-
Notifications
You must be signed in to change notification settings - Fork 4
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Texture mapping to a sphere #257
Comments
@banesullivan has done a lot of the implementation of texture mapping, so he'll probably correct me if I'm wrong, but I think the issue is that there's no way for import pyvista
mesh = pyvista.Plane()
tex = pyvista.read_texture("Earth.png")
mesh.texture_map_to_plane(inplace=True)
mesh.plot(texture=tex) What the So, what you'd have to do if you wanted it properly mapped is to get the right relationship of the points on the sphere to a plane (effectively, UV Mapping), and then use that projection to apply your texture. If you do choose to project to a sphere, please post your solution here so others can benefit from it. |
Thanks, that helped me understand why the texture gets mapped so. |
I searched the documentation site and found out that texture coordinates can be defined using the |
That's correct! Keep your UV coordinates scaled between 0 and 1 and map each vertex to its coordinates in the UV image space. FYI, there is a |
I created the globe example manually with the code in this PVGeo filter Basically, I reproject the sphere's coordinates to a Lat/Lon space (thus a 2D plane) and essentially map the UV coordinates linearly there. On a sphere, you HAVE to make sure there is an open edge along one longitude or else you will get artifacts of the texture folding back on itself. I outlined all of this in this ParaView discourse thread |
I used this slightly modified form of the relation given in Wikipedia: import pyvista
import math
import numpy
sphere = pyvista.Sphere(radius=1, theta_resolution=120, phi_resolution=120, start_theta=270.001, end_theta=270)
sphere.t_coords = numpy.zeros((sphere.points.shape[0], 2))
for i in range(sphere.points.shape[0]):
sphere.t_coords[i] = [0.5 + math.atan2(-sphere.points[i, 0], sphere.points[i, 1])/(2 * math.pi), 0.5 + math.asin(sphere.points[i, 2])/math.pi]
tex = pyvista.read_texture("Earth.png")
sphere.plot(texture=tex) (I've used a sphere of radius 1, so I don't have to explicitly calculate the unit vector) |
Awesome work, @KDarshan20! Thanks for sharing this |
Thanks to you as well!
|
Nice, now need to find a Mars! |
Found Mars. import pyvista
import math
import numpy
import numpy as np
sphere = pyvista.Sphere(radius=1, theta_resolution=120, phi_resolution=120,
start_theta=270.001, end_theta=270)
sphere.t_coords = numpy.zeros((sphere.points.shape[0], 2))
sphere.t_coords[:, 0] = 0.5 + np.arctan2(-sphere.points[:, 0], sphere.points[:, 1])/(2 * math.pi)
sphere.t_coords[:, 1] = 0.5 + np.arcsin(sphere.points[:, 2]) / math.pi
tex = pyvista.read_texture("mars.jpg")
# sphere.plot(texture=tex, smooth_shading=False, background='black', show_axes=False)
# with stars
pl = pyvista.Plotter()
pl.add_background_image('stars.jpg')
pl.add_mesh(sphere, texture=tex, smooth_shading=False)
pl.show() Marshttp://www.solarviews.com/raw/mars/marscyl1l.jpg Stars |
Great, thanks Alex! |
Nice! @akaszynski, let's add that to pyvista/pyvista#940 |
A source of textures for all planets, the sun, etc.: https://www.solarsystemscope.com/textures/ |
Awesome! I think I have to make a solar system example then! |
@akaszynsk If the radius needs to be defined as 1738 km, what I need to do? I already tried your code but it doesn't work. |
Hello, Please post the full code you used. |
