New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
FastAPI and Pydantic - Relationships Not Working #37
Comments
@Chunkford - I tried your code above, it works fine. I suspect maybe the way you are doing things isn't working. The sequence I used was:
I assume the order you did things is different? - If you set the Team ID to something that doesn't exist in the database then you will see a null response, if you then add a team that has that ID then the get will start to populate. So in your screenshots, you would want to check the teams and make sure that a team with team_id 2 actually exists in the database - my suspicion is that it doesn't. If it was a production app, I would likely add some checks to stop setting a team_id when the id doesn't exist in the team table but that would complicate the example I think. |
I too am having the exact same issue regarding backrefs not displaying data. Both with my own app, and the demo above. I followed your example too @obassett with a failed outcome. Data was created and linked (according to dbeaver), however no data is being displayed with a API call is requested. Tested Versions on 0.0.3 and 0.0.4 Edit: added screenshots |
Do you see it trying to do the SQL lookups if you set echo=True in the create engine? Or are there any errors logged when trying to access it? |
@obassett The code I posted came straight from the tutorial pages - https://sqlmodel.tiangolo.com/tutorial/fastapi/relationships/ Here's the SQL output for when I access an individual hero
|
OK, worked out what it was. @alucarddelta update fastapi |
@Chunkford I suppose, you shouldn't ever parse lazy relations in production. You would query your database (n * k + 1) times on each request, where n is amount of items in response, and k is amount of relation-fields in single model. You should either restrict relations in your DTOs (to avoid lazy loading), or specify SQLAlchemy's select options (selectinload, joinedload etc). |
This worked @Chunkford, I have submitted a pull request #48 to change the pip requirement for the project to be 0.68.1 as it was set to 0.68.0. |
🤦♂️ I lost so many hours on this -- thank you for the version update recommendation and MR! |
Still having the same issue, here is my case:
class Professional(Base, table=True):
permissions: ProfessionalPermissions = Field(ProfessionalPermissions.NONE, nullable=False,
sa_column_kwargs={'server_default': text('0')})
store_id: UUID = Field(foreign_key="store.id", primary_key=True)
store: List['Store'] = Relationship(back_populates="professionals") class Store(Base, table=True):
id: UUID = UUIDField(primary_key=True)
# ORM Relationships
professionals: List['Professional'] = Relationship(back_populates="store") @router.get("", response_model=List[Store])
def list_stores(
limit: int = 100,
offset: int = 0,
crud: CRUDController = Depends(get_crud),
_: User = Depends(get_current_user),
):
r = crud.store.list(limit, offset)
print(r[0].professionals)
return r What I get in the request response [
{
"created_at": "2021-12-10T15:45:05.382071",
"id": "7847365b-bae3-467e-9efa-e6b6f09c5e80",
"phone_number": "+351210000001",
"email": "store1@scedle.com",
"updated_at": "2021-12-10T15:45:05.382071",
"name": "Store 1"
},
...
] What I get in the terminal with the
|
I had the same problem but using an async engine. I solved it using selectinload as suggested here #74 response = await db_session.exec(select(self.model).where(self.model.id == id).options(selectinload('*'))) |
My issue with this was that my Pydantic models for my endpoints (response models) weren't lining up with my SQLAlchemy models. There's really good info here. |
This issue exists in 0.78.0 version for me. Anyone else facing the same in the latest version? Please help. |
Have a look at: #315 Pinning |
I had the same error using sqlmodel with SQLAlchemy 1.4.40... How to reproduce: Use sqlmodel=0.0.6 with SQLAlchemy=1.4.40 |
related to tiangolo/sqlmodel#37
With sqlmodel See tiangolo/sqlmodel#37
We are still seeing this error, very similarly to rickerp. We have a model with a one to many relationship (e.g. Team to Heroes). In our route, we can print the Team object, and it has Heroes populated. However, when we get the response from the router, it is totally missing. We are on FastAPI version 0.85.1 and SQLModel version 0.0.8. e.g.
