-
Notifications
You must be signed in to change notification settings - Fork 4
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
"clear" color leak on edges when using BlitIntoBitmap #5
Comments
Actually, the "red" line is larger than 1 px, and the offsets on BlitIntoBitmap are simply masking it off. This is deeper something's not putting color on those edges. |
@kf6kjg |
My research indicates that after the return of the FS call in |
@kf6kjg This fix might result in your attributes being extrapolated so its critical that you clamp them. I have released a v0.3.3b version with this issue fixed.As for the the bar on the top and the bottom il try to work out a fix soon, once I'm able to reproduce the problem. |
Just gave it a run using the code at commit 1dca3eb. I still have the red.. :/ |
@kf6kjg |
@kf6kjg |
The mesh is square and occupies the range 0-256 units in both axes. In this range there are two tris for every unit, due to filling the quad defined by the square between the pixels of the heightmap that is 256x256 pixel: each pixel is converted to a vertex. In fact here's an STL of the one I've been testing, exported right out of my vertex buffer: My testing VS and FS are pretty simple: public unsafe void ScaleCorrectionVS(float* OUT, float* IN, int Index)
{
OUT[0] = IN[0];
OUT[1] = IN[1];
OUT[2] = IN[2];
}
public unsafe void TerrainShaderFS(byte* bgr, float* attributes, int faceIndex)
{
bgr[0] = 255;
bgr[1] = 255;
bgr[2] = 255;
if (faceIndex < 50)
{
bgr[0] = 0;
bgr[1] = 255;
bgr[2] = 0;
}
} The value var GL = new renderX(_pixelScale, _pixelScale); // 512, 512
//...lots of code...
GL.BlitIntoBitmap(mapbmp.Bitmap, new Point(), new Rectangle(0, 0, mapbmp.Width, mapbmp.Height)); // 512,512 |
lol, the above review points out to me that I'm putting too many vertices into my mesh: it's 512x512 even though it only has 256x256 data in the base terrain heightmap. EDIT: Fixing the code to a 256x256 resolution mesh doesn't change the red line. Even dropping it to 64x64 doesn't do anything. |
So based of the information here, I have to assume the issue is related to the rasterization. The first problem relates to how renderXF translates its "Normalized" world coordinates into screen space coordinates: VERTEX_DATA[im * Stride + 0] = roundf(rw + VERTEX_DATA[im * Stride + 0] / ox);
VERTEX_DATA[im * Stride + 1] = roundf(rh + VERTEX_DATA[im * Stride + 1] / oy); In the last release The second problem relines in the Regardless I am releasing v0.3.4 which has rounding on the XYZ to XY transforms and loosened epsilons for the bigger or equal checker. Additionally I have improved the performance for tiny triangles by ~3x (at the cost of big triangles) by removing the extra parallelization loop inside for the I have tested this update with a 256x256 image and a 256 by 256 mesh and I didn't have any pixels not being filled. |
Hmmm, Heres the release v0.3.4b |
I pull straight from master. And that commit doesn't look right, you removes a couple dozen files and left an unresolved merge in the tree... :/ |
Sorry, I accidentally merged the files incorrectly, and thus I had to delete the incorrectly copied ones. I'm a mech eng student and not a software eng student so I'm not very good with github and git in general. |
I was a mech engineer for 15 years designing routers and saws for granite, and a software engineer all through and since. Hopefully I can help. I've created a Pull Request that might help keep the source code tree clean. I'll try again with the new code. |
Ok, that's very different. The red edges are now gone, but in their place is a grid of red dots: GL.BlitIntoBitmap(mapbmp.Bitmap, new Point(), new Rectangle(0, 0, mapbmp.Width, mapbmp.Height));
mapbmp.Bitmap.RotateFlip(RotateFlipType.RotateNoneFlipY); // Fix the image being upside down. So thus the first scanline is now on the bottom and the grid of dots is in the last few scanlines. [EDIT: Side note I had to use the changes that are in PR #7 to accomplish this.] |
Hmm can you try adding ...
