-
Notifications
You must be signed in to change notification settings - Fork 78
English Documents
PPTShow is an open source free Java generated PPT toolkit .
It is completely independent of other programs on any system to help users use code to generate PPT files .
But PPT to generate pictures , PPT to generate video functions are achieved by calling Microsoft Office , so only available under Windows .
- adding pages to the PPT;
- adding text, graphics, line segments, pictures to the page;
- text support for sub-paragraph text, and support for multiple styles of text appearing in the same line;
- support background music;
- support for PPT page background style settings;
- support for element and page animation, element animation support for order adjustment and moment setting;
- support for common element style settings;
- support PPT to video and PPT to picture in Windows environment (please make sure Microsoft Office is installed and activated)
- can only generate pptx files, do not support generating ppt files
- not recommended to use PPTShow to read PPT files (reading is still in demo state, not suitable for production environment)
- do not support table elements at the moment
Our current plans that are not yet developed are:
- table element support
- embedded font support
Q: Can it be used for commercial purposes?
A: PPTShow follows the Apache License 2.0 open source agreement which allows users to use it for purposes including, but not limited to, commercial use and personal study use.
Q: Is it possible to generate PPT documents in Linux environment?
A:Support
Q:Does the PPT generate images with messy Chinese or default fonts?
A: No, we generate pictures by calling the system Office, so there is no need to worry about similar problems.
Q: Is it possible to set the animation order of elements?
A: We support setting the order and common operations such as appearing at the same time and appearing after the previous animation is finished.
Q:Does PPTShow guarantee the availability or take responsibility for the loss caused by code problems?
A: PPTShow does not guarantee the usability of the project and is not responsible for any loss, please test the function by yourself before using it.
This tutorial will guide you through the Java code to generate a PPT file containing HelloWorld
You can introduce jar packages by maven or by importing them manually
<dependency>
<groupId>cc.pptshow</groupId>
<artifactId>pptshow</artifactId>
<version>1.3</version>
</dependency>
Download address: https://s01.oss.sonatype.org/service/local/repositories/releases/content/cc/pptshow/pptshow/1.3/pptshow-1.3.jar
Create a new Java class file named Main
.
Copy the following code into the project and run it.
You'll have a PPT file containing HelloWorld!
import cc.pptshow.ppt.domain.*;
import cc.pptshow.ppt.element.impl.*;
import cc.pptshow.ppt.show.PPTShow;
import cc.pptshow.ppt.show.PPTShowSide;
public class Main {
public static void main(String[] args) {
//Create a new PPT object
PPTShow pptShow = PPTShow.build();
//Create a new PPT page
PPTShowSide side = PPTShowSide.build();
//Create an in-line text object with the text set to Hello World
PPTInnerText pptInnerText = PPTInnerText.build("Hello World");
//Create an in-line text style object so that the text color is red
PPTInnerTextCss pptInnerTextCss = PPTInnerTextCss.build().setColor("FF00000");
//Binding in-line text and style objects
pptInnerText.setCss(pptInnerTextCss);
//Create a line text object from in-line text and create a text object from a line text object
PPTText pptText = PPTText.build(PPTInnerLine.build(pptInnerText));
//Adding text objects to a PPT page
side.add(pptText);
//Add PPT page inside PPT
pptShow.add(side);
//Output to file
pptShow.toFile("C:/Users/xxx/Desktop/test.pptx");
}
}
If things go well, you will get a file similar to the one below
PPTShow is different from common PPT generation programs such as POI, PPTShow almost completely shields the underlying XML code of PPT itself.
PPTShow considers each small indivisible element as an object, and the objects at different levels are nested with each other to form the whole PPT.
For example, as shown in the figure below, we consider the whole of PPT, different pages, and various elements in the pages are all one object.
Each element object has a corresponding style sheet object, and style binding can be achieved by means of element object.setCss(style sheet object)
.
Element Object | Element Meaning | Style Sheet Object |
---|---|---|
PPTShowSide | PPT page | PPTSideCss |
PPTImg | PPT images | PPTImgCss |
PPTShape | PPT graphics | PPTShapeCss |
PPTLine | PPT Line Segment | PPTLineCss |
PPTText | PPT Text | PPTTextCss |
PPTInnerLine | Paragraphs in PPT text | PPTInnerLineCss |
PPTInnerText | A style minimum unit in a PPT text paragraph | PPTInnerTextCss |
- PPTShow objects need to manually call
close()
method to delete temporary files after use - All element objects and style sheet objects in this project implement the
cloneable
interface, and are deep clones.
The PPTShow object represents a PPT file, and the system builds the necessary components of the file in the temporary directory and outputs the assembled file to the specified location when the user specifies the output.
Therefore you must perform the close operation manually to delete the temporary directory files, otherwise the more temporary directory files are piled up may lead to insufficient disk space.
You can create a PPTShow object in the following two ways, which do not differ in any way.
PPTShow pptShow = PPTShow.build();
PPTShow pptShow = new PPTShow();
PPTShowSide is the PPT page object, which will be mentioned later in the document.
Insert at the end of the document page:
void add(PPTShowSide pptShowSide)
Insert into a page in the middle of the document:
/**
* indexId Indicates that the index of the specified page is to be inserted, and the index starts from 0
*/
void add(PPTShowSide pptShowSide, int indexId)
Generate a PPT file in pptx format based on PPTShow content
/**
* path Output path, please end with .pptx
*/
void toFile(String path)
The cache will be cleared after closing the object, and the closed object cannot generate files
void close()
PPT page object represents a page of PPT, a page in which usually consists of many elements, many pages make up the PPT
You can create a PPTShowSide object in the following two ways, which do not differ in any way.
PPTShowSide pptShowSide = PPTShowSide.build();
PPTShowSide pptShowSide = new PPTShowSide();
Elements follow the index of the smaller elements in the lower level, the index of the larger elements in the upper level
void add(PPTElement pptElement)
/**
* indexId Indicates that the index of the specified page is to be inserted, and the index starts from 0
*/
void add(PPTElement pptElement, int indexId)
void addAll(List<PPTElement> pptElements)
Read the current element list information by Get method
List<PPTElement> getElements()
You can set the background music of a slide with the backgroundMusic
property
Currently, you can only play to the end of the slide, but not to the end of the slide.
/**
* Background music files
*/
@Getter
@Setter
private String backgroundMusic;
You can set the time for the current slide to automatically switch to the next page via the autoPagerTime
property
Unit: milliseconds, default is 0, which means no automatic page change
/**
* The time of auto-page change
* unit milliseconds, default is 0 means no automatic page change
*/
@Getter
@Setter
private long autoPagerTime = 0L;
You can set the animation of slide switching by setting pageSwitchingType
The default is the cut-out effect (i.e. no style, just a hard switch)
private PageSwitchingType pageSwitchingType = PageSwitchingType.DEFAULT;
The set value corresponds to the enumeration class PageSwitchingType
.
The enumeration class contains the Chinese name of the action style of the slide (parameter 1), you can check or test it yourself.
DEFAULT("切出(默认效果)", PageSwitchingType.DEFAULT_XML),
SMOOTH("平滑", PageSwitchingType.SMOOTH_XML),
FADE_OUT("淡出", PageSwitchingType.FADE_OUT_XML),
ERASE("擦除", PageSwitchingType.ERASE_XML),
SHAPE("形状", PageSwitchingType.SHAPE_XML),
DISSOLVE("溶解", PageSwitchingType.DISSOLVE_XML),
NEWS_FLASH("新闻快报", PageSwitchingType.NEWS_FLASH_XML),
SPOKES("轮辐", PageSwitchingType.SPOKES_XML),
BLIND("百叶窗", PageSwitchingType.BLIND_XML),
COMB("梳理", PageSwitchingType.COMB_XML),
TAKING("抽出", PageSwitchingType.TAKING_XML),
SEGMENTATION("分割", PageSwitchingType.SEGMENTATION_XML),
LINE("线条", PageSwitchingType.LINE_XML),
BOARD("棋盘", PageSwitchingType.BOARD_XML),
LAUNCH("推出", PageSwitchingType.LAUNCH_XML),
INSERT("插入", PageSwitchingType.INSERT_XML),
PAGE_CURLING("页面卷曲", PageSwitchingType.PAGE_CURLING_XML),
CUBE("立方体", PageSwitchingType.CUBE_XML),
BOX("框", PageSwitchingType.BOX_XML),
PLAIN("飞机", PageSwitchingType.PLAIN_XML),
OPEN_DOOR("开门", PageSwitchingType.OPEN_DOOR_XML),
STRIPPING("剥离", PageSwitchingType.STRIPPING_XML),
RANDOM("随机", PageSwitchingType.RANDOM_XML)
PPTSideCss
is the style sheet corresponding to PPTShowSide
Currently there is only one property that allows user customization
private Background background;
Background style, please refer to [Reference Document] - [General Style] - [background background background]
PPTImg object represents the image element in PPT
You can create a PPTImg object in the following two ways, which do not differ in any way.
PPTImg pptImg = PPTImg.build();
PPTImg pptImg = new PPTImg();
You can also specify the image at the time of creation. There are two ways to create a PPTImg object, and they don't make any difference
PPTImg pptImg = PPTImg.build(String file);
PPTImg pptImg = new PPTImg(String file);
Set what image corresponds to the current PPTImg object, you can specify it directly when you create the object, or you can manually call the following method after creation to specify the embedded image path.
Support png, jpg, gif, svg; webp format is not supported
Note: Only the absolute path of the local file is supported; online image information such as http is not supported!
PPTImg setFile(String file)
//Entry animation
private InAnimation inAnimation;
//Appearance animation
private OutAnimation outAnimation;
Please refer to [Reference Document] - [General Styles] - [InAnimation InAnimation] and [OutAnimation OutAnimation] to set the content
Get the image path of the PPTImg object
String getFile()
You can set style information through the css
property, and we will explain the PPTImgCss
property and its meaning in detail.
@Getter
@Setter
private PPTImgCss css;
PPTImg
Corresponding stylesheet object
private double left;
Distance from the left side of the page, in cm
private double top;
Distance from the top edge of the page, in cm
private double width;
Element width in cm
private double height;
Element height, in cm
private Cutting cutting;
Crop style, please refer to [Reference Document] - [General Style] - [Cutting Crop]
private Border border;
Border style, please refer to [Reference Document] - [General Style] - [border border]
private Shadow shadow;
Border style, please refer to [Reference Document] - [General Style] - [shadow shading]
private String name = "图片";
Element name, default is "picture", this information is not visible in normal PPT screening
In scenarios such as setting element animation, the element name information will be displayed in the animation pane
Duplicate names are allowed in the same slide page
private String describe = "image";
Element description, default is "image", this information is not visible during normal PPT projection
Repeat describe is allowed on the same slide
private double angle;
Rotation angle, the clockwise rotation angle, the default is 0 means no rotation.
Legal value range: 0 ≤ angle < 360
private String removeColor;
Set the transparent color in the format of a color value expressed in hexadecimal 6 characters without the # sign, for example: FFFFFF
.
For example, a white background image can be set to white; this property is not valid for png images
PPTShape object represents the graphical elements in PPT
You can create a PPTImg object in the following two ways, which do not differ in any way.
PPTShape pptShape = PPTShape.build();
PPTShape pptShape = new PPTShape();
//Entry animation
private InAnimation inAnimation;
//Appearance animation
private OutAnimation outAnimation;
Please refer to [Reference Document] - [General Styles] - [InAnimation InAnimation] and [OutAnimation OutAnimation] to set the content
You can set the style information through the css
property, we will explain the PPTImgCss
property and its meaning in detail.
@Getter
@Setter
private PPTShapeCss css;
PPTShape
Corresponding stylesheet object
private Shape shape = new Rect();
The graphical shape, if you do not make special settings, will default to a right-angled rectangle.
Shape is an abstract class and has the following graphical implementations of it:
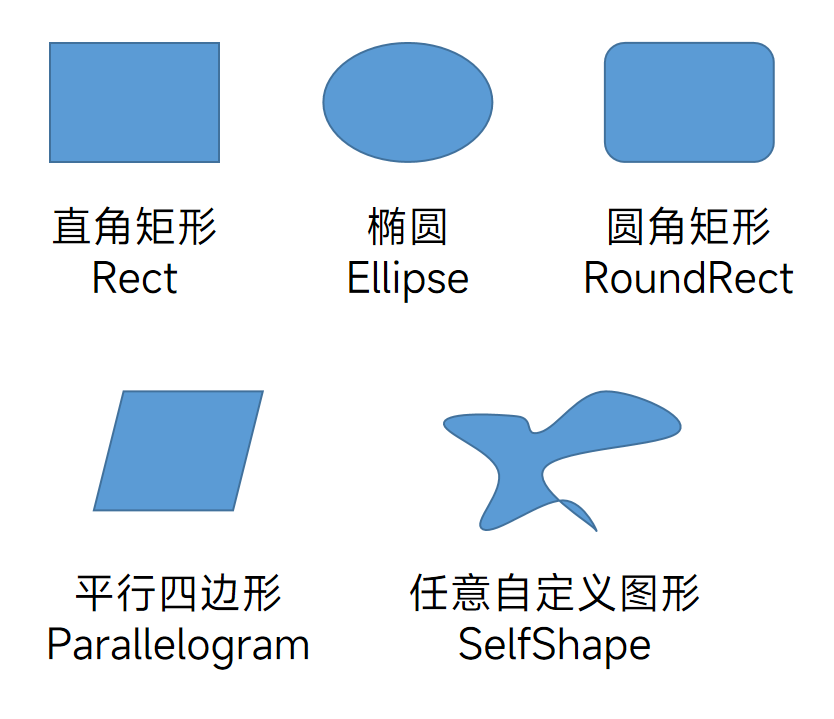
Right-angled rectangles have no more parameters to set
Displayed as a square when and only when width and height are set equal
Ellipse has no more parameters to set
Displays as a square when and only when the width and height are set equal
The rounded range parameter can be set via the get and set methods, the allowed range is 0 - 50, which means 0% - 50%.
private double fillet;
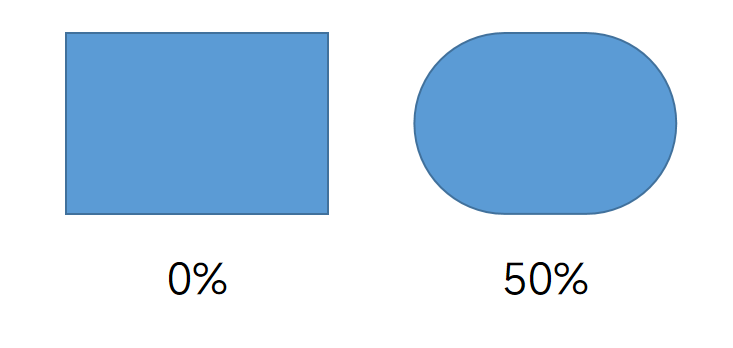
The degree of parallelogram tilt can be set by the get and set methods, the allowed range is 0-100, which means 0% - 100%
private double fillet;
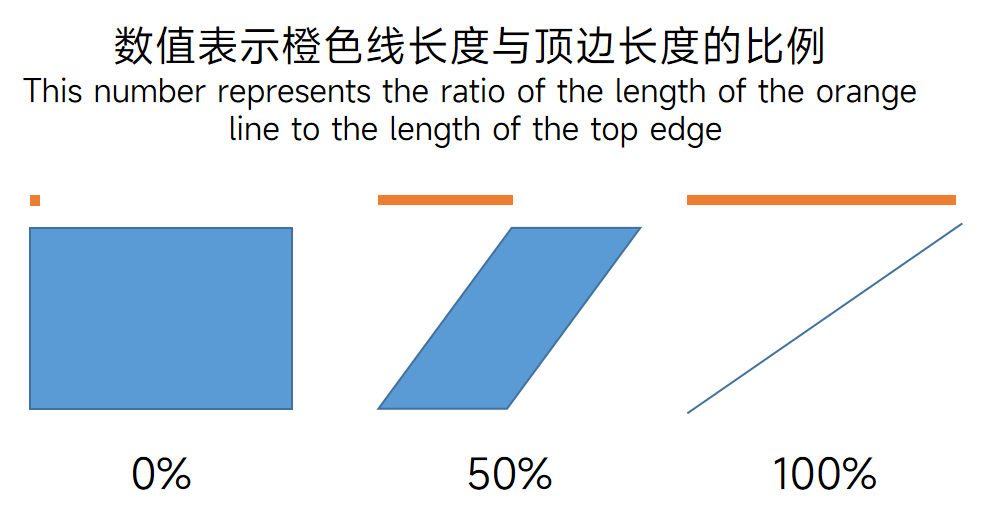
Temporary solution for graphics not implemented by the system, not recommended
/**
* A user-defined piece of XML
*/
private String custGeom;
A user-defined piece of XML is used to determine the graphical information, and the content is written directly to the file without any legality checks other than non-empty
private double left;
Distance from the left side of the page, in cm
private double top;
Distance from the top edge of the page, in cm
private double width;
Element width in cm
private double height;
Element height, in cm
private Background background;
Background style, please refer to [Reference Document] - [General Style] - [background background background]
private Border border;
Border style, please refer to [Reference Document] - [General Style] - [border border]
private String name = "图片";
Element name, default is "图片", this information is not visible in normal PPT screening
In scenarios such as setting element animation, the element name information will be displayed in the animation pane
Duplicate names are allowed in the same slide page
private double angle;
Rotation angle, the clockwise rotation angle, the default is 0 means no rotation.
Legal value range: 0 ≤ angle < 360
private boolean flipX;
Whether to flip horizontally, the default is not to flip
private boolean flipY
Whether or not to flip vertically, the default is not to flip
PPTLine represents the line segment element in PPT
You can create a PPTLine object in the following way
PPTLine pptLine = new PPTLine();
//Entry animation
private InAnimation inAnimation;
//Appearance animation
private OutAnimation outAnimation;
Please refer to [Reference Document] - [General Styles] - [InAnimation InAnimation] and [OutAnimation OutAnimation] to set the content
You can set the style information through the css
property, we will explain the PPTImgCss
property and its meaning in detail.
@Getter
@Setter
private PPTLineCss css;
PPTLine
Corresponding stylesheet object
private double left;
Distance from the left side of the page, in cm
private double top;
Distance from the top edge of the page, in cm
private String color = "333333";
Line color, hex color, without # sign
default is 333333
private double lineWidth = 0.5;
The width of the line segment, in pounds, default 0.5 pounds
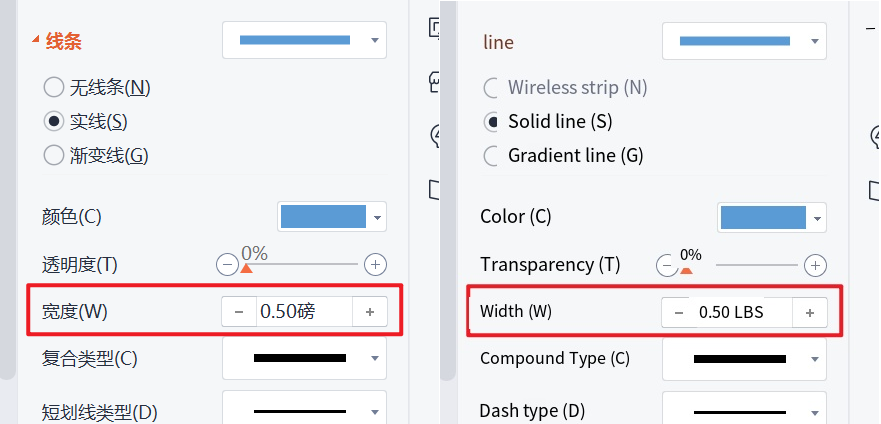
private double width;
Element width, in cm, vertical line width must be set to 0
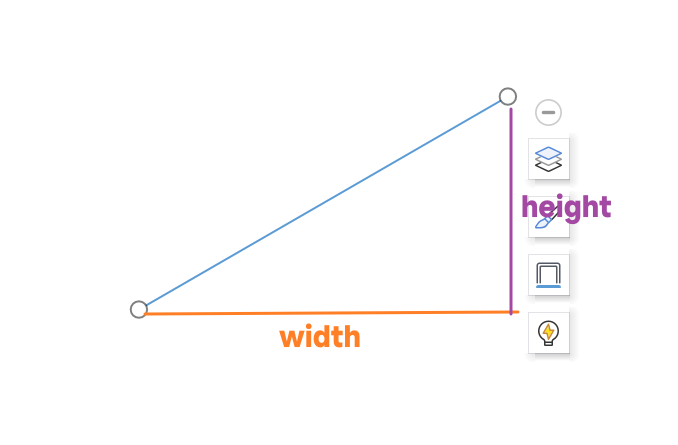
private double height;
Element height, unit cm, horizontal line height must be set to 0
private LineType type = LineType.LINE;
The direction of the line segment, the default line segment connects from the top left vertex to the bottom right vertex
If you need to connect from the top-right corner to the bottom-left corner, set the parameter to LineType.TOP_RIGHT_BOTTOM_LEFT
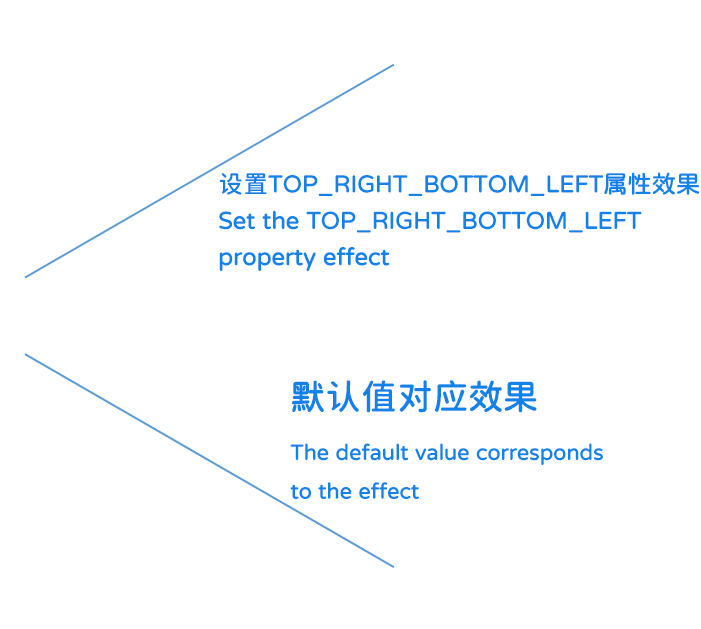
PPTText
indicates a PPT text element, note that it is the text element as a whole, not a part of it, for example, the dotted box area in the figure below is a PPT text
If we want to include different paragraphs in a PPText
object, as in the effect above, which contains two paragraphs, respectively PPTShow is a free open source Java native generation of PPT toolkit.
and support the 2010 version of the new features of PPT , does not rely on any third-party components , Linux, Windows can be used smoothly .
Each of these paragraphs is a PPTInnerLine
object.
There may also be multiple text rendering styles in a paragraph, which are close together without line breaks.
For example, there is a difference between 2010 version
and the text before and after it, which is equivalent to a PPTInnerLine
object containing three PPTInnerText
objects, the first of which has the same style as the third, and the second has a bold and red special effect. (As shown in the figure below, each part of the wireframe represents a PPTInnerText
object)
You can create a PPTText
object without any difference between the two ways by:
PPTText pptText = new PPTText();
PPTText pptText = PPTText.build();
You can also bind the PPTInnerLine
object collection directly at creation time:
When there is only one PPTInnerLine
, you can create the PPTText
object in the following way, without any difference between the two ways:
PPTText pptText = new PPTText(PPTInnerLine line);
PPTText pptText = PPTText.build(PPTInnerLine line);
When there are more than one, the PPText
object can be created in the following way, without any difference between the two ways:
PPTText pptText = new PPTText(List<PPTInnerLine> lineList);
PPTText pptText = PPTText.build(List<PPTInnerLine> textList);
You can also bind style files directly at the time of creation: the
PPTText pptText = new PPTText(PPTInnerLine line, PPTTextCss css);
PPTText pptText = PPTText.build(PPTInnerLine line, PPTTextCss css);
PPTText pptText = new PPTText(List<PPTInnerLine> lineList, PPTTextCss css);
PPTText pptText = PPTText.build(List<PPTInnerLine> textList, PPTTextCss css);
double findMinWidthSize()
The minimum required width for each paragraph without line breaks
double findMinHeightSize()
Get the minimum required height value under the current width.
In fact, even if the height in the set style is smaller than the minimum height, the text will automatically overflow and will not affect the display.
String findAllText()
Get all the text information in all paragraphs and all text within paragraphs of the text object.
Return information contains line breaks, you can use line breaks to distinguish paragraph information, but the text within the paragraph can not be distinguished.
Example:
The above PPText
object calls the findAllText()
method and gets the following text:
PPTShow is a free open source Java native generation of PPT toolkit.
support the 2010 version of the new features of PPT.
Add a paragraph at the end:
PPTText add(PPTInnerLine text)
Add multiple paragraphs at the end:
PPTText addAll(Collection<PPTInnerLine> text)
You can also directly take out the paragraph List object to modify their own operations, support get and set operations
@Setter
@Getter
private List<PPTInnerLine> lineList = Lists.newArrayList();
You can set the style information through the css
property, we will explain the PPTextCss
property and its meaning in detail.
@Getter
@Setter
private PPTTextCss css;
PPText
corresponding style sheet object
private double left;
Distance from the left side of the page, in cm
private double top;
Distance from the top edge of the page, in cm
private double width;
Element width in cm, default is minimum width
private double height;
Element height, unit: cm, default is the minimum height
private double lineHeight;
Line height, unit: times line spacing, default is 1
private Background background
Background style, please refer to [Reference Document] - [General Style] - [background background background]
private double angle;
Rotation angle, the clockwise rotation angle, the default is 0 means no rotation.
Legal value range: 0 ≤ angle < 360
private String name = "文本";
Element name, default is "text", this information is not visible in normal PPT projection
In scenarios such as setting element animation, the element name information will be displayed in the animation pane
Duplicate names are allowed in the same slide page
For the relationship between PPTText
, PPTInnerLine
, and PPTInnerText
, please refer to the documentation of PPTText
You can create a PPTInnerLine
object, without any difference between the two ways, by:
PPTInnerLine pptInnerLine = new PPTInnerLine();
PPTInnerLine pptInnerLine = PPTInnerLine.build();
You can also quickly create empty line objects directly:
PPTInnerLine pptInnerLine = PPTInnerLine buildEmptyLine();
/**
* num: empty lines
*/
PPTInnerLine pptInnerLine = PPTInnerLine buildEmptyLine(int num);
You can also bind the PPTInnerText
object collection directly at the time of creation:
When there is only one PPTInnerText
, you can create the PPTInnerLine
object in the following way, without any difference between the two ways:
PPTInnerLine pptInnerLine = new PPTInnerLine(PPTInnerText text);
PPTInnerLine pptInnerLine = PPTInnerLine.build(PPTInnerText text);
When there are more than one, the PPTInnerLine
object can be created in the following way, without any difference between the two ways:
PPTInnerLine pptInnerLine = new PPTInnerLine(List<PPTInnerText> textList);
PPTInnerLine pptInnerLine = PPTInnerLine.build(List<PPTInnerText> textList);
You can also bind style files directly at the time of creation: there is any difference between:
PPTInnerLine pptInnerLine = new PPTInnerLine(PPTInnerText text, PPTInnerLineCss css);
PPTInnerLine pptInnerLine = PPTInnerLine.build(PPTInnerText text, PPTInnerLineCss css);
PPTInnerLine pptInnerLine = new PPTInnerLine(List<PPTInnerText> textList, PPTInnerLineCss css);
PPTInnerLine pptInnerLine = PPTInnerLine.build(List<PPTInnerText> textList, PPTInnerLineCss css);
Append a paragraph at the end:
PPTInnerLine add(PPTInnerText text)
Add multiple paragraphs at the end:
PPTInnerLine addAll(Collection<PPTInnerText> text)
You can also directly take out the paragraph List object to modify their own operations, support get and set operations
@Setter
@Getter
private List<PPTInnerText> textList = Lists.newArrayList();
You can set the style information through the css
property, we will explain the PPTInnerLineCss
property and its meaning in detail.
@Getter
@Setter
private PPTInnerLineCss css;
PPTInnerLine
Corresponding stylesheet object
private double lineHeight;
Line height, unit: times line spacing, default is 1
private String align = PPTNameConstant.ALIGN_LEFT;
Alignment methods, the following alignment methods exist
-
PPTNameConstant.ALIGN_LEFT
left-aligned -
PPTNameConstant.ALIGN_RIGHT
right-aligned -
PPTNameConstant.ALIGN_CENTER
center-aligned -
PPTNameConstant.ALIGN_JUST
Align both ends -
PPTNameConstant.ALIGN_DIST
Discrete alignment
The five types correspond to the circled part of the chart below, the order from left to right in the chart is the order above
There is actually an end object for each line, which the user can get or set:
private PPTInnerTextEnd end
It indicates the end of the line information, we strongly discourage users to set the relevant parameters, the system will automatically add the end of the line object.
If you do not fully understand the meaning of the parameters, please do not manually modify, if you think that the current function can not be achieved recommended to mention issues to solve!
For the relationship between PPTText
, PPTInnerLine
, and PPTInnerText
, please refer to the documentation of PPTText
.
You can create a PPTInnerText
object, without any difference between the two ways, by:
PPTInnerText pptInnerText = new PPTInnerText();
PPTInnerText pptInnerText = PPTInnerText.build();
You can also create objects directly and set text messages at the same time:
PPTInnerText pptInnerText = new PPTInnerText(String text);
PPTInnerText pptInnerText = PPTInnerText.build(String text);
You can also set the style sheet again at the same time:
PPTInnerText pptInnerText = new PPTInnerText(String text, PPTInnerTextCss pptInnerTextCss);
PPPTInnerText pptInnerText = PPTInnerText.build(String text, PPTInnerTextCss pptInnerTextCss);
You can set or read the content of the text with the text property, which supports the Get and Set methods
@Setter
@Getter
private String text = null;
You can set the style information by using the css
property, next we will explain the PPTInnerTextCss
property and its meaning in detail.
@Setter
@Getter
private PPTInnerTextCss css = new PPTInnerTextCss();
PPTInnerText
Corresponding stylesheet object
private int fontSize = 18;
Font size, default is 18
and WPS, Ms Office inside the font size is the same
private double spacing = 0;
Character spacing, default is 0, which means default
Changing to negative value makes the text more compact, changing to positive value makes the text more loose
private String fontFamily = "宋体";
Font, if the computer does not have this font even if the settings can not be displayed properly
It is recommended to read what fonts are in the system before use
In addition, some fonts are packaged by multiple individuals or organizations, which may lead to a set of fonts with different names (e.g. Siyuan series fonts), please pay attention to similar situations!
private String color = "000000";
Text color, hexadecimal color code, without # sign. Default is black
private boolean italic;
If or not the text is tilted, the default is not tilted. Tilted display when true.
private boolean bold;
If or not the text is bolded, the default is not bolded, when it is true, it is bolded.
Cutting objects represent the cutting of elements to form a specified shape
You can create a Cutting object in the following two ways, which do not differ in any way.
Cutting cutting = Cutting.build();
Cutting cutting = new Cutting();
private double left;
Cut off the percentage of the left part 0-100
private double top;
Percentage of top side part cut off 0-100
private double right;
Cut off the percentage of the right part 0-100
private double bottom;
Percentage of bottom side part cut off 0-100
You can set the shape of the cut with the cutShape
property
The default is a right-angle rectangle
private CutShape cutShape = new RectCutShape();
There are the following classes that implement CutShape:
private double fillet;
Rounded corner ratio, the minimum value is 0 maximum value is 50
Because a called bend 50% length up, two corners add a piece has been 100%
Ellipse CutShape does not support custom parameters
RectCutShape does not support custom parameters
Border object represents a border, it is an abstract class, when using it, please use the following color border or gradient border class
Single color border object, you can create a ColorBorder object by new method
private String color = "000000";
Set color, 16-bit color information, no # included, default is black
private double width = 1.0;
Set the width in pounds, the default is 1.0
Scenes with a fixed border width and gradient color
private double width = 1.0;
Set the width in pounds, the default is 1.0
private double gradientDirection = 90;
Set the angle, the default is 90 degrees, the angle effect please refer to the gradient angle and color effect in WPS or Microsoft Office
private List<Gradient> gradients = Lists.newArrayList();
Store the gradient color object, please refer to [Reference Document] - [General Style] - [Gradient gradient] to see how to set this object
Shadow object represents the shadow, is a new version of the ppt only a function
private String color = "000000";
Set color, 16-bit color information, does not contain #, default is black
private double alpha = 0;
Set transparency, the larger the value the more transparent, the default is 0, opaque
private double sizeX = 100;
Horizontal size of the shadow, as a percentage, the same size as the original element by default
private double sizeY = 100;
Vertical size of the shadow, in percentage form, default is the same as the original element size
private double distance = 5;
Set the distance in pounds, the default is 5 pounds
private double blur = 10;
The degree of edge blur in pounds, default is 10 pounds
private double angle = 0;
Shadow cast angle, default is 0
Background is an abstract class that represents the background of an element, use its implementation class when using
Represents a single color background of a certain color
private String color;
16 prohibited color values without # sign, no default value
private Double alpha;
Support values 0 - 100, the larger the number the more transparent, the default is not transparent
Indicates the use of an image as a background
private String img;
Image path, supports png, jpg, gif
The image will be forced to stretch, it is recommended to crop in advance according to the element size
Indicates the background of the element in the form of multiple color gradients
private Double gradientDirection;
Support 0-360, default angle is 0
private List<Gradient> gradients = Lists.newArrayList();
Store the gradient color object, please refer to [Reference Document] - [General Style] - [Gradient gradient] to see how to set this object
The Gradient object represents a gradient node in the gradient process, and the system will complete the intermediate color transition according to the properties of the two adjacent gradient nodes to form a gradient effect
private String color;
Color information, hexadecimal color value information, without # sign
private double proportion;
Percentage of position, the range of values: 0-100
private Double alpha;
Transparency, value range: 0-100, the larger the number the more transparent
private Double lum;
Brightness, 0-100, the larger the number the darker
InAnimation represents the incoming animation, also known as the emergent animation.
All subclasses of AnimationElement
have incoming and outgoing animation properties.
private InAnimation inAnimation;
You can modify or read this content with the Set or Get methods
The default value is null, i.e. there is no animation.
The following properties are included in InAnimation:
private ShowAnimationType showAnimationType = ShowAnimationType.SAME_TIME;
This property sets the time of appearance of the animation, and the following three enumeration values are allowed:
-
ShowAnimationType.SAME_TIME
Simultaneous appearance (default) -
ShowAnimationType.CLICK
Click to appear -
ShowAnimationType.AFTER
Appears after the completion of the previous animation
private InAnimationType inAnimationType;
The default is null
If you don't want animation please don't set it to null here, but directly set inAnimation
to null as mentioned at the beginning of the document
property is an enumeration class that currently supports the following effects:
InAnimationType.BLINDS,//Blinds
InAnimationType.WIPE,//Erase
InAnimationType.WHEEL,//Wheels
InAnimationType.SPLITTING,//Splitting
InAnimationType.BOARD,//Checkerboard
InAnimationType.LINE,//Random Lines
InAnimationType.ZOOM//Scaling
InAnimationType
Chinese names and default durations are included in the enumeration classes for your reference.
Styles that are not in the above list are not recommended
private AnimationAttribute animationAttribute;
Properties, which are enumerated classes, are allowed to appear in some animation effects, you can set them as you like
But please make sure that the properties you set are allowed to be set in this action, if you set a property that does not belong to this action, it will automatically revert to default.
The relationship between animation effects and properties can be found in the animation settings window of WPS or Office.
private Integer timeMs;
The duration in milliseconds. Each animation contains a default duration. The default duration is the same as the default duration of this action in Office, and is generally not required to be filled in if you want to be consistent with Office.
In Office or WPS readers, the following milliseconds will be displayed as the specified semantic text. We recommend that you choose from the following values:
Duration | Label |
---|---|
500 | Very fast |
1000 | Fast |
2000 | Medium |
3000 | Slow |
5000 | Very Slow |
OutAnimation represents the incoming animation, also known as the emergent animation.
All subclasses of AnimationElement
have incoming and outgoing animation properties.
private OutAnimation outAnimation;
You can modify or read this content with the Set or Get methods
The default value is null, i.e. there is no animation.
Setting appearance animation is not supported for now, it is expected to be supported in subsequent versions!
static synchronized void PPT2MP4(String pptPath, String mp4Path)
pptPath: path to the PPT file
mp4Path: path of the mp4 file to be generated
There is currently a problem that two PPTs can't be supported by the video vbs script at the same time, so the new version forces a lock, which is currently to be solved.
Attention:
- the system must have Microsoft Office installed and activated
- Windows system only
- the generation process may have Office software anomalies resulting in the possibility of failure to convert
static void mp4Compression(String bigMp4Path, String smallMp4Path)
bigMp4Path: MP4 file generated by PPT2MP4 method
smallMp4Path: the storage path of the reduced file, ending with .mp4, does not support overwriting, this path must not exist files
Compression generated MP4 file, automatically compressed to 1280x720 size 15 frames of video, generally 25 pages of PPT video compressed in about 20MB
Notes:
1. the computer must be installed ffmeng, and add the environment variables
2. do not support adjusting parameters
static void PPT2PNG(String pptPath, String pngPath)
pptPath: PPT file path
pngPath: path to generate image storage
Calling this method will generate a folder with one image for each PPT in it
static void png2LongImg(String pngPath, String longImgPath, String waterImgPath)
pngPath: the path of the picture folder generated by the PPT2PNG method, can't contain other picture files not generated by this method, otherwise it will be wrong
longImgPath: the storage address of the long image, ending with .jpg
waterImgPath: watermark file, will be randomly attached to the long image, temporarily does not support the generation of no watermark
Generate the following effect images:
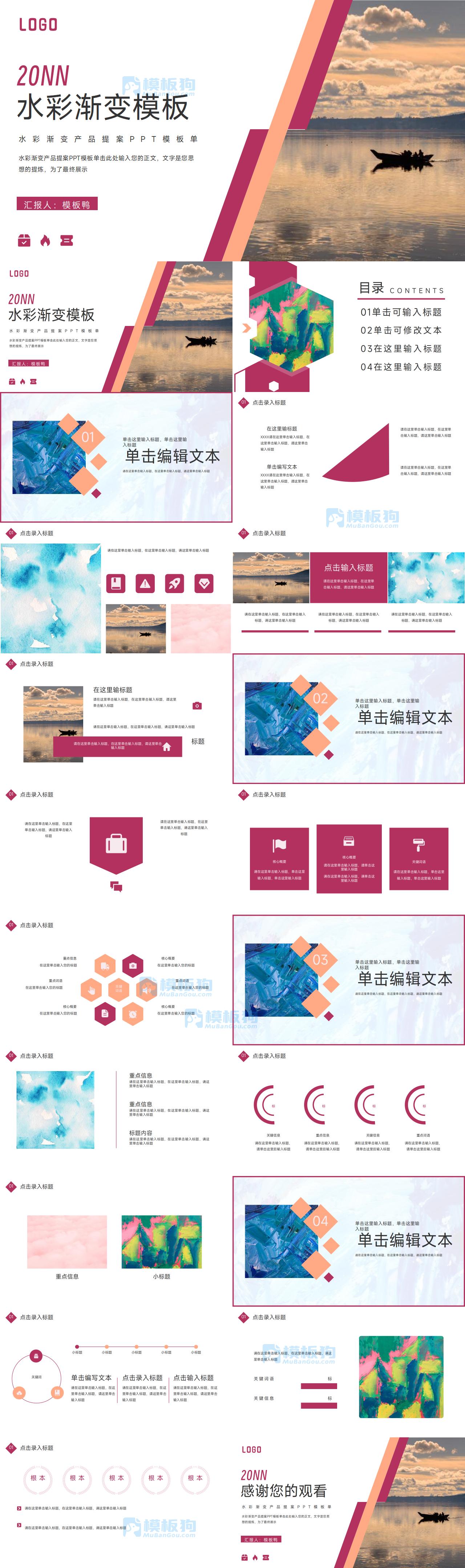
If the total number of pages is even, the first page of the small picture will appear; if the total number of pages is odd, the first page of the small picture will not appear.
static boolean isWindows()
Since several methods only support Windows computers, it is recommended that you make a determination before calling them.
If a non-Windows computer calls the above methods that are only available for Windows computers, an exception will be thrown directly.
Project Name: PPTShow
Git code address: pptshow on Github (recommended) pptshow at Gitee (Helpful to remember to point Star Oh ~)
Project website: PPTShow official website
Submit issues: Commit on Github (recommended) Commit at Gitee
View the usage agreement at Apache License 2.0
Developed by: beihem
Contact me at qiruipeng@88.com