New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Slowdown detection with many lines #975
Comments
An easy answer here is that we really don't recommend outputting that many rows with one BUT, this is a performance issue, so I did a quick profile. Looks like the issue is that on I would suspect a much simpler TableRow comparer that checked if the object reference equals would improve performance across the board and be potentially worth adding. |
Thought some more about this. Might be a bit hacky, but removing
|
Yes, I confirm the removal of
Are you doing a pull request with this? |
When printing tens of thousands of lines, there is a performance issue. The TableRowCollection -> Add method was being called millions of times. Once it is called, it returns the index of the added element. As the element is added to a List (new List<TableRow>, I believe it is not needed to use IndexOf because it will be added always at the last position. Replacing the IndexOf call by a Count - 1 improves the performance in this scenario. Related issue: spectreconsole#975
Information
Describe the bug
Detected that Spectre.Console is faster than Console.Writeline from 100 lines until 20K lines. Then, it's performance drops until taking twice the time to print 50K lines or even 10 times slower with 100K lines.
To Reproduce
I prepared a small program to do the testing, for your consideration:
Expected behavior
We have detected when printing 100K lines withing tables of 3 columns, that using Sprectre.Console takes up to 103 seconds in average while we are printing with Console.Writeline the same amouint of lines in 11 seconds. I'm not saying that it should take the same, just the difference seems too big and keeps on growing with the number of files.
Screenshots
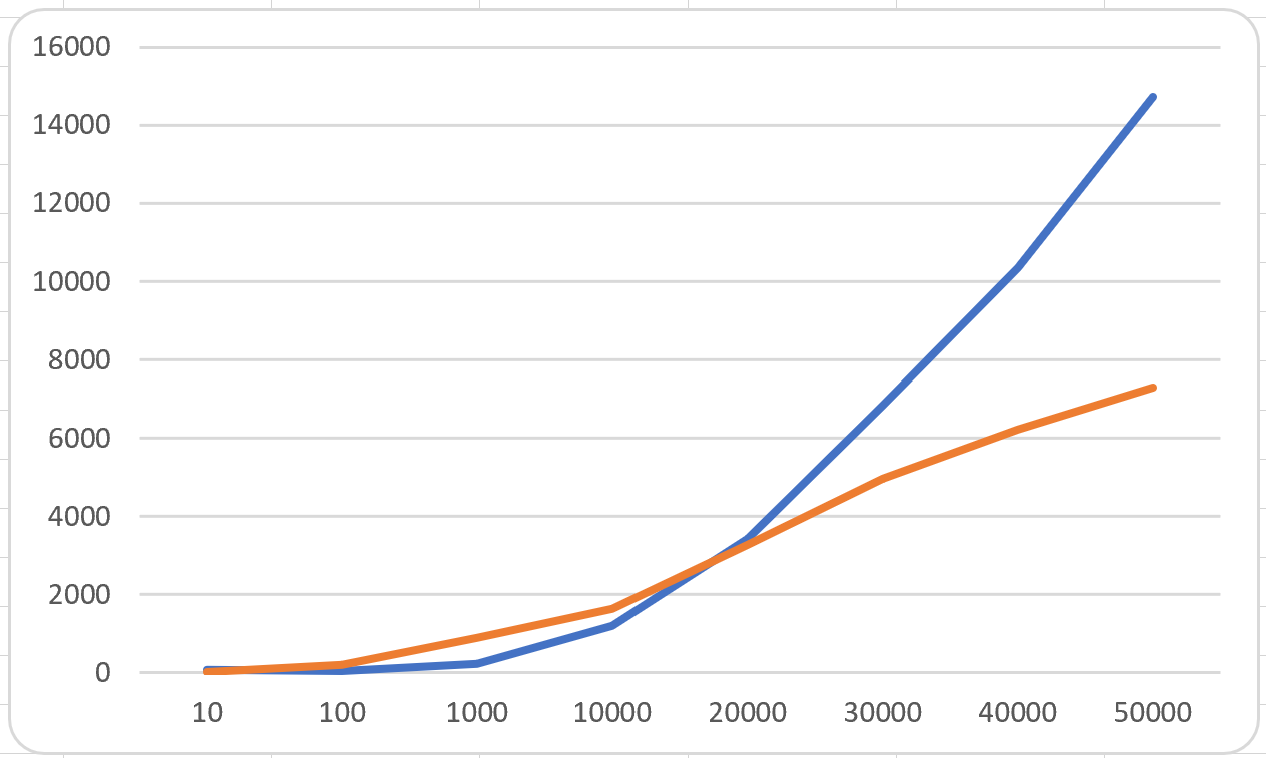
Additional context
I'm not sure whether the preprocessing is lagging the overall times, maybe it is just a compromise solution, but JIC the might be a memory issue somewhere. We are working with Version Control and it is not rare that, when creating a new repo, there might be hundreds of thousands of new files to upload. If we do an status with that amount of private files, it gets frozen for almost 2 minutes.
Please upvote 👍 this issue if you are interested in it.
The text was updated successfully, but these errors were encountered: