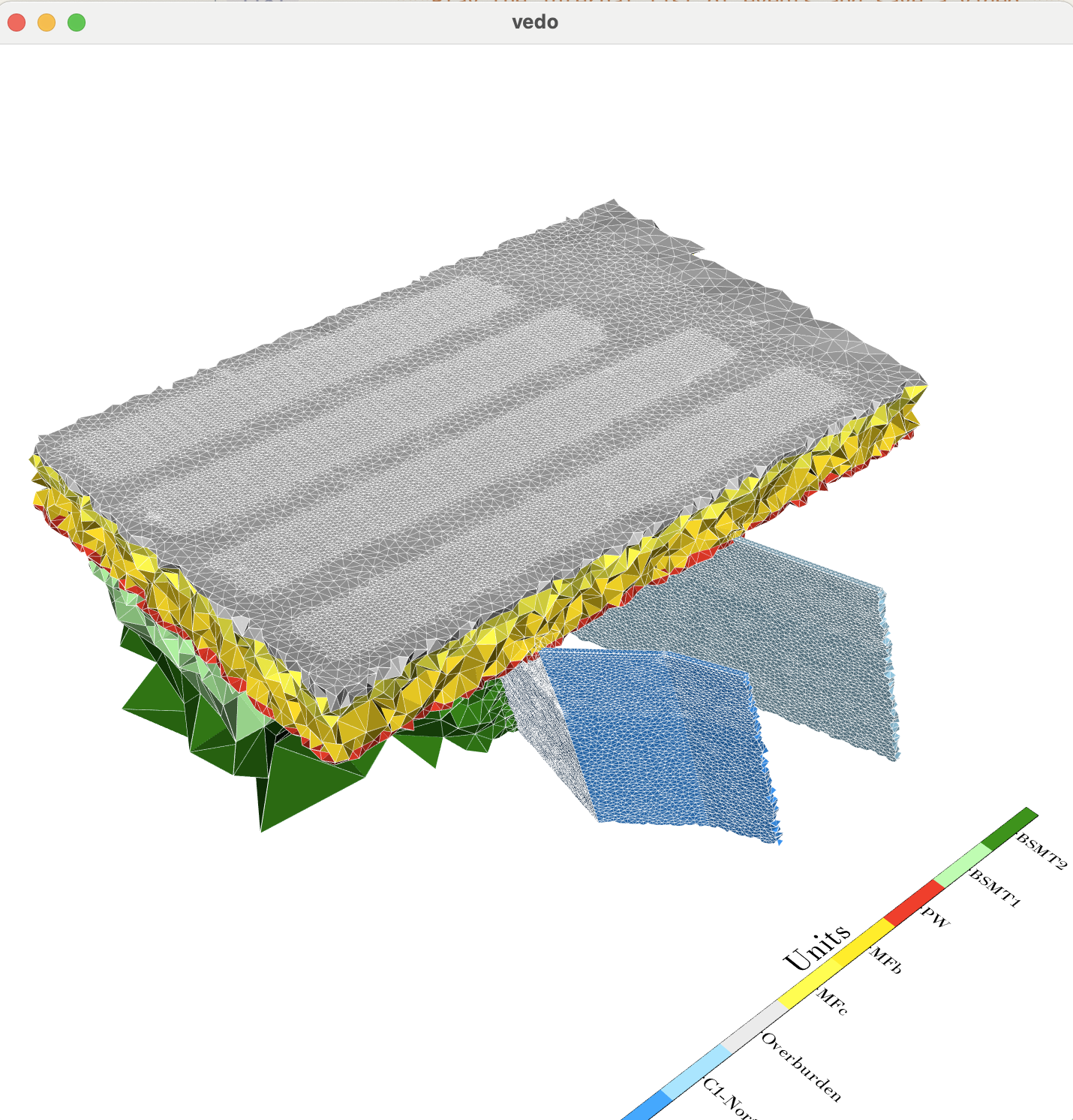
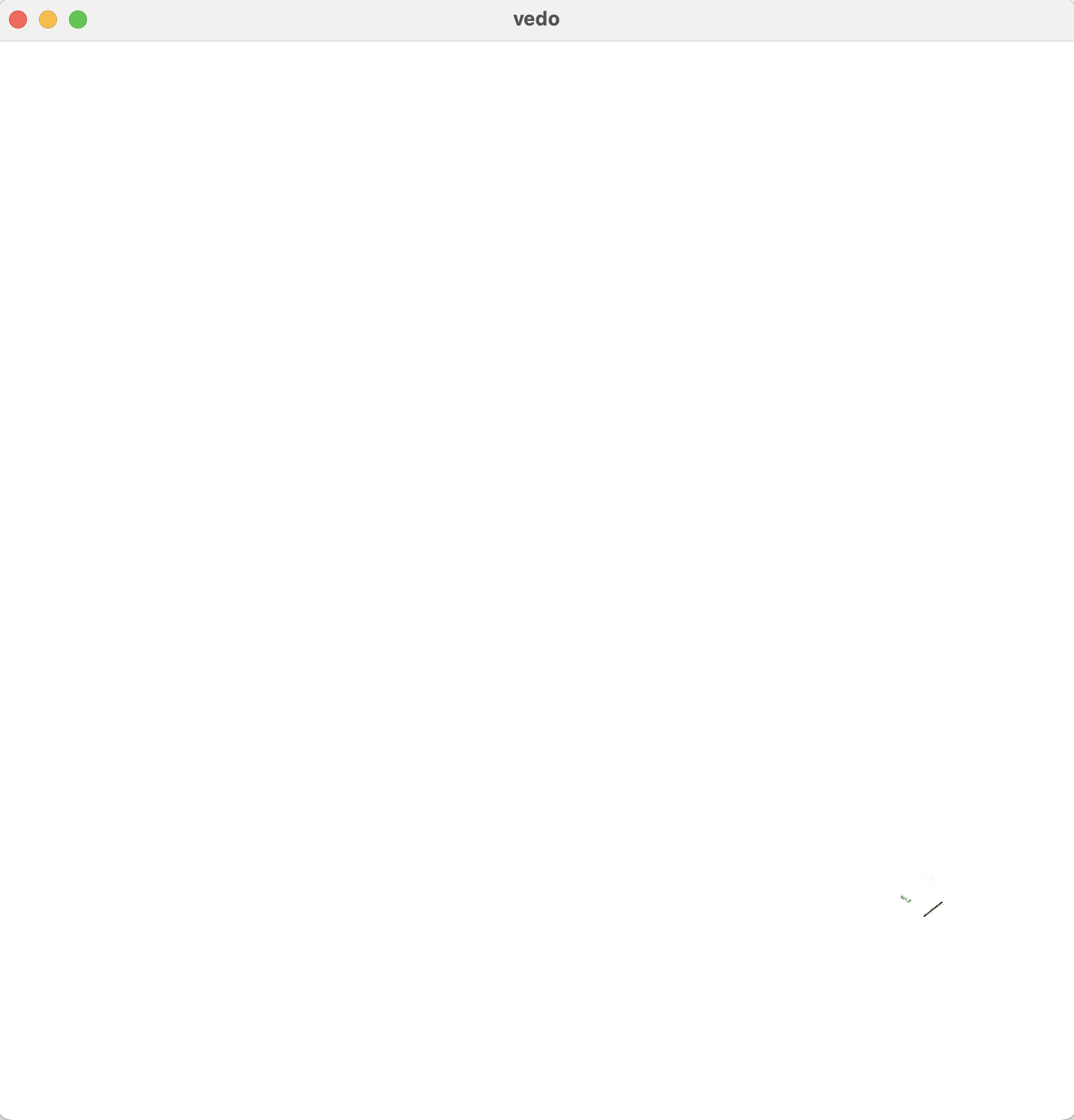
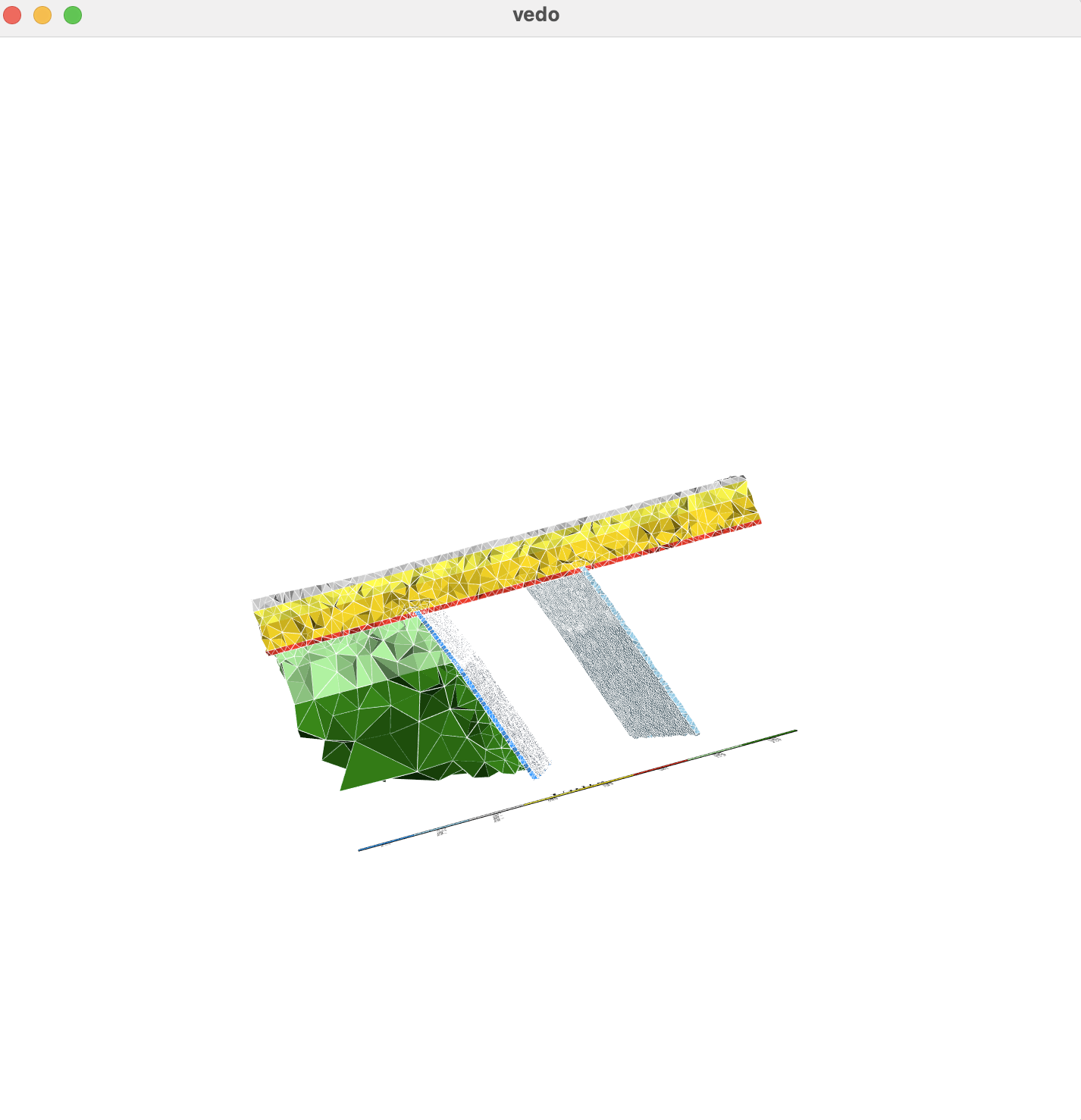
And here is what I mean by 'disappear' (can still be visible at the bottom-right coner):
You can see that they are still there if you zoom in to that area:
Well, I just discovered that it does not matter how many objects I rotate. This would happen even if I just rotate one object.
Also, there is no way for me to add Axes objects because of the following error: AttributeError: 'Assembly' object has no attribute 'alpha'".
The added 3D scalar bar also disappears.
The .mp4 file cannot be viewed using QuickTime Player on Mac. I've tried another video player and it does not work either.
Thanks very much,
Xushan
The text was updated successfully, but these errors were encountered: