-
Notifications
You must be signed in to change notification settings - Fork 258
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
docs(examples): show layout constraints (#393)
Shows the way that layout constraints interact visually 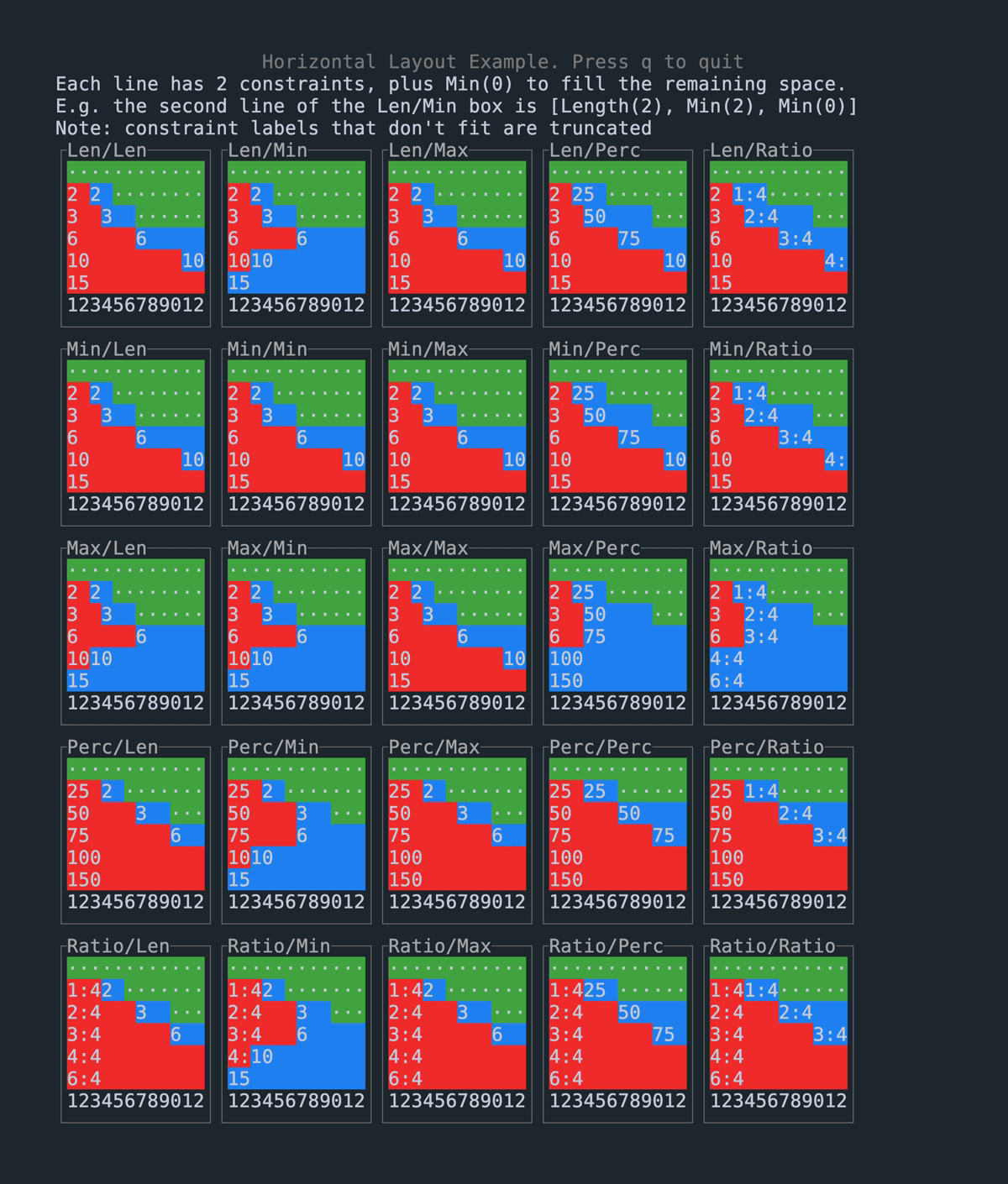
- Loading branch information
Showing
3 changed files
with
173 additions
and
45 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,11 +1,12 @@ | ||
# This is a vhs script. See https://github.com/charmbracelet/vhs for more info. | ||
# To run this script, install vhs and run `vhs ./examples/layout.tape` | ||
Output "target/layout.gif" | ||
Set Theme "OceanicMaterial" | ||
Set Width 1200 | ||
Set Height 600 | ||
Set Height 1410 | ||
Hide | ||
Type "cargo run --example=layout --features=crossterm" | ||
Enter | ||
Sleep 1s | ||
Show | ||
Sleep 5s | ||
Sleep 2s |