-
Notifications
You must be signed in to change notification settings - Fork 0
Technology
Picking the right technology can often be a daunting task, especially for new developers. Head over to reddit or stack overflow and you'll find thousands of people chiming in on which technologies to use. Maybe it's the Blub Paradox coming to realization, or maybe certain tools really are best suited for certain applications. Either way, here is what I chose!
📍 Core
Initially, I developed this app in JavaScript, and it worked okay. The biggest drawback was that I was manipulating a lot of data and needed type safety. If I tried to refactor one piece of the code, another would break. So, I learned TypeScript. It was a beautiful blend of my time in C++ and Java and my new found interest in JS. It allowed me to ensure the data I was sending for conversion was correct, my props were safe, and I could sleep at night...
I also initially developed this app without auto-formatters to get a better grasp of the language and prevent any form of dependency on formatters / auto-complete. HOWEVER, I do understand the important of consistency. This is where Prettier and ESLint came into play (more on that below)
📍 Library
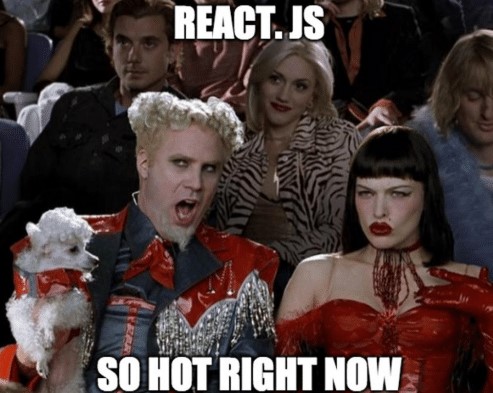
Prior to starting this project, I had written a few small apps using React. The syntax felt nice, I liked building components, and I knew there was a large community out there if I ever felt stuck. After all, it's no secret that React's popularity has skyrocketed.
While it's popularity certainly sparked my interest, I didn't rule out the possibility of frameworks like Vue. In fact, I found Vue to be quite nice. It offered similar features like the virtual DOM, JSX, and great runtime performance. Now, I won't bore you and compare React and Vue in terms of data-binding, dom manipulation, etc... I'll just say I liked React's syntax a heck of a lot more.
I can't offer a concrete "aha" moment that led me to React. Rather, a combination of wanting a developer experience that was comfortable and utilizing tools that are relevant.
📍 Build
Untill now, the few React projects I've developed used CRA (not before attepting to configure a React app from scratch). It felt slow, verbose, and unreliable at times.
After testing Vite in my last project (will link soon), it became apparant that this was what I was looking for. Not only is the set-up process extremely quick, but the development process is so smooth. The way is utilizes ES modules, the hot module replacement, and the lack of useless boilerplate code was enough to convince me. Sure, I'm no tenured developer, but I can appreciate Vite for what it is and how it helps me.
📍 Test
Naturally, as I'm using Vite, I couldn't help but take notice of Vitest. Much like Vite offered me quick bundling, Vitest offered me a quick testing workflow.
Plus, its compatable with much of the Jest ecosystem.
If I'm using Vitest, why did I list Jest? Well, I'm using jest-dom. This way, I can extend Jest's matchers, making the work flow quite interesting. I haven't taken a deep dive into the tests yet, but I wanted to be prepared for when I do. In fact, by the time you read this, there will most likely be quite a few more tests- some of which utilizing the jest-dom.
describe("r3mot", () => {
it("should probably spend less time working on this readme", () => {
expect(isProcrastinating()).toBe(true);
});
});
I won't bore you with formatting. I just wanted my code to have a visible consistency. Not only for myself (you know what I mean), but for others that will potentially see this repo. I'm open to suggestions, opionated as they may be, while I find my own style.
As for linting, it helps keep my code clean and error free. ESLint + TypeScript has been a blessing so far.
📍 State Management
State in React is... interesting. I can't recall the number of times I has to console.log("rendering");
Aside from renders, I found myself prop drilling my state with little success.
That's when I found Redux . Not only am I learning a million things at once, I decided to throw in state management library... Like I said earlier, I initially wrote this app in JS. Create stores in JS was fairly painless. Once I migrated to TS, I found myself reluctant. It was getting quite verbose and the syntax was dampening readability.
So, I said my goodbyes. I will keep the original Redux stores in the codebase for now, in the event I decide to switch back or as a reference.
After spending a day learning how to use Redux, I decided to go with hooks. Good ol' hooks! I set up some context, set up my state, and immediately felt like I had more control over my code. Maybe I was doing Redux wrong, or maybe I just like hooks. It's hard to tell. Either way, I'm currently really liking useContext combined with useEffect. For now, this works.