New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[WIP][web] Add tests for components/ContentView (con't) #2376
Merged
Merged
Changes from all commits
Commits
Show all changes
11 commits
Select commit
Hold shift + click to select a range
46eae0a
[web] Add tests for js/components/ContentView/DownloadContentButton.jsx
MatthewShao 298ac83
[web] Add tests for js/components/ContentView/MetaViews.jsx
MatthewShao bff2327
[web] Add tests for js/components/ContentView/ShowFullContentButton.jsx
MatthewShao c9a7286
[web] Imporve indentation.
MatthewShao 3d51595
[web] Add tests for js/components/ContentView/UploadContentButton.jsx
MatthewShao 4d3d187
[web] Add tests for js/components/ContentView/ViewSelector.jsx
MatthewShao edd3632
[web] Add mock-xmlhttprequest dependency.
MatthewShao 55fd82b
[web] Add tests for js/components/ContentView/ContentLoader.jsx
MatthewShao 602ab1a
[web] Add tests for js/components/ContentView/ContentView.jsx
MatthewShao d1a40de
[web] Export PureViewServer in ContentView/ContentView.jsx
MatthewShao 49a04e3
[web] Update ContentLoader class name.
MatthewShao File filter
Filter by extension
Conversations
Failed to load comments.
Jump to
Jump to file
Failed to load files.
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
74 changes: 74 additions & 0 deletions
74
web/src/js/__tests__/components/ContentView/ContentLoaderSpec.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,74 @@ | ||
import React from 'react' | ||
import renderer from 'react-test-renderer' | ||
import withContentLoader from '../../../components/ContentView/ContentLoader' | ||
import { TFlow } from '../../ducks/tutils' | ||
import TestUtils from 'react-dom/test-utils' | ||
import mockXMLHttpRequest from 'mock-xmlhttprequest' | ||
|
||
global.XMLHttpRequest = mockXMLHttpRequest | ||
class tComponent extends React.Component { | ||
constructor(props, context){ | ||
super(props, context) | ||
} | ||
render() { | ||
return (<p>foo</p>) | ||
} | ||
} | ||
|
||
let tflow = new TFlow(), | ||
ContentLoader = withContentLoader(tComponent) | ||
|
||
describe('ContentLoader Component', () => { | ||
it('should render correctly', () => { | ||
let contentLoader = renderer.create(<ContentLoader flow={tflow} message={tflow.response}/>), | ||
tree = contentLoader.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
|
||
let contentLoader = TestUtils.renderIntoDocument(<ContentLoader flow={tflow} message={tflow.response}/>) | ||
|
||
it('should handle updateContent', () => { | ||
tflow.response.content = 'foo' | ||
contentLoader.updateContent({flow: tflow, message: tflow.response}) | ||
expect(contentLoader.state.request).toEqual(undefined) | ||
expect(contentLoader.state.content).toEqual('foo') | ||
// when content length is 0 or null | ||
tflow.response.contentLength = 0 | ||
tflow.response.content = undefined | ||
contentLoader.updateContent({flow: tflow, message: tflow.response}) | ||
expect(contentLoader.state.request).toEqual(undefined) | ||
expect(contentLoader.state.content).toEqual('') | ||
}) | ||
|
||
it('should handle componentWillReceiveProps', () => { | ||
contentLoader.updateContent = jest.fn() | ||
contentLoader.componentWillReceiveProps({flow: tflow, message: tflow.request}) | ||
expect(contentLoader.updateContent).toBeCalled() | ||
}) | ||
|
||
it('should handle requestComplete', () => { | ||
expect(contentLoader.requestComplete(tflow.request, {})).toEqual(undefined) | ||
// request == this.state.request | ||
contentLoader.state.request = tflow.request | ||
contentLoader.requestComplete(tflow.request, {}) | ||
expect(contentLoader.state.content).toEqual(tflow.request.responseText) | ||
expect(contentLoader.state.request).toEqual(undefined) | ||
}) | ||
|
||
it('should handle requestFailed', () => { | ||
console.error = jest.fn() | ||
expect(contentLoader.requestFailed(tflow.request, {})).toEqual(undefined) | ||
//request == this.state.request | ||
contentLoader.state.request = tflow.request | ||
contentLoader.requestFailed(tflow.request, 'foo error') | ||
expect(contentLoader.state.content).toEqual('Error getting content.') | ||
expect(contentLoader.state.request).toEqual(undefined) | ||
expect(console.error).toBeCalledWith('foo error') | ||
}) | ||
|
||
it('should handle componentWillUnmount', () => { | ||
contentLoader.state.request = { abort : jest.fn() } | ||
contentLoader.componentWillUnmount() | ||
expect(contentLoader.state.request.abort).toBeCalled() | ||
}) | ||
}) |
85 changes: 85 additions & 0 deletions
85
web/src/js/__tests__/components/ContentView/ContentViewSpec.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,85 @@ | ||
import React from 'react' | ||
import renderer from 'react-test-renderer' | ||
import TestUtils from 'react-dom/test-utils' | ||
import { Provider } from 'react-redux' | ||
import { ViewServer, ViewImage, PureViewServer, Edit } from '../../../components/ContentView/ContentViews' | ||
import { TFlow, TStore } from '../../ducks/tutils' | ||
import mockXMLHttpRequest from 'mock-xmlhttprequest' | ||
|
||
global.XMLHttpRequest = mockXMLHttpRequest | ||
let tflow = new TFlow() | ||
|
||
describe('ViewImage Component', () => { | ||
let viewImage = renderer.create(<ViewImage flow={tflow} message={tflow.response}/>), | ||
tree = viewImage.toJSON() | ||
|
||
it('should render correctly', () => { | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
}) | ||
|
||
describe('ViewServer Component', () => { | ||
let store = TStore(), | ||
setContentViewDescFn = jest.fn(), | ||
setContentFn = jest.fn() | ||
|
||
it('should render correctly and connect to state', () => { | ||
let provider = renderer.create( | ||
<Provider store={store}> | ||
<ViewServer | ||
setContentViewDescription={setContentViewDescFn} | ||
setContent={setContentFn} | ||
flow={tflow} | ||
message={tflow.response} | ||
/> | ||
</Provider>), | ||
tree = provider.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
|
||
let viewServer = renderer.create( | ||
<PureViewServer | ||
showFullContent={true} | ||
maxLines={10} | ||
setContentViewDescription={setContentViewDescFn} | ||
setContent={setContentViewDescFn} | ||
flow={tflow} | ||
message={tflow.response} | ||
content={JSON.stringify({lines: [['k1', 'v1']]})} | ||
/> | ||
) | ||
tree = viewServer.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
|
||
it('should handle componentWillReceiveProps', () => { | ||
// case of fail to parse content | ||
let viewSever = TestUtils.renderIntoDocument( | ||
<PureViewServer | ||
showFullContent={true} | ||
maxLines={10} | ||
setContentViewDescription={setContentViewDescFn} | ||
setContent={setContentViewDescFn} | ||
flow={tflow} | ||
message={tflow.response} | ||
content={JSON.stringify({lines: [['k1', 'v1']]})} | ||
/> | ||
) | ||
viewSever.componentWillReceiveProps({...viewSever.props, content: '{foo' }) | ||
let e = '' | ||
try {JSON.parse('{foo') } catch(err){ e = err.message} | ||
expect(viewSever.data).toEqual({ description: e, lines: [] }) | ||
}) | ||
}) | ||
|
||
describe('Edit Component', () => { | ||
it('should render correctly', () => { | ||
let edit = renderer.create(<Edit | ||
content="foo" | ||
onChange={jest.fn} | ||
flow={tflow} | ||
message={tflow.response} | ||
/>), | ||
tree = edit.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
}) |
15 changes: 15 additions & 0 deletions
15
web/src/js/__tests__/components/ContentView/DownloadContentButtonSpec.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,15 @@ | ||
import React from 'react' | ||
import renderer from 'react-test-renderer' | ||
import DownloadContentButton from '../../../components/ContentView/DownloadContentButton' | ||
import { TFlow } from '../../ducks/tutils' | ||
|
||
let tflow = new TFlow() | ||
describe('DownloadContentButton Component', () => { | ||
it('should render correctly', () => { | ||
let downloadContentButton = renderer.create( | ||
<DownloadContentButton flow={tflow} message={tflow.response}/> | ||
), | ||
tree = downloadContentButton.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
}) |
37 changes: 37 additions & 0 deletions
37
web/src/js/__tests__/components/ContentView/MetaViewsSpec.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
import React from 'react' | ||
import renderer from 'react-test-renderer' | ||
import { ContentEmpty, ContentMissing, ContentTooLarge } from '../../../components/ContentView/MetaViews' | ||
import { TFlow } from '../../ducks/tutils' | ||
|
||
let tflow = new TFlow() | ||
|
||
describe('ContentEmpty Components', () => { | ||
it('should render correctly', () => { | ||
let contentEmpty = renderer.create(<ContentEmpty flow={tflow} message={tflow.response}/>), | ||
tree = contentEmpty.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
}) | ||
|
||
describe('ContentMissing Components', () => { | ||
it('should render correctly', () => { | ||
let contentMissing = renderer.create(<ContentMissing flow={tflow} message={tflow.response}/>), | ||
tree = contentMissing.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
}) | ||
|
||
describe('ContentTooLarge Components', () => { | ||
it('should render correctly', () => { | ||
let clickFn = jest.fn(), | ||
uploadContentFn = jest.fn(), | ||
contentTooLarge = renderer.create(<ContentTooLarge | ||
flow={tflow} | ||
message={tflow.response} | ||
onClick={clickFn} | ||
uploadContent={uploadContentFn} | ||
/>), | ||
tree = contentTooLarge.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
}) |
39 changes: 39 additions & 0 deletions
39
web/src/js/__tests__/components/ContentView/ShowFullContentButtonSpec.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,39 @@ | ||
import React from 'react' | ||
import renderer from 'react-test-renderer' | ||
import { Provider } from 'react-redux' | ||
import ConnectedComponent, { ShowFullContentButton } from '../../../components/ContentView/ShowFullContentButton' | ||
import { TStore } from '../../ducks/tutils' | ||
|
||
|
||
describe('ShowFullContentButton Component', () => { | ||
let setShowFullContentFn = jest.fn(), | ||
showFullContentButton = renderer.create( | ||
<ShowFullContentButton | ||
setShowFullContent={setShowFullContentFn} | ||
showFullContent={false} | ||
visibleLines={10} | ||
contentLines={20} | ||
/> | ||
), | ||
tree = showFullContentButton.toJSON() | ||
|
||
it('should render correctly', () => { | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
|
||
it('should handle click', () => { | ||
tree.children[0].props.onClick() | ||
expect(setShowFullContentFn).toBeCalled() | ||
}) | ||
|
||
it('should connect to state', () => { | ||
let store = TStore(), | ||
provider = renderer.create( | ||
<Provider store={store}> | ||
<ConnectedComponent/> | ||
</Provider> | ||
), | ||
tree = provider.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
}) |
12 changes: 12 additions & 0 deletions
12
web/src/js/__tests__/components/ContentView/UploadContentButtonSpec.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,12 @@ | ||
import React from 'react' | ||
import renderer from 'react-test-renderer' | ||
import UploadContentButton from '../../../components/ContentView/UploadContentButton' | ||
|
||
describe('UpdateContentButton Component', () => { | ||
it('should render correctly', () => { | ||
let uploadContentFn = jest.fn(), | ||
uploadContentButton = renderer.create(<UploadContentButton uploadContent={uploadContentFn}/>), | ||
tree = uploadContentButton.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
}) |
38 changes: 38 additions & 0 deletions
38
web/src/js/__tests__/components/ContentView/ViewSelectorSpec.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,38 @@ | ||
import React from 'react' | ||
import renderer from 'react-test-renderer' | ||
import ConnectedComponent, { ViewSelector } from '../../../components/ContentView/ViewSelector' | ||
import { Provider } from 'react-redux' | ||
import { TStore } from '../../ducks/tutils' | ||
|
||
|
||
describe('ViewSelector Component', () => { | ||
let contentViews = ['Auto', 'Raw', 'Text'], | ||
activeView = 'Auto', | ||
setContentViewFn = jest.fn(), | ||
viewSelector = renderer.create( | ||
<ViewSelector contentViews={contentViews} activeView={activeView} setContentView={setContentViewFn}/> | ||
), | ||
tree = viewSelector.toJSON() | ||
|
||
it('should render correctly', () => { | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
|
||
it('should handle click', () => { | ||
let mockEvent = { preventDefault: jest.fn() }, | ||
tab = tree.children[1].children[0].children[1] | ||
tab.props.onClick(mockEvent) | ||
expect(mockEvent.preventDefault).toBeCalled() | ||
}) | ||
|
||
it('should connect to state', () => { | ||
let store = TStore(), | ||
provider = renderer.create( | ||
<Provider store={store}> | ||
<ConnectedComponent/> | ||
</Provider> | ||
), | ||
tree = provider.toJSON() | ||
expect(tree).toMatchSnapshot() | ||
}) | ||
}) |
11 changes: 11 additions & 0 deletions
11
web/src/js/__tests__/components/ContentView/__snapshots__/ContentLoaderSpec.js.snap
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
// Jest Snapshot v1, https://goo.gl/fbAQLP | ||
|
||
exports[`ContentLoader Component should render correctly 1`] = ` | ||
<div | ||
className="text-center" | ||
> | ||
<i | ||
className="fa fa-spinner fa-spin" | ||
/> | ||
</div> | ||
`; |
52 changes: 52 additions & 0 deletions
52
web/src/js/__tests__/components/ContentView/__snapshots__/ContentViewSpec.js.snap
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,52 @@ | ||
// Jest Snapshot v1, https://goo.gl/fbAQLP | ||
|
||
exports[`Edit Component should render correctly 1`] = ` | ||
<div | ||
className="text-center" | ||
> | ||
<i | ||
className="fa fa-spinner fa-spin" | ||
/> | ||
</div> | ||
`; | ||
|
||
exports[`ViewImage Component should render correctly 1`] = ` | ||
<div | ||
className="flowview-image" | ||
> | ||
<img | ||
alt="preview" | ||
className="img-thumbnail" | ||
src="/flows/d91165be-ca1f-4612-88a9-c0f8696f3e29/response/content" | ||
/> | ||
</div> | ||
`; | ||
|
||
exports[`ViewServer Component should render correctly and connect to state 1`] = ` | ||
<div | ||
className="text-center" | ||
> | ||
<i | ||
className="fa fa-spinner fa-spin" | ||
/> | ||
</div> | ||
`; | ||
|
||
exports[`ViewServer Component should render correctly and connect to state 2`] = ` | ||
<div> | ||
<pre> | ||
<div> | ||
<span | ||
className="k" | ||
> | ||
1 | ||
</span> | ||
<span | ||
className="v" | ||
> | ||
1 | ||
</span> | ||
</div> | ||
</pre> | ||
</div> | ||
`; |
13 changes: 13 additions & 0 deletions
13
web/src/js/__tests__/components/ContentView/__snapshots__/DownloadContentButtonSpec.js.snap
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,13 @@ | ||
// Jest Snapshot v1, https://goo.gl/fbAQLP | ||
|
||
exports[`DownloadContentButton Component should render correctly 1`] = ` | ||
<a | ||
className="btn btn-default btn-xs" | ||
href="/flows/d91165be-ca1f-4612-88a9-c0f8696f3e29/response/content" | ||
title="Download the content of the flow." | ||
> | ||
<i | ||
className="fa fa-download" | ||
/> | ||
</a> | ||
`; |
Oops, something went wrong.
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I'm not sure if I understand this without a comment - which purpose does this serve here and in ContentViewSpec.js?
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Because we use
XMLHttpRequest
here:mitmproxy/web/src/js/components/ContentView/ContentLoader.jsx
Line 59 in 5f91b7a
Introducing
mock-xmlhttprequest
dependency can save us a lot of troubles.There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Ok, but we never tell the mock function what to return, so what would happen if we didn't do this here?
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
If we don't mock
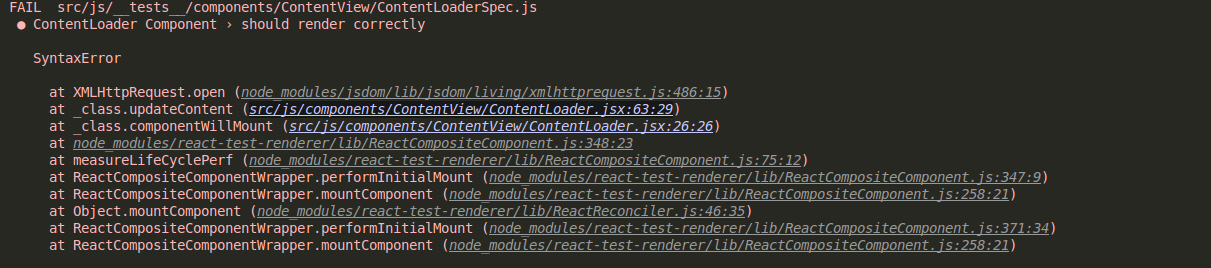
XMLHttpRequest
, it fails like this: