// file: check.js
const axios = require("axios");
const otp = "111111";
axios
.post(`https://dev-zzzzzzzz.auth0.com/oauth/token`, {
otp,
grant_type: "http://auth0.com/oauth/grant-type/passwordless/otp",
client_id: "zzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzz",
client_secret:
"zzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzz",
audience: "https://dev-zzzzzzzz.auth0.com/userinfo",
username: "zzzzzzzzzzzzzzzzzzzz@yopmail.com",
realm: "email",
scope: "openid",
})
.then((res) => {
console.log("resp::", res.data);
});
// -------------
// file: send.js
const axios = require("axios");
const userEmail = "zzzzzzzzzzzzzzzzzzzz@yopmail.com";
const AuthData = {
client_id: "zzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzz",
client_secret:
"zzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzz",
connection: "email",
email: userEmail,
send: "code",
};
axios
.post(`https://dev-zzzzzzzz.auth0.com/passwordless/start`, AuthData)
.then((res) => {
console.log("data received: ", res.data);
});
// -------------
// React Component
import auth0 from "auth0-js";
let l = console.log;
let domain = "dev-zzzzzzzz.us.auth0.com";
let clientID = "zzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzz";
let redirectUri = "http://localhost:3001";
// let clientsecret = aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa
var webAuth = new auth0.WebAuth({
clientID,
domain,
redirectUri,
responseType: "token id_token",
});
const email = "zzzzzzzzzzzzzzzzz@yopmail.com";
const fun = () => {
webAuth.passwordlessStart(
// ^ This send the magic link.
{
connection: "email",
send: "code",
// send: "link",
email,
},
function (err, res) {
// handle errors or continue
}
);
setTimeout(() => {
webAuth.passwordlessLogin(
{
connection: "email",
email,
verificationCode: String(prompt("Enter otp")),
},
function (err, res) {
// handle errors or continue
l({ err, res });
}
);
}, 8000);
};
export default function App() {
return (
<div className="App">
<h1>Hello CodeSandbox</h1>
<button
onClick={() => {
fun();
}}
>
Send magic link
</button>
</div>
);
}
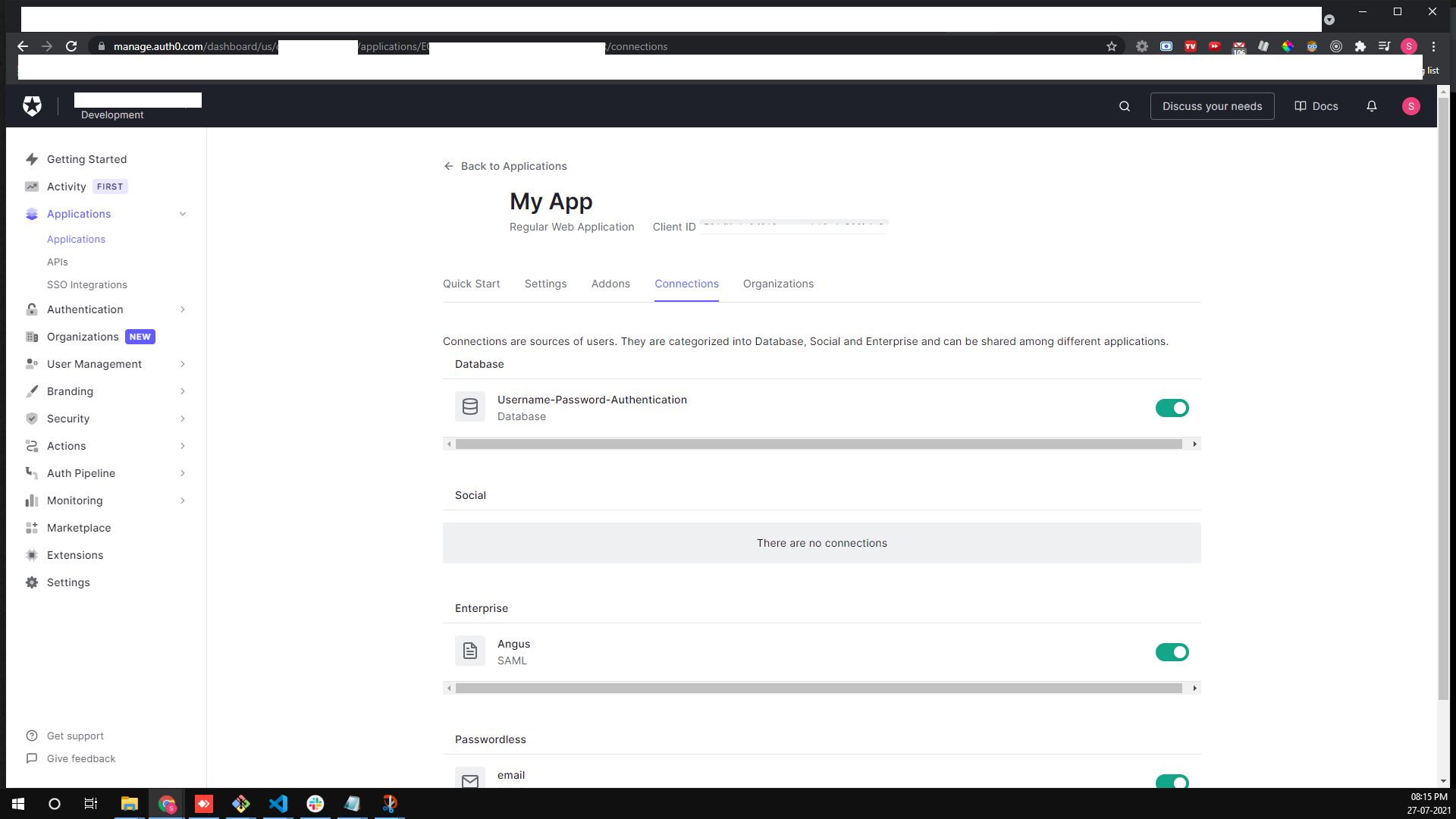
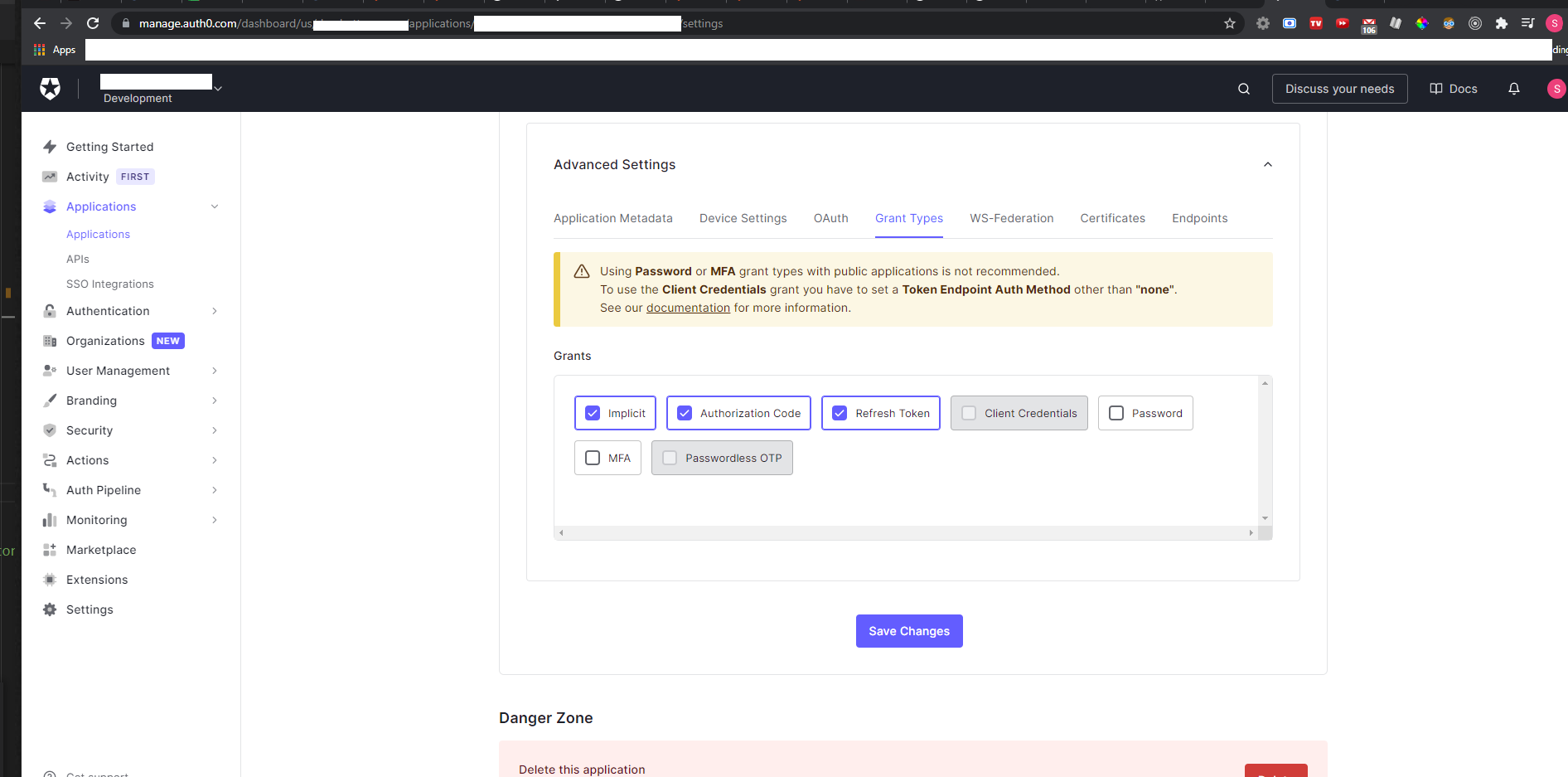
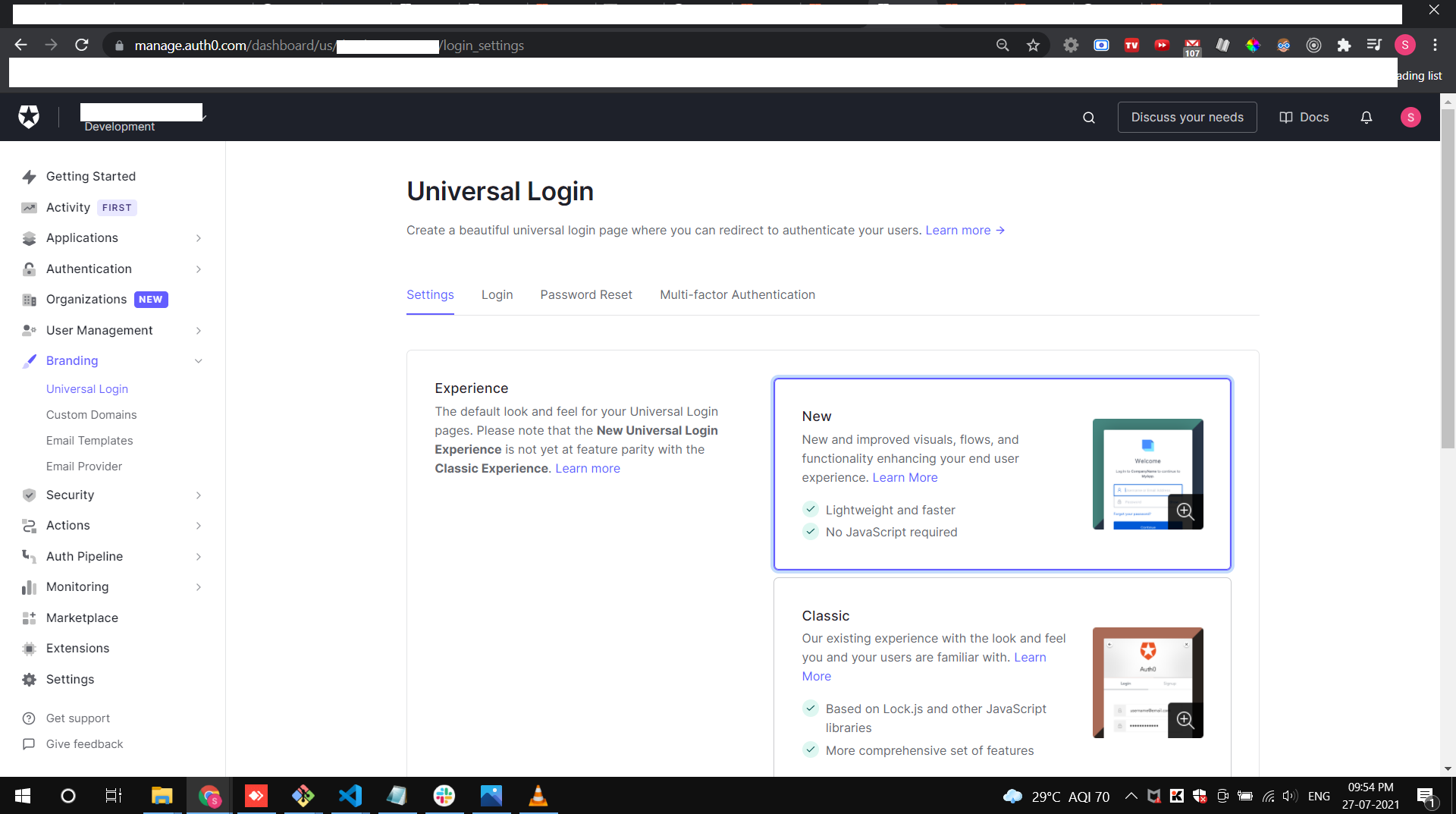
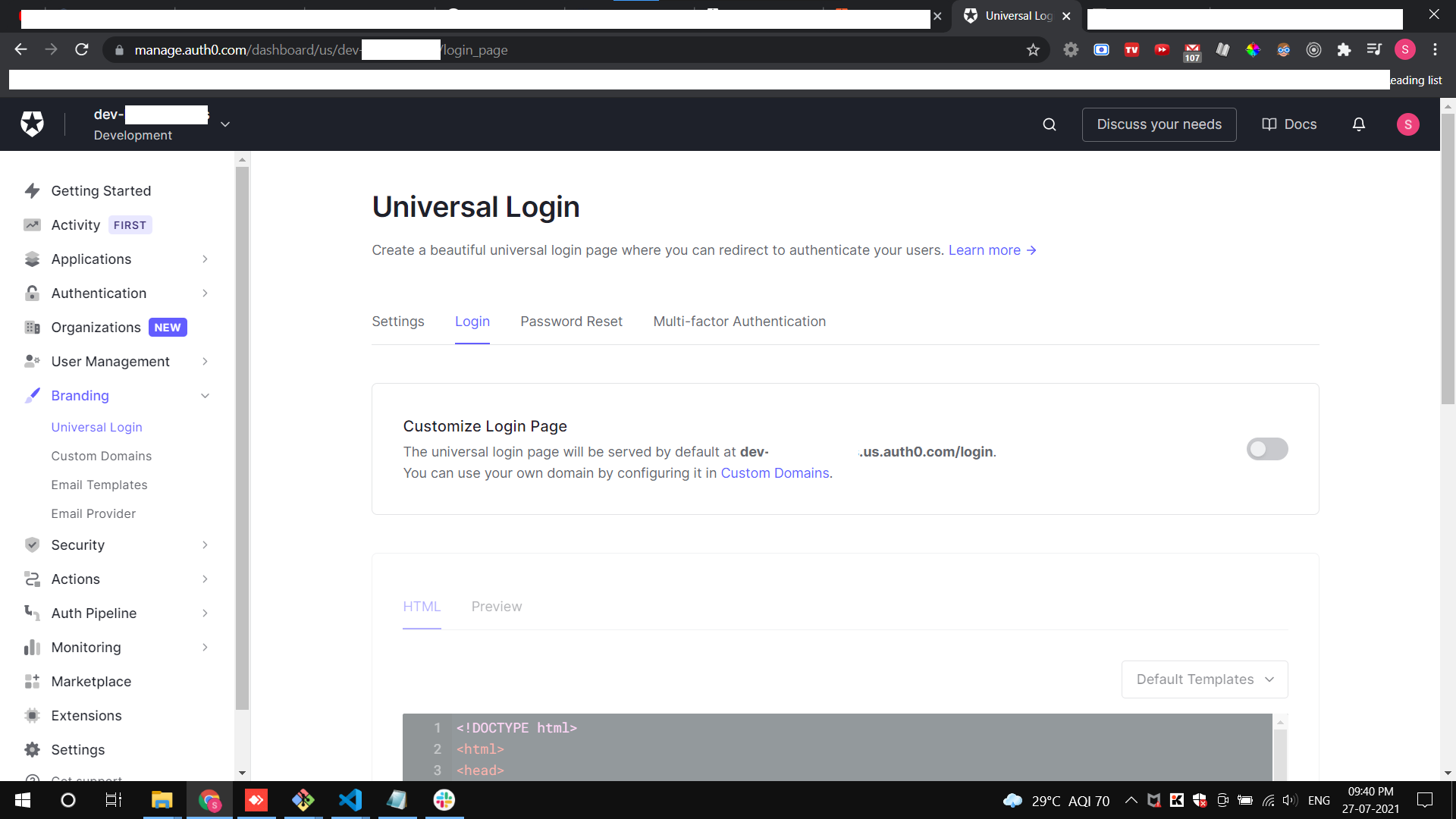
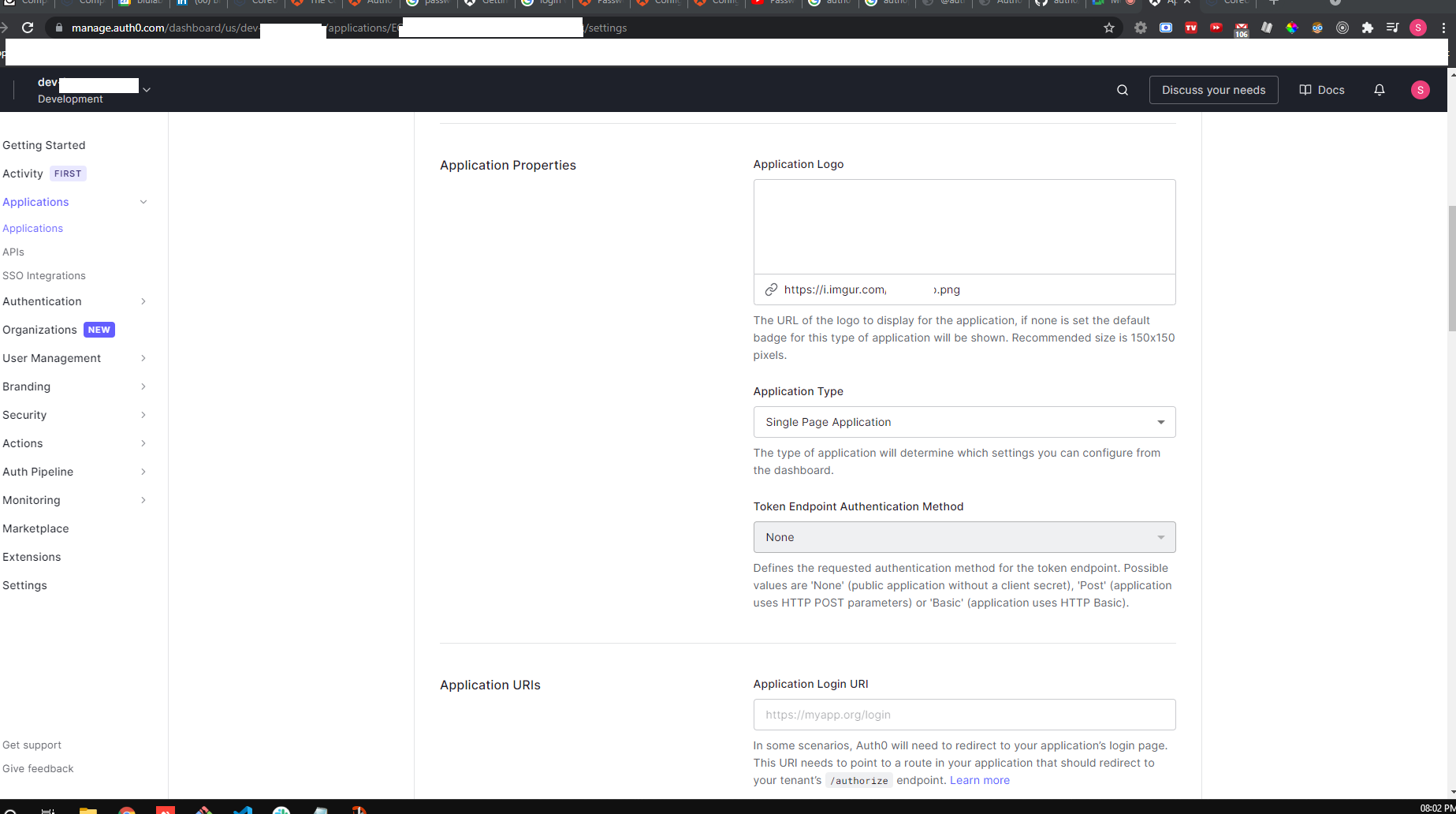