else if (matrixlerpv == 1)
for (int im = 0; im < BUFFER_SIZE; im++)
{
VERTEX_DATA[im * Stride + 0] = roundf(rw + VERTEX_DATA[im * Stride + 0] * iox);
VERTEX_DATA[im * Stride + 1] = roundf(rh + VERTEX_DATA[im * Stride + 1] * ioy);
if (VERTEX_DATA[im * Stride + 1] > yMaxValue) yMaxValue = VERTEX_DATA[im * Stride + 1];
if (VERTEX_DATA[im * Stride + 1] < yMinValue) yMinValue = VERTEX_DATA[im * Stride + 1];
} Additionally you can try tightening the |
Hmm, I don't recall changing the attribute code, however il go check if see if I broke it. In the meanwhile can you try this small last change, as it will tell me if the scanline algorithm or the XYZ to XY transforms are at fault here: In yMax = (int)yMaxValue; Replace this with yMax = (int)yMaxValue + 1; OR, this: yMax = (int)yMaxValue == renderHeight - 2 ? renderHeight - 1 : (int)yMax; |
Alright, it seems like my only remaining choice is to reinforce the |
Okay, sorry for the delay, I got a bit carried away writing XFDraw's new shader parser/compiler, but regardless I think I've fixed the problem. Can you test the fix by replacing the unsafe bool ScanLinePLUS(int Line, float* TRIS_DATA, int TRIS_SIZE, float* Intersects)
{
int IC = 0;
for (int i = 0; i < TRIS_SIZE - 1; i++)
{
float y1 = TRIS_DATA[i * Stride + 1];
float y2 = TRIS_DATA[(i + 1) * Stride + 1];
if (y2 == y1 && Line == y2){
LIPA_PLUS(Intersects, 0, TRIS_DATA, i, i + 1, Line);
LIPA_PLUS(Intersects, 1, TRIS_DATA, i + 1, i, Line);
return true;
}
if (y2 < y1){
float t = y2;
y2 = y1;
y1 = t;
}
if (Line <= y2 && Line > y1){
LIPA_PLUS(Intersects, IC, TRIS_DATA, i, i + 1, Line);
IC++;
}
if (IC >= 2) return true;
}
if (IC < 2)
{
float y1 = TRIS_DATA[0 * Stride + 1];
float y2 = TRIS_DATA[(TRIS_SIZE - 1) * Stride + 1];
if (y2 == y1 && Line == y2){
LIPA_PLUS(Intersects, 0, TRIS_DATA, 0, (TRIS_SIZE - 1), Line);
LIPA_PLUS(Intersects, 1, TRIS_DATA, (TRIS_SIZE - 1), 0, Line);
return true;
}
if (y2 < y1){
float t = y2;
y2 = y1;
y1 = t;
}
if (Line <= y2 && Line > y1){
LIPA_PLUS(Intersects, IC, TRIS_DATA, 0, TRIS_SIZE - 1, Line);
IC++;
}
}
if (IC == 2) return true;
else return false;
} and then replacing these lines right after ...
{
FROM = Intersects;
TO = Intersects + (Stride - 1);
}
FROM[0] = roundf(FROM[0]);
TO[0] = roundf(TO[0]);
FromX = (int)FROM[0] == 0 ? 0 : (int)FROM[0] + 1;
ToX = (int)TO[0];
slopeZ = (FROM[1] - TO[1]) / (FROM[0] - TO[0]);
bZ = -slopeZ * FROM[0] + FROM[1];
if (ToX >= renderWidth) ToX = renderWidth - 1;
if (FromX < 0) FromX = 0;
float ZDIFF = 1f / FROM[1] - 1f / TO[1];
... Lastly, ensure the As for updating to newer net standards, I plan on rewriting renderXF with XFDraw's code once its done, but with the only difference being that the rendering would still be done in c#. This would allow for nice things such as being able to have multiple |
Combining all that together on top of latest master it's perfect: all red lines and dots are gone. Good news on XFDraw. I look forward to integrating it when it's ready: the way I built Anaximander2 it can have multiple renderers that are user selectable. |
I built a variant of the texturetest package that demonstrates the clear color leakage: https://github.com/kf6kjg/renderXF/tree/anax_issues_demo The above changes seem to fix it, so if those could land in the repo it'd be great. |
This might be a problem in my code, but my testing seems to be ruling that out.
Here's my camera setup:
I then make a mesh that occupies from 0,0 to 256,256 - the Z value is variable. I've validated that the
terrainVertexPoints
array that feeds the vertex buffer has that range via debugging.I then render the mesh using
You can see the 1 pixel red lines on the edges:
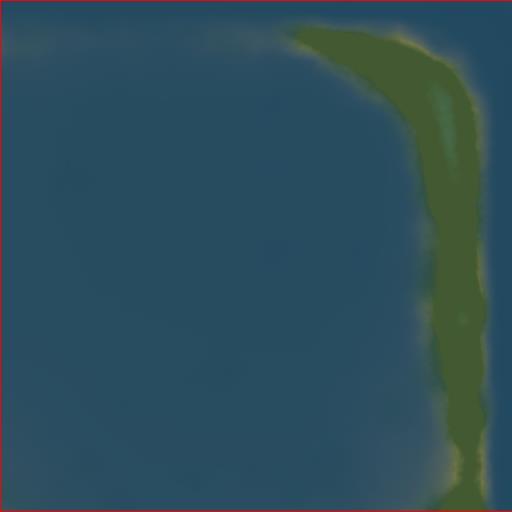
To test what was going on:
GL.SetMatrixData(FOV, 64f, 1f)
so that I was certain that the terrain fully filled the viewport, and still got the same red lines.The text was updated successfully, but these errors were encountered: