-
Notifications
You must be signed in to change notification settings - Fork 2.8k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
GitHub OpenAPI skill integration with planner (#792)
### Motivation and Context This PR enables GitHub integration with Copilot Planner. ### Description Server-side - Added C# classes for GitHub schema objects - Adds tokenLimit for Planner response content. Currently, it's set at 75% of the remaining limit after memories response and document context have already been allocated. - Added `OptimizeOpenApiSkillJson` method that is called on Planner completion. This method: - removes all new line characters (huge token suck) from API response - truncates the API response by object until it's under the relatedInformationTokenLimit. - If the request can't be truncated, error message added to bot context - For GitHub skills specifically, the API response is deserialized into `PullRequest` or `PullRequest[]` objects to filter noisy properties. Web app side - If plugins require additional api requirements, these are added to the ask variables (rather than headers in http request) -- this allows the kernel/planner to directly consume these without any additional handling server side - `repo` and `owner` added as additional GitHub api requirements - Rename ApiRequirements to ApiProperties. These properties can be optional. 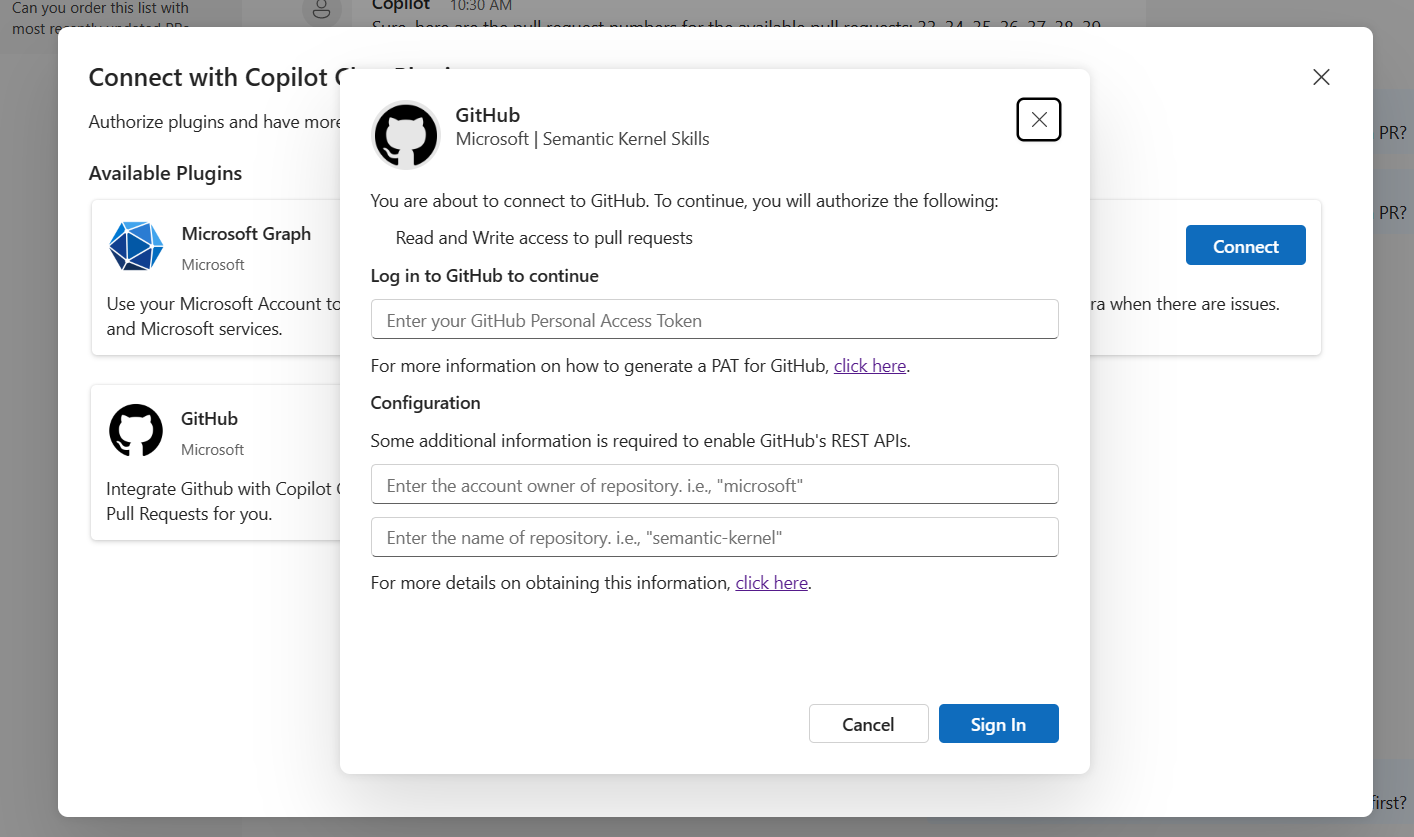
- Loading branch information
1 parent
bc399e4
commit 9917ca3
Showing
13 changed files
with
442 additions
and
89 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
40 changes: 40 additions & 0 deletions
40
samples/apps/copilot-chat-app/webapi/Skills/OpenApiSkills/GitHubSkill/Model/Label.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
using System.Text.Json.Serialization; | ||
|
||
namespace SemanticKernel.Service.Skills.OpenApiSkills; | ||
|
||
/// <summary> | ||
/// Represents a pull request label. | ||
/// </summary> | ||
public class Label | ||
{ | ||
/// <summary> | ||
/// Gets or sets the ID of the label. | ||
/// </summary> | ||
[JsonPropertyName("id")] | ||
public long Id { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the name of the label. | ||
/// </summary> | ||
[JsonPropertyName("name")] | ||
public string Name { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the description of the label. | ||
/// </summary> | ||
[JsonPropertyName("description")] | ||
public string Description { get; set; } | ||
|
||
/// <summary> | ||
/// Initializes a new instance of the <see cref="Label"/> class. | ||
/// </summary> | ||
/// <param name="id">The ID of the label.</param> | ||
/// <param name="name">The name of the label.</param> | ||
/// <param name="description">The description of the label.</param> | ||
public Label(long id, string name, string description) | ||
{ | ||
this.Id = id; | ||
this.Name = name; | ||
this.Description = description; | ||
} | ||
} |
116 changes: 116 additions & 0 deletions
116
samples/apps/copilot-chat-app/webapi/Skills/OpenApiSkills/GitHubSkill/Model/PullRequest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,116 @@ | ||
using System.Text.Json.Serialization; | ||
|
||
namespace SemanticKernel.Service.Skills.OpenApiSkills; | ||
|
||
/// <summary> | ||
/// Represents a GitHub Pull Request. | ||
/// </summary> | ||
public class PullRequest | ||
{ | ||
/// <summary> | ||
/// Gets or sets the URL of the pull request | ||
/// </summary> | ||
[JsonPropertyName("url")] | ||
public System.Uri Url { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the unique identifier of the pull request | ||
/// </summary> | ||
[JsonPropertyName("id")] | ||
public int Id { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the number of the pull request | ||
/// </summary> | ||
[JsonPropertyName("number")] | ||
public int Number { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the state of the pull request | ||
/// </summary> | ||
[JsonPropertyName("state")] | ||
public string State { get; set; } | ||
|
||
/// <summary> | ||
/// Whether the pull request is locked | ||
/// </summary> | ||
[JsonPropertyName("locked")] | ||
public bool Locked { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the title of the pull request | ||
/// </summary> | ||
[JsonPropertyName("title")] | ||
public string Title { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the user who created the pull request | ||
/// </summary> | ||
[JsonPropertyName("user")] | ||
public GitHubUser User { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the labels associated with the pull request | ||
/// </summary> | ||
[JsonPropertyName("labels")] | ||
public List<Label> Labels { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the date and time when the pull request was last updated | ||
/// </summary> | ||
[JsonPropertyName("updated_at")] | ||
public DateTime UpdatedAt { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the date and time when the pull request was closed | ||
/// </summary> | ||
[JsonPropertyName("closed_at")] | ||
public DateTime? ClosedAt { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the date and time when the pull request was merged | ||
/// </summary> | ||
[JsonPropertyName("merged_at")] | ||
public DateTime? MergedAt { get; set; } | ||
|
||
/// <summary> | ||
/// Initializes a new instance of the <see cref="PullRequest"/> class, representing a pull request on GitHub. | ||
/// </summary> | ||
/// <param name="url">The URL of the pull request.</param> | ||
/// <param name="id">The unique identifier of the pull request.</param> | ||
/// <param name="number">The number of the pull request within the repository.</param> | ||
/// <param name="state">The state of the pull request, such as "open", "closed", or "merged".</param> | ||
/// <param name="locked">A value indicating whether the pull request is locked for comments or changes.</param> | ||
/// <param name="title">The title of the pull request.</param> | ||
/// <param name="user">The user who created the pull request.</param> | ||
/// <param name="labels">A list of labels assigned to the pull request.</param> | ||
/// <param name="updatedAt">The date and time when the pull request was last updated.</param> | ||
/// <param name="closedAt">The date and time when the pull request was closed, or null if it is not closed.</param> | ||
/// <param name="mergedAt">The date and time when the pull request was merged, or null if it is not merged.</param> | ||
public PullRequest( | ||
System.Uri url, | ||
int id, | ||
int number, | ||
string state, | ||
bool locked, | ||
string title, | ||
GitHubUser user, | ||
List<Label> labels, | ||
DateTime updatedAt, | ||
DateTime? closedAt, | ||
DateTime? mergedAt | ||
) | ||
{ | ||
this.Url = url; | ||
this.Id = id; | ||
this.Number = number; | ||
this.State = state; | ||
this.Locked = locked; | ||
this.Title = title; | ||
this.User = user; | ||
this.Labels = labels; | ||
this.UpdatedAt = updatedAt; | ||
this.ClosedAt = closedAt; | ||
this.MergedAt = mergedAt; | ||
} | ||
} |
33 changes: 33 additions & 0 deletions
33
samples/apps/copilot-chat-app/webapi/Skills/OpenApiSkills/GitHubSkill/Model/Repo.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,33 @@ | ||
using System.Text.Json.Serialization; | ||
|
||
namespace SemanticKernel.Service.Skills.OpenApiSkills; | ||
|
||
/// <summary> | ||
/// Represents a GitHub Repo. | ||
/// </summary> | ||
public class Repo | ||
{ | ||
/// <summary> | ||
/// Gets or sets the name of the repo | ||
/// </summary> | ||
[JsonPropertyName("name")] | ||
public string Name { get; set; } | ||
|
||
/// <summary> | ||
/// Gets or sets the full name of the repo | ||
/// </summary> | ||
[JsonPropertyName("full_name")] | ||
public string FullName { get; set; } | ||
|
||
|
||
/// <summary> | ||
/// Initializes a new instance of the <see cref="Repo"/>. | ||
/// </summary> | ||
/// <param name="name">The name of the repository, e.g. "dotnet/runtime".</param> | ||
/// <param name="fullName">The full name of the repository, e.g. "Microsoft/dotnet/runtime".</param> | ||
public Repo(string name, string fullName) | ||
{ | ||
this.Name = name; | ||
this.FullName = fullName; | ||
} | ||
} |
56 changes: 56 additions & 0 deletions
56
samples/apps/copilot-chat-app/webapi/Skills/OpenApiSkills/GitHubSkill/Model/User.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,56 @@ | ||
using System.Text.Json.Serialization; | ||
|
||
namespace SemanticKernel.Service.Skills.OpenApiSkills; | ||
|
||
/// <summary> | ||
/// Represents a user on GitHub. | ||
/// </summary> | ||
public class GitHubUser | ||
{ | ||
/// <summary> | ||
/// The user's name. | ||
/// </summary> | ||
[JsonPropertyName("name")] | ||
public string Name { get; set; } | ||
|
||
/// <summary> | ||
/// The user's email address. | ||
/// </summary> | ||
[JsonPropertyName("email")] | ||
public string Email { get; set; } | ||
|
||
/// <summary> | ||
/// The user's numeric ID. | ||
/// </summary> | ||
[JsonPropertyName("id")] | ||
public int Id { get; set; } | ||
|
||
/// <summary> | ||
/// The user's type, e.g. User or Organization. | ||
/// </summary> | ||
[JsonPropertyName("type")] | ||
public string Type { get; set; } | ||
|
||
/// <summary> | ||
/// Whether the user is a site admin. | ||
/// </summary> | ||
[JsonPropertyName("site_admin")] | ||
public bool SiteAdmin { get; set; } | ||
|
||
/// <summary> | ||
/// Creates a new instance of the User class. | ||
/// </summary> | ||
/// <param name="name">The user's name.</param> | ||
/// <param name="email">The user's email address.</param> | ||
/// <param name="id">The user's numeric ID.</param> | ||
/// <param name="type">The user's type.</param> | ||
/// <param name="siteAdmin">Whether the user is a site admin.</param> | ||
public GitHubUser(string name, string email, int id, string type, bool siteAdmin) | ||
{ | ||
this.Name = name; | ||
this.Email = email; | ||
this.Id = id; | ||
this.Type = type; | ||
this.SiteAdmin = siteAdmin; | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.