Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
[Web UI] Introduce toast notification system (#2026)
## What is this change? ### Replace react-motion with react-spring React-spring is directly inspired by the API of react-motion but has much better performance Upgrading to latest version of react due to a bug in 16.3.x see: - pmndrs/react-spring#136 - pmndrs/react-spring#215 - https://reactjs.org/blog/2018/05/23/react-v-16-4.html#bugfix-for-getderivedstatefromprops ### Create "relocation" system This PR introduces "relocation" as more flexible alternative to `React.Portal` supporting both declarative (`Relocation.Sink`) and imperative (`Relocation.Consumer`) interfaces. The intention is to eventually rely on the published `relocation` package once it is updated to match the pattern used here. Showing a toast notification: ```jsx const SomeComponent = props => ( <ToastProvider> {({ addToast }) => ( <button onClick={someAsyncAction.then( result => addToast(({ id, remove }) => ( <Toast variant="success" message={`Action succeeded with result: ${result}`} onClose={remove} maxAge={5000} // 5 seconds /> )), error => addToast(({ id, remove }) => ( <Toast variant="error" message={`Action failed with error: ${error}`} onClose={remove} maxAge={5000} // 5 seconds /> )), )} > Fire Async Action </button> )} </ToastProvider> ); ``` ### Add feedback toast for check execution Displays a toast indicating pending and success states for each requested check execution. 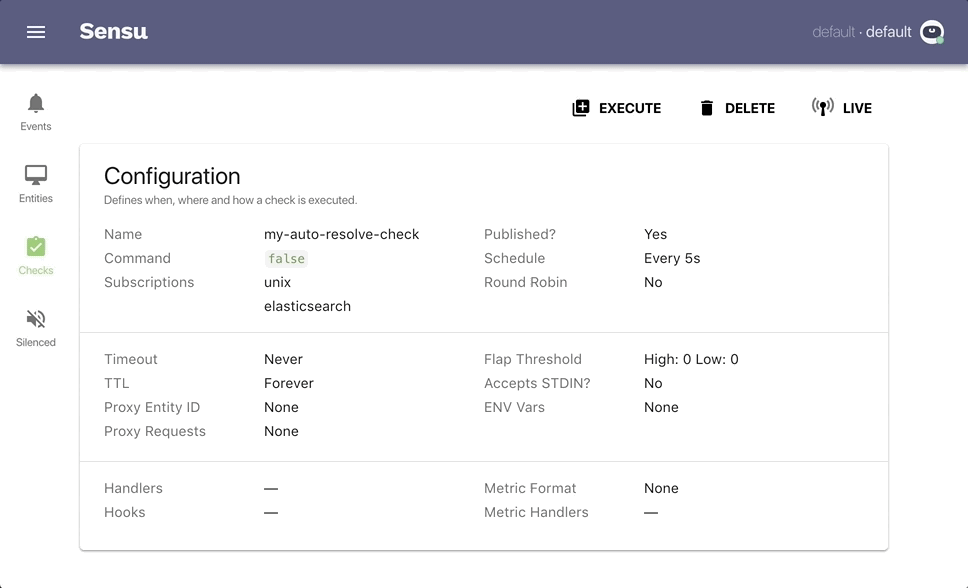 ## Why is this change necessary? Closes #1932 Closes #2012 ## Does your change need a Changelog entry? No ## Do you need clarification on anything? No ## Were there any complications while making this change? No ## Have you reviewed and updated the documentation for this change? Is new documentation required? No
- Loading branch information
Showing
32 changed files
with
1,200 additions
and
238 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,66 @@ | ||
import React from "react"; | ||
import PropTypes from "prop-types"; | ||
import ResizeObserver from "react-resize-observer"; | ||
|
||
class CircularProgress extends React.PureComponent { | ||
static propTypes = { | ||
width: PropTypes.number, | ||
value: PropTypes.number, | ||
children: PropTypes.node, | ||
opacity: PropTypes.number, | ||
}; | ||
|
||
static defaultProps = { | ||
width: 8, | ||
value: 0, | ||
children: undefined, | ||
opacity: 1, | ||
}; | ||
|
||
state = { | ||
size: 0, | ||
}; | ||
|
||
handleResize = rect => { | ||
this.setState(state => { | ||
const size = Math.min(rect.width, rect.height); | ||
if (size === state.size) { | ||
return null; | ||
} | ||
return { size }; | ||
}); | ||
}; | ||
|
||
render() { | ||
const { size } = this.state; | ||
const { width, value, children, opacity } = this.props; | ||
|
||
return ( | ||
<div style={{ position: "relative" }}> | ||
<ResizeObserver onResize={this.handleResize} /> | ||
<svg | ||
viewBox={`0 0 ${size} ${size}`} | ||
style={{ display: "block", position: "absolute" }} | ||
> | ||
{size > 0 && ( | ||
<circle | ||
transform={`rotate(-90, ${size * 0.5}, ${size * 0.5})`} | ||
cx={size * 0.5} | ||
cy={size * 0.5} | ||
r={(size - width) / 2} | ||
strokeDasharray={Math.PI * (size - width)} | ||
strokeDashoffset={Math.PI * (size - width) * (1 - value)} | ||
fill="none" | ||
stroke="currentColor" | ||
opacity={opacity} | ||
strokeWidth={width} | ||
/> | ||
)} | ||
</svg> | ||
{children} | ||
</div> | ||
); | ||
} | ||
} | ||
|
||
export default CircularProgress; |
Oops, something went wrong.