Hi @akaszynski, I am sorry for my late response but here is the code and the output. I am creating a sphere with the real radius of the moon. (1738 km) ` sphere.t_coords = np.zeros((sphere.points.shape[0], 2)) sphere.t_coords[:, 0] = 0.5 + np.arctan2(-sphere.points[:, 0], sphere.points[:, 1])/(2 * math.pi) tex = pv.read_texture("2k_moon.jpg") p.add_mesh(sphere, texture=tex) |
BTW, if anyone is using this with import pyvista
import math
import numpy
import numpy as np
from pyvista import examples
sphere = pyvista.Sphere(radius=1, theta_resolution=120, phi_resolution=120,
start_theta=270.001, end_theta=270)
sphere.active_t_coords = numpy.zeros((sphere.points.shape[0], 2))
sphere.active_t_coords[:, 0] = 0.5 + np.arctan2(-sphere.points[:, 0], sphere.points[:, 1])/(2 * math.pi)
sphere.active_t_coords[:, 1] = 0.5 + np.arcsin(sphere.points[:, 2]) / math.pi
mars = pyvista.Texture(examples.download_mars_jpg())
stars = examples.download_stars_jpg()
# with stars
pl = pyvista.Plotter()
pl.add_background_image(stars)
pl.add_mesh(sphere, texture=mars, smooth_shading=False)
pl.show() |
Definitely will try that one! |
Are there any methods to control the mapping position with coordinates? Many of the methods found are full package methods. I want a method to use coordinates for local mapping |
Hello,
I found PyVista while surfing the internet for a library that would help me in my project, a 3D solar system animation. So while working on the project I came across a problem: I couldn't get the textures mapped correctly. For instance,
Gave the following output:
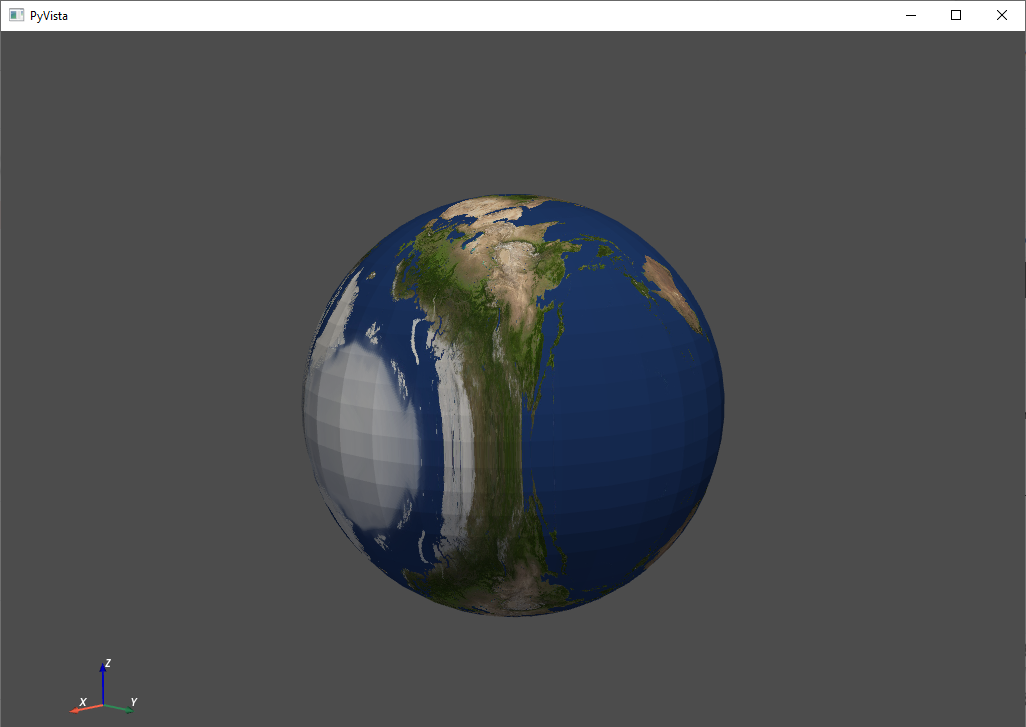
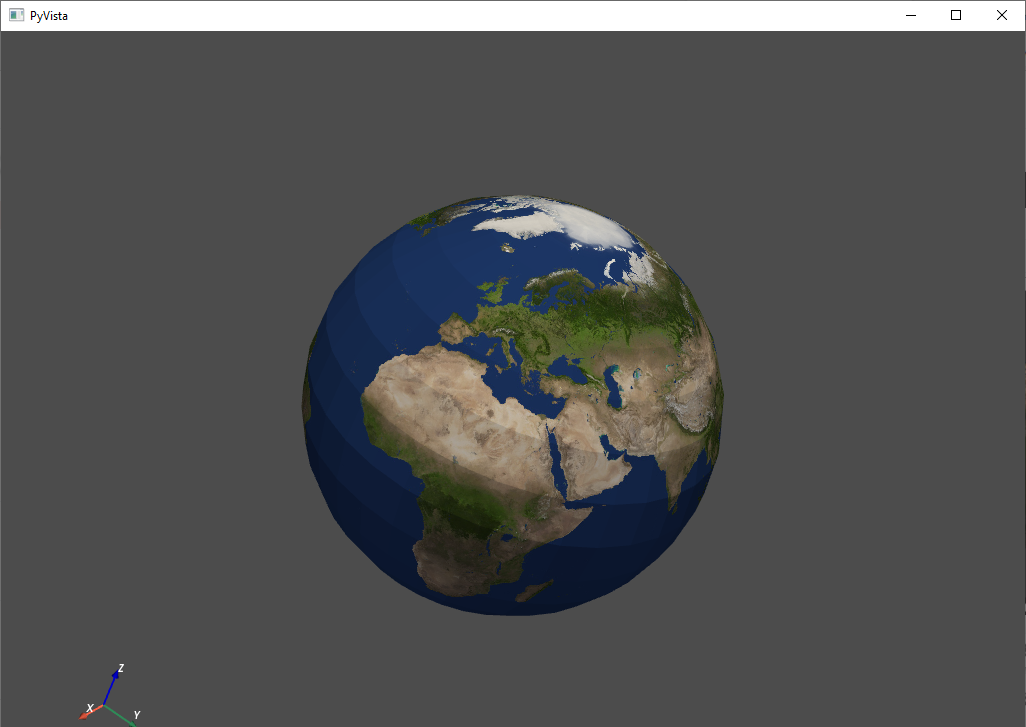
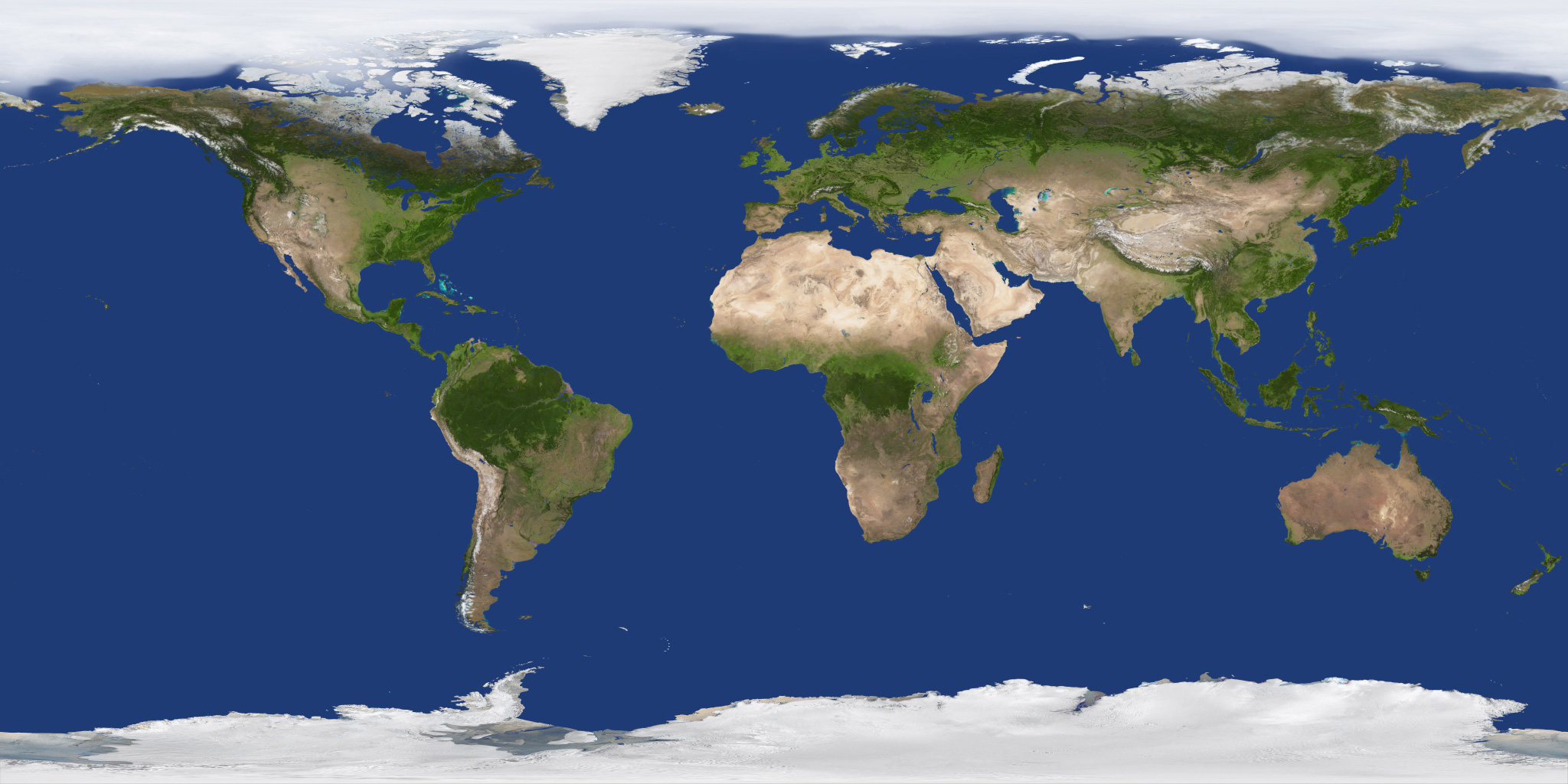
While I want it to look like the in-built globe example:
Earth.png:
The text was updated successfully, but these errors were encountered: