-
Notifications
You must be signed in to change notification settings - Fork 26.3k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Improve RSC compiler error in external module (#46953)
When the RSC compiler error was caused by an external package, make the error message display which import caused the error. Also don't show node_module files in the import trace. Continuation of #45484 Before 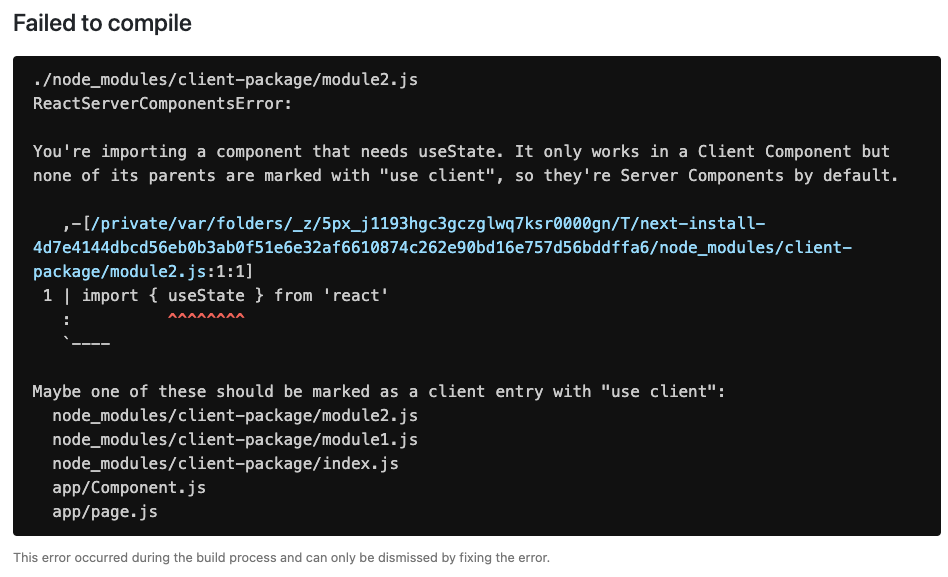 After 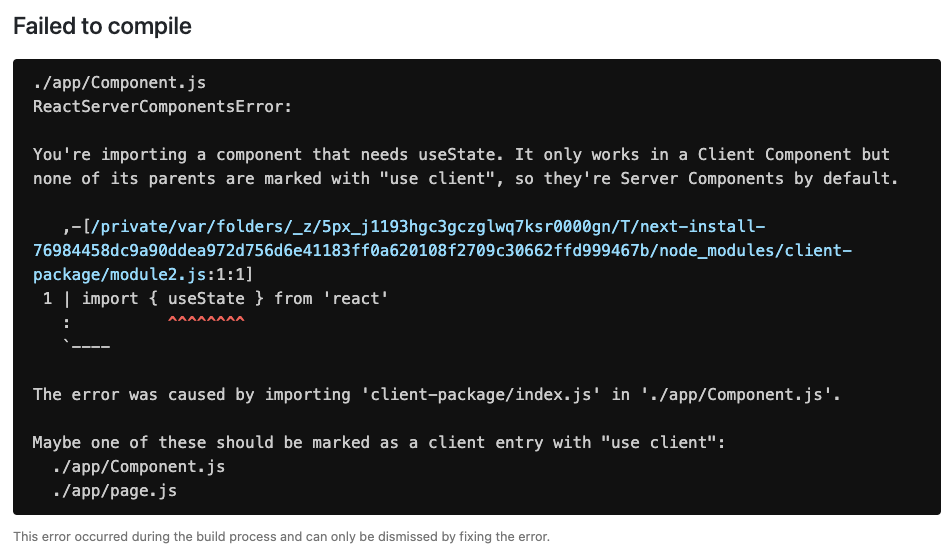 Updates the format of the files, from `app/page.js` to `./app/page.js` to align it with other import traces. 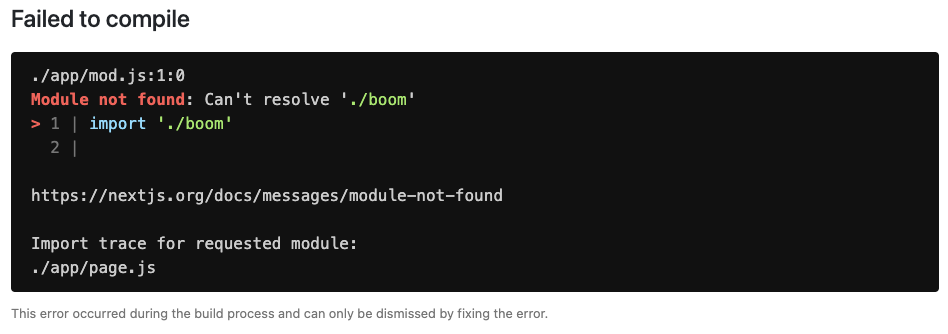 Closes NEXT-523
- Loading branch information
1 parent
09d21d6
commit 9a41ba9
Showing
6 changed files
with
137 additions
and
73 deletions.
There are no files selected for viewing
55 changes: 48 additions & 7 deletions
55
packages/next/src/build/webpack/plugins/wellknown-errors-plugin/getModuleTrace.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters