AdapterView state (RecyclerView, ViewPager)
// in ViewModel
private val gridState = RecyclerViewState<AntonioModel>()
private fun onGridRefreshed() {
gridState.currentList.clear()
gridState.currentList.addAll(count = 7)
gridState.notifyDataSetChanged()
}
// in Fragment or Activity
private fun initState(){
// Extension is ready!
binding.recyclerView.setState(viewModel.recommendGridState)
}
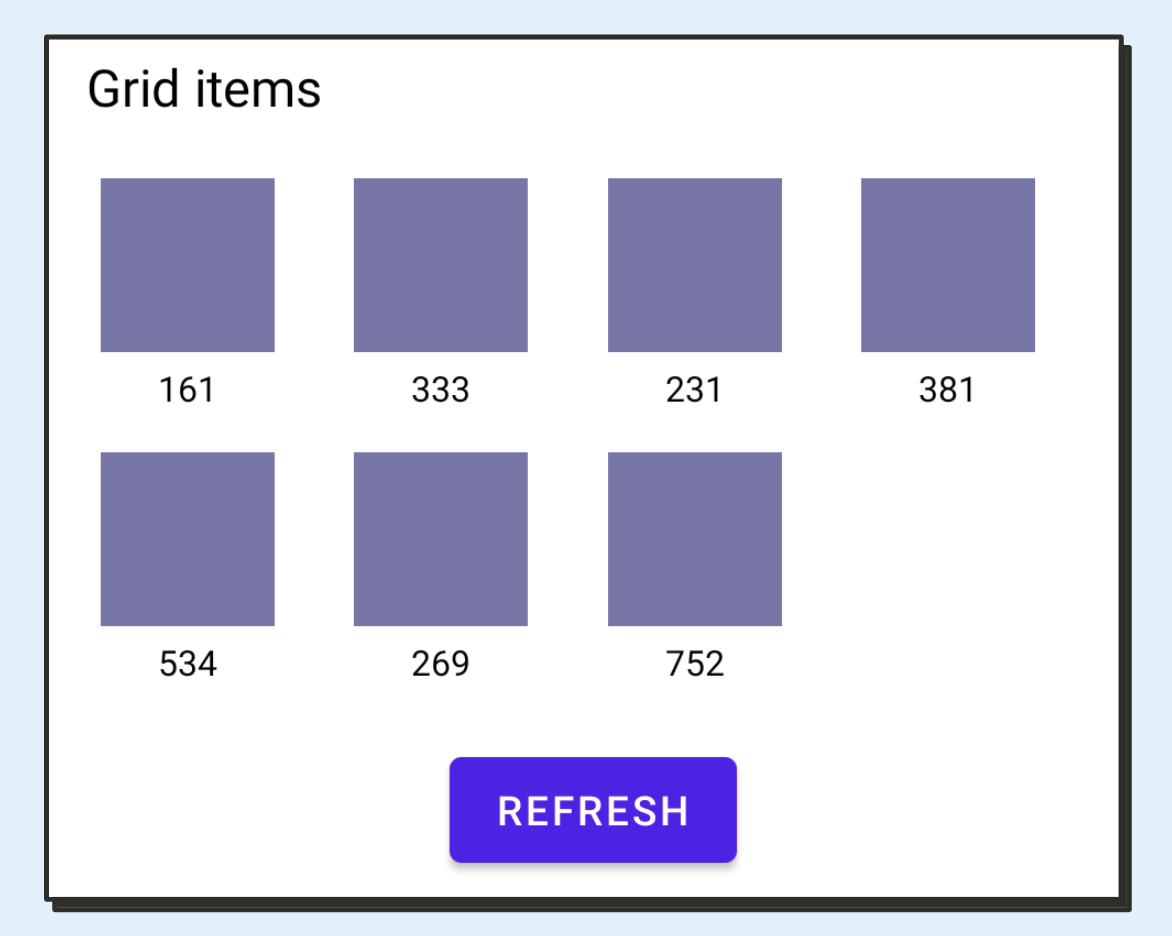
It would be not so easy to make AdapterView as a state in your view model because of some reasons as follows.
-
AdapterView needs to be notified.
-
It could be expressed as a live data or state flow if you only need
NotifyDataSetChanged
orSubmitList
. But sometimes you need to notify specific indices for some reason (e.g, Fast inserted chat in streaming). For this situation, You need to make another model for each notifying action. -
If there are lots of AdapterViews in one screen, It needs the same amount of models for the notifying action.
-
-
Adapter cannot be inside your view model.
-
You might think it would be an easy solution to put the adapter in your view model for this situation. But It's not a good solution in terms of test code because of Android framework dependencies on Adapter.
-
We inject dependencies for adapter notify APIs with Interface to RecyclerViewState, ViewPagerState for solving the problems.
Now You just notify in your view model and you also write some test code for your view model!
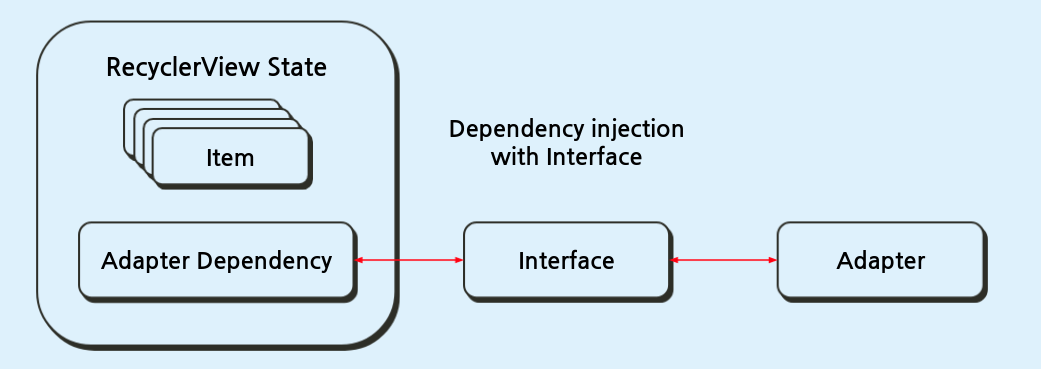
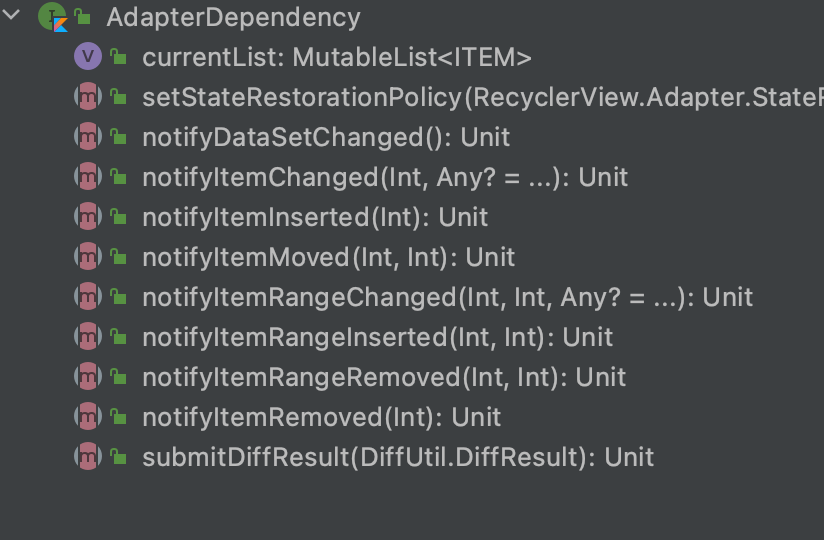
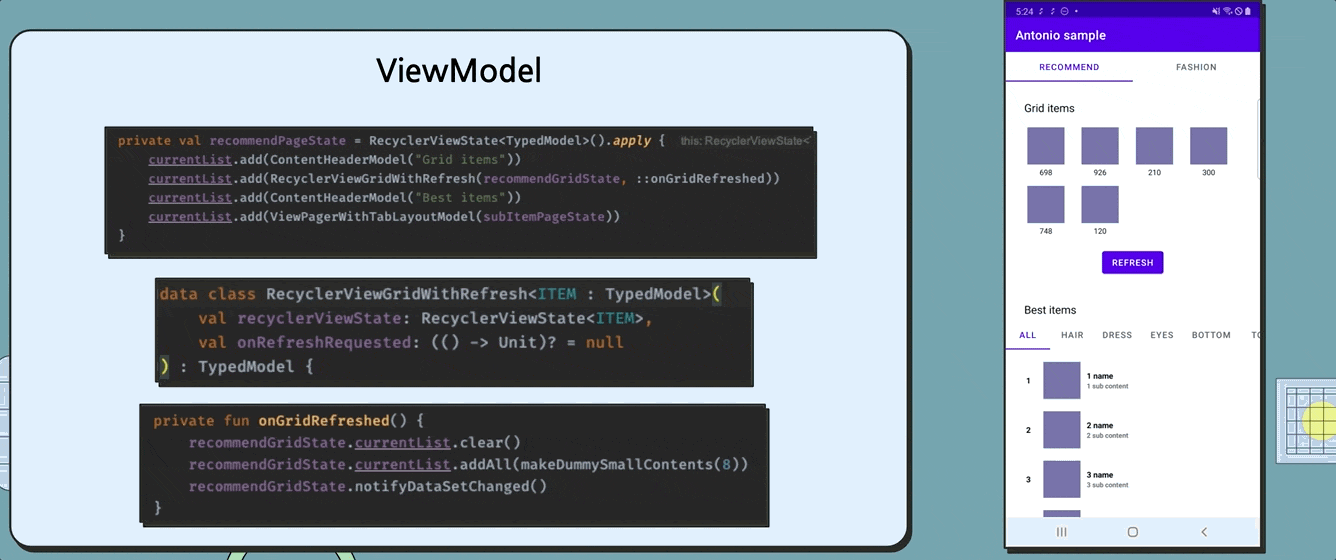
You need to change the global thread executor builder first for your test code!
val executorForTest= Executors.newSingleThreadExecutor()
AntonioSettings.setExecutorBuilder { executorForTest }