The prints have all the data populated. But in receiving this response, the data for heroes is totally missing. Our team model looks like this:
|
It seems that at the root of the issue is the fact that in FastAPI's encoder call, it's calling |
@nick-catalist, did you have a solution to this? I am also struggling with this. Even when using |
There is no need to do subqueryload, if you properly define "out models" and configure |
@JBorrow @tonjo .. right, thank you tonjo for jogging my memory on this as well. All you need to do is define a separate response model and redefine the relationship field as a list instead. E.G. you'd want a set up like this: class TeamBase(SQLModel):
name: str = Field(sa_column=Column(String, nullable=False))
class Team(TeamBase, table=True):
__tablename__ = 'team'
id: uuid.UUID = Field(sa_column=Column(
UUID(as_uuid=True),
primary_key=True,
server_default=text("gen_random_uuid()"),
nullable=False,
unique=True,
))
heroes: list["Hero"] = Relationship(back_populates="team", sa_relationship_kwargs={
"cascade": "all, delete-orphan"
})
class TeamResponse(TeamBase):
heroes: list["Hero"] Like tonjo said, Pydantic doesn't know how to render a relationship, but it does know how to render a plain list. Additionally, it will coerce your returned value into whatever you have defined as your See more info at https://sqlmodel.tiangolo.com/tutorial/fastapi/relationships/#models-with-relationships |
Ah fantastic, thank you both for your quick reply! This makes sense, though it does feel like a bit of an antipattern ( |
Also, @nick-catalist, I presume you want |
Yes, that was a typo, fixed :) |
First Check
Commit to Help
Example Code
Description
Is realationships working for anyone?
I either get null or an empty list.
OK, so, I've copied the last full file preview at the - https://sqlmodel.tiangolo.com/tutorial/fastapi/relationships/
Run it and it creates the Db and the foreign key
Then I've insert the data into the Db.
Checking the docs UI everything looks great
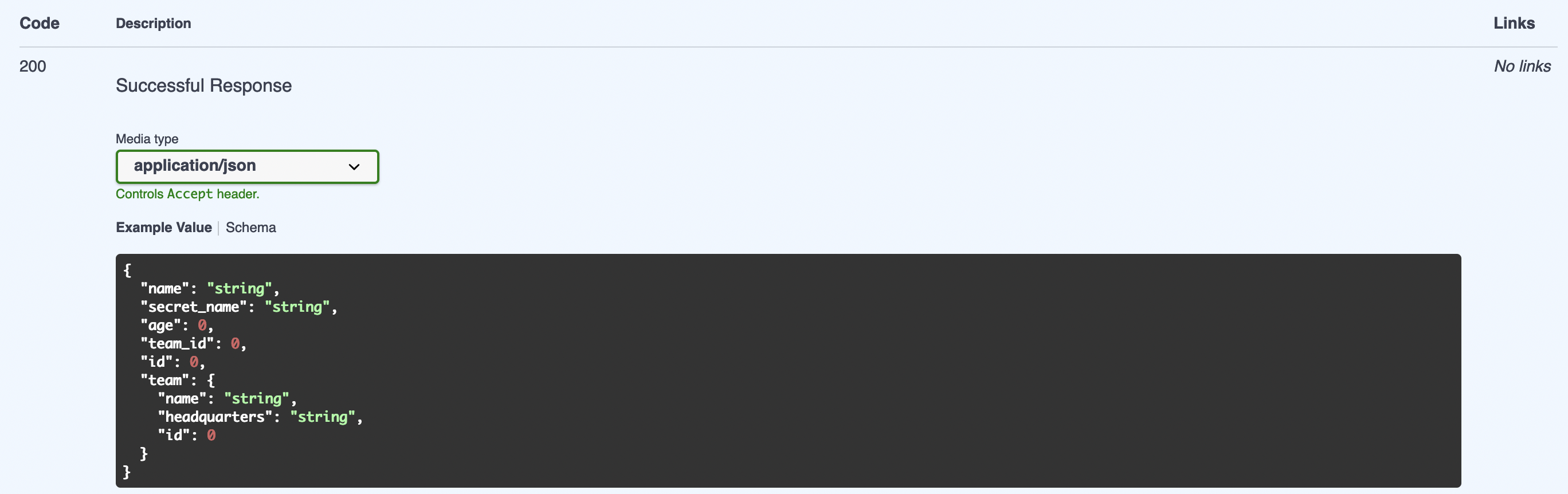
But when I do a request for a hero,
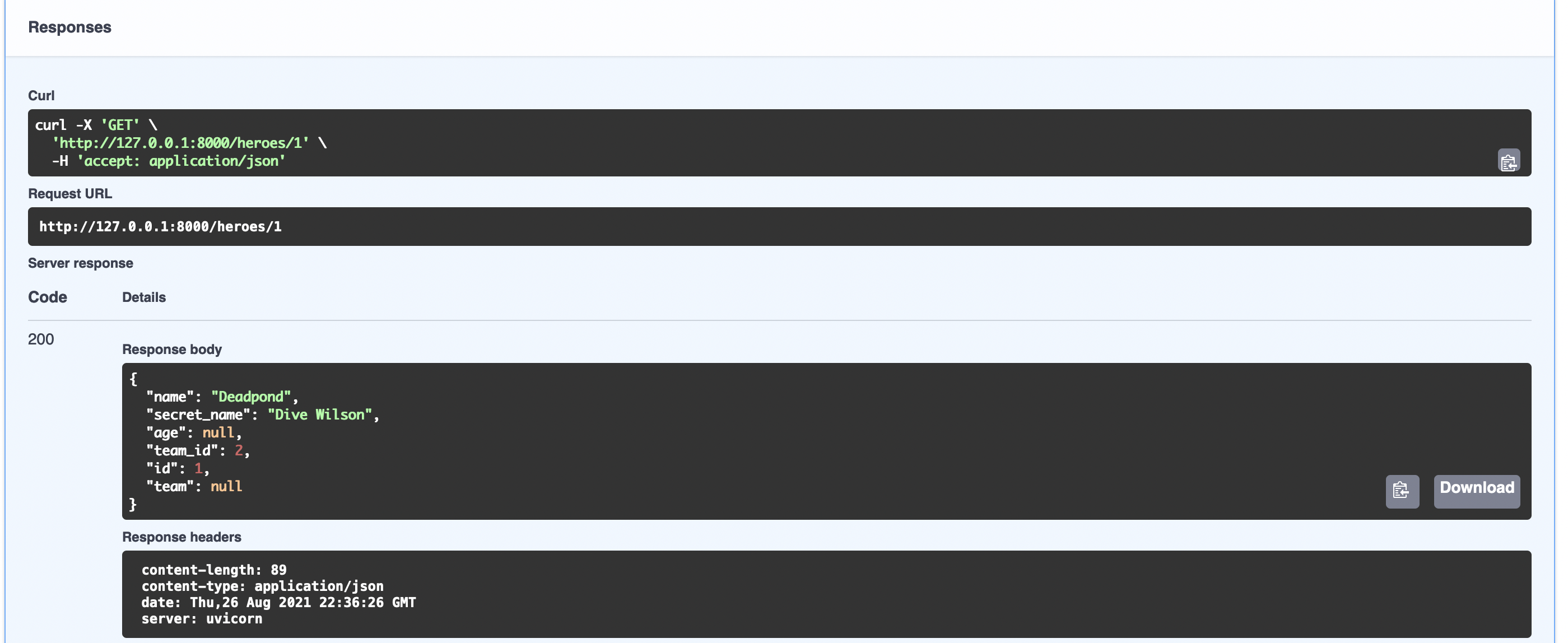
team
isnull
Really not sure what going on, especially when all I have just is copied the code example with no changes?
Operating System
Linux
Operating System Details
No response
SQLModel Version
0.0.4
Python Version
3.8.2
Additional Context
No response
The text was updated successfully, but these errors were encountered: