Contributing to Identity Server
- How to find codes (Repository structure)
- Best Practices in Coding
- How to configure WSO2 code style template
- Useful IntelliJ IDEA plugins
- Coding process
- Customizing WSO2 Log Files
- Connectors
- How to publish your connector in WSO2 connector store
- How to raise issues
- Code review guidelines
Working in progress .......
Topics that are going to cover
-
About - Identity server source code structure and how we maintain them in a GitHub
-
The product maintains the source code under two GitHub organizations: wso2 and wso2-extensions.
-
Describe wso2 and wso2-extensions.
-
Why we use Versions and tags
-
rEx tool Reference to rEx - https://github.com/prabath/wso2is-repo-explorer
https://wso2.github.io/github-repositories.html http://soatutorials.blogspot.com/2015/02/simple-tutorial-to-work-with-wso2-git.html
Code readability is a universal subject in the world of computer programming. This article will detail the most important best practices when writing readable code.
80% of the lifetime cost of a piece of software goes to maintenance. Hardly any software is maintained for its whole life by the original author. Good quality code improves the readability of the software, allowing engineers to understand new code more quickly and thoroughly. If you ship your source code as a product, you need to make sure it is as well packaged and clean as any other product you create.
All source files should begin with a c-style comment that lists the programmer(s), the date, a copyright notice, and also a brief description of the purpose of the program. For example:
Ex 1.
* Classname
*
* Version info
*
* Copyright notice
*/
Ex 2.
/*
* Copyright (c) 2022, WSO2 LLC. (http://www.wso2.com).
*
* WSO2 LLC. licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file except
* in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
For the components developed privately, the following copyright comment is used.
/*
* Copyright (c) 2022, WSO2 LLC. (http://www.wso2.com). All Rights Reserved.
*
* This software is the property of WSO2 Inc. and its suppliers, if any.
* Dissemination of any information or reproduction of any material contained
* herein in any form is strictly forbidden, unless permitted by WSO2 expressly.
* You may not alter or remove any copyright or other notice from copies of this content.
*/
The first non-comment line of most Java source files is a package statement. After that, import statements can follow.
For example:
import org.apache.commons.lang.StringUtils;
import org.apache.commons.logging.Log;
In the wso2 we avoid lines longer than 120 characters since they’re not handled well by many terminals and tools.
When an expression will not fit on a single line, break it according to these general principles:
• Break after a comma. • Break before an operator. • Prefer higher-level breaks to lower-level breaks. (in other words avoid breaking nested expressions)
Ex -
// prefer higher-level breaks
longName1 = longName2 * (longName3 + longName4 - longName5)
+ 4 * longname6;
• Align the new line with the beginning of the expression at the same level on the previous line. • If the above rules lead to confusing code or to code that’s squished up against the right margin, just indent 4 spaces instead.
Here are some examples of breaking method calls:
function(longExpression1, longExpression2, longExpression3,
longExpression4, longExpression5);
var = function1(longExpression1,
function2(longExpression2,
longExpression3));
Following are two examples of breaking an arithmetic expression. The first is preferred, since the break occurs outside the parenthesized expression, which is at a higher level.
longName1 = longName2 * (longName3 + longName4 - longName5)
+ 4 * longname6; // PREFER
longName1 = longName2 * (longName3 + longName4
- longName5) + 4 * longname6; // AVOID
Line wrapping for if statements should generally use the 4-space rule.
Ex -
//DON’T USE THIS INDENTATION
if ((condition1 && condition2)
|| (condition3 && condition4)
||!(condition5 && condition6)) { //BAD WRAPS
doSomethingAboutIt(); //MAKE THIS LINE EASY TO MISS
}
//USE THIS INDENTATION INSTEAD
If ((condition1 && condition2)
|| (condition3 && condition4)
||!(condition5 && condition6)) {
doSomethingAboutIt();
}
//OR USE THIS
if ((condition1 && condition2) || (condition3 && condition4)
||!(condition5 && condition6)) {
doSomethingAboutIt();
}
Here are three acceptable ways to format ternary expressions:
alpha = (aLongBooleanExpression) ? beta : gamma;
alpha = (aLongBooleanExpression) ? beta
: gamma;
alpha = (aLongBooleanExpression)
? beta
: gamma;
Java programs can have two kinds of comments
Implementation comments. - Implementation comments are mean for commenting out code or for comments about the particular implementation
Documentation comments. (known as “doc comments”) - Doc comments are meant to describe the specification of the code, from an implementation-free perspective. to be read by developers who might not necessarily have the source code at hand.
Note: The frequency of comments sometimes reflects poor quality of code. When you feel compelled to add a comment, consider rewriting the code to make it clearer.
Programs can have four styles of implementation comments:
- block.
- single-line.
- trailing and end-of-line.
Block comments are used to provide descriptions of files, methods, data structures and algorithms. Block comments should be used at the beginning of each file and before each method. They can also be used in other places, such as within methods. Block comments inside a function or method should be indented to the same level as the code they describe. A block comment should be preceded by a blank line to set it apart from the rest of the code. Block comments have an asterisk “*” at the beginning of each line except the first.
/*
* Here is a block comment.
*/
Short comments can appear on a single line indented to the level of the code that follows. If a comment can’t be written in a single line, it should follow the block comment format.A single-line comment should be preceded by a blank line. Here’s an example of a single-line comment in Java code
if (condition) {
/* Handle the condition. */
...
}
Very short comments can appear on the same line as the code they describe, but should be shifted far enough to separate them from the statements. If more than one short comment appears in a chunk of code, they should all be indented to the same tab setting
if (a == 2) {
return TRUE; /* special case */
} else {
return isprime(a); /* works only for odd a */
}
The // comment delimiter begins a comment that continues to the newline. It can comment out a complete line or only a partial line. It shouldn’t be used on consecutive multiple lines for text comments; however, it can be used in consecutive multiple lines for commenting out sections of code. But in final code we remove all the commented codes. Examples of all styles follow:
if (foo > 1) {
// Do a double-flip.
...
}
else
return false; // Explain why here.
//if (bar > 1) {
//
// // Do a triple-flip.
// ...
// }
//else
// return false;
Doc comments describe Java classes, interfaces, constructors, methods, and fields. Each doc comment is set inside the comment delimiters /**...*/, with one comment per API. This comment should appear just before the declaration:
Note - type /** and press Return (Enter) to let IntelliJ IDEA automatically generate JavaDoc stubs
/**
* The Example class provides ...
*/
class Example { …
/**
* Add String to the buffer
*
* @param tempBuffer the buffer to add the attribute to.
* @param offset offset to add the attribute.
* @param str the attribute value (a string).
* @param type the attribute type.
* @return the offset after adding the attribute
*/
private static int addString(byte[] tempBuffer, int offset, String str, byte type) {
The if-else class of statements should have the following form:
if (condition) {
statements;
}
if (condition) {
statements;
} else {
statements;
}
if (condition) {
statements;
} else if (condition) {
statements;
} else if (condition) {
statements;
}
Note: if statements always use braces {}. Avoid the following error-prone form:
if (condition) //AVOID! THIS OMITS THE BRACES {}!
Statement;
A try-catch statement should have the following format:
try {
statements;
} catch (ExceptionClass e) {
statements;
}
Blank lines improve readability by setting off sections of code that are logically related.
Two blank lines should always be used in the following circumstances: • Between sections of a source file • Between class and interface definitions
One blank line should always be used in the following circumstances: • Between methods • Between the local variables in a method and its first statement • Before a block or single-line comment • Between logical sections inside a method to improve readability
Blank spaces should be used in the following circumstances:
• A keyword followed by a parenthesis should be separated by a space.
Example:
while (true) {
...
}
Note- that a blank space should not be used between a method name and its opening parenthesis. This helps to distinguish keywords from method calls.
• A blank space should appear after commas in argument lists. • All binary operators except . should be separated from their operands by spaces.
Blank spaces should never separate unary operators such as unary minus, increment (“++”), and decrement (“--”) from their operands. Example:
a += c + d;
a = (a + b) / (c * d);
while (d++ = s++) {
n++;
}
prints("size is " + foo + "\n");
• The expressions in a for statement should be separated by blank spaces.
Example:
for (expr1; expr2; expr3)
• Casts should be followed by a blank.
Examples:
myMethod((byte) aNum, (Object) x);
myFunc((int) (cp + 5), ((int) (i + 3)) + 1);
Note - Better to have whitespace when you print an error message, so can identify the error message and exception clearly. log.error("Could not get the Datagram responsePacket ", ioe);
Wso2 has created a code style template for apply Best Practices easily.But the best way to coding is to follow the best practices rules while you are coding. The following steps show how to configure wso2 code style template with IntelliJ IDEA.
Step 01
Download the WSO2 code style template from
https://raw.githubusercontent.com/wso2/product-is/master/docs/wso2_codestyle.xml
and save it as wso2_codestyle.xml
Step 02
In the intellij IDEA go to the IntelliJ IDEA > Preferences > Code Style > Scheme
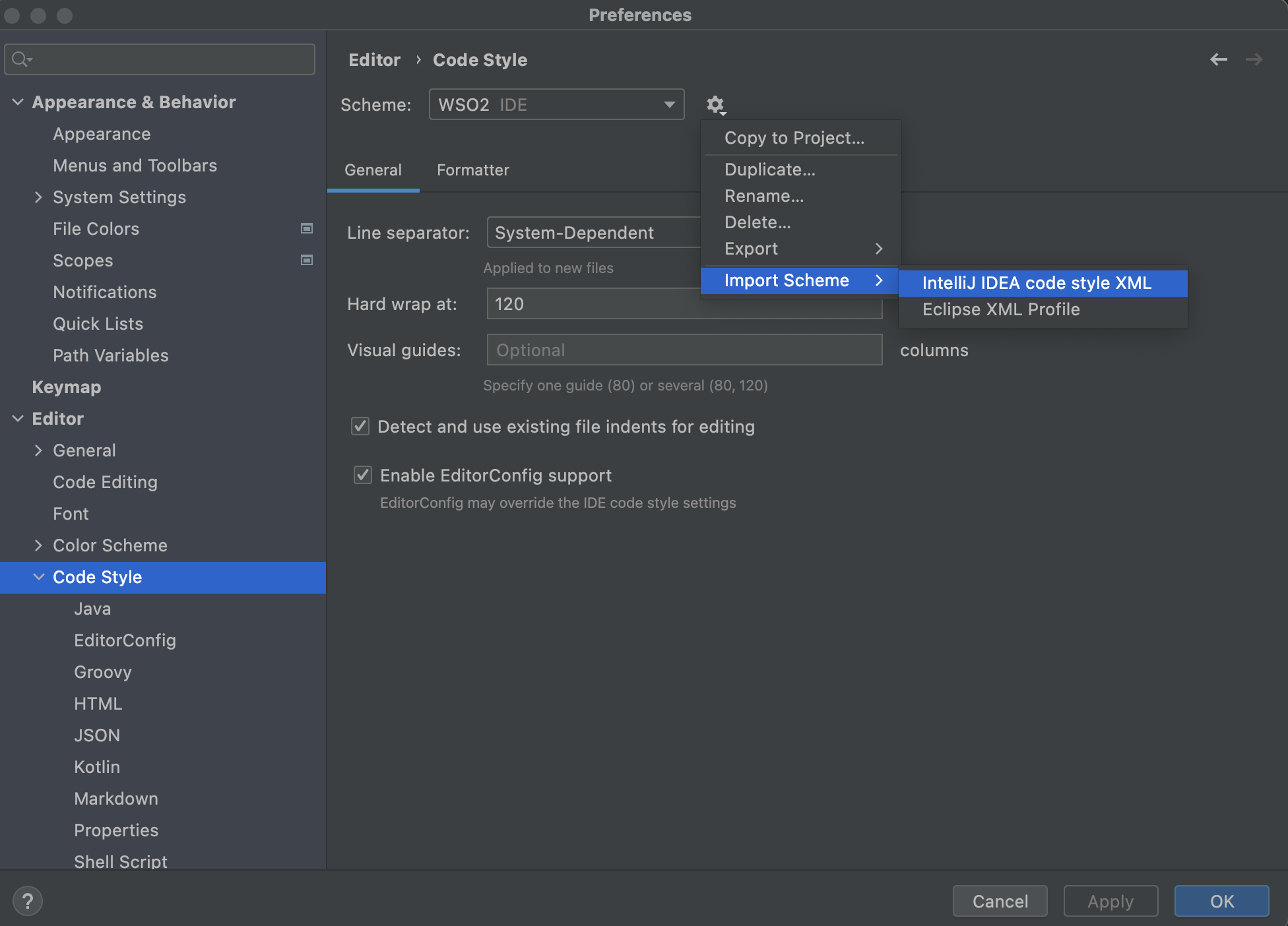
Select Import Scheme > Intellij IDEA code style XML and select wso2-codestyle from the download folder
Step 03
Restart the Intellij IDEA
SonarLint - SonarLint is an IDE extension that helps you detect and fix quality issues as you write code. Like a spell checker, SonarLint squiggles flaws so they can be fixed before committing code. You can get it directly from the IntelliJ IDEA Plugin Repository
The FindBugs --discontinued and may not work in the latest versions - Provides static byte code analysis to look for bugs in Java code from within IntelliJ IDEA. FindBugs is a defect detection tool for Java that uses static analysis to look for more than 200 bug patterns, such as null pointer dereferences, infinite recursive loops, bad uses of the Java libraries and deadlocks. FindBugs can identify hundreds of serious defects in large applications (typically about 1 defect per 1000-2000 lines of non-commenting source statements).
The SpotBugs - Provides static byte code analysis to look for bugs in Java code from within IntelliJ IDEA. SpotBugs is a defect detection tool for Java that uses static analysis to look for more than 400 bug patterns, such as null pointer dereferences, infinite recursive loops, bad uses of the Java libraries and deadlocks. SpotBugs can identify hundreds of serious defects in large applications (typically about 1 defect per 1000-2000 lines of non-commenting source statements)
Working in progress .......
Topics that are going to cover
- Code,
- Write Test cases,
- How to patch and test the changes
- Document
- How to submit PR
Refference - https://wso2.github.io/ https://github.com/wso2/wso2.github.io/blob/master/documentation-guidelines.md
Please refer below medium post for to get better understanding on how to Customizing WSO2 Log Files
https://medium.com/@pamodaaw/customizing-wso2-log-files-a338139620fe
Working in progress .......
After you have written your connector you can add it in to the WSO2 connector store You can follow the following documentation for that purpose.
Working in progress .......
Topics that are going to cover
- How to raise issues
WSO2 has a public bug-tracking system that you can use to report issues, submit enhancement requests, and track and comment on issues. You can also use this system to report issues related to WSO2 product documentation . If you find a bug, first search the dev mailing list to see if someone has faced the same issue, and also check the bug-tracking system. If you can't find any information on the issue, create an issue in the bug-tracking system with as much information as possible, including the product version you were using, how to reproduce the issue, etc.
- How to report a security issue
The WSO2 security team welcomes contributions from our user community, developers, and security researchers to reinforce our product security. If you find a security vulnerabilities, we strongly encourage you to report them to our private security mailing list security@wso2.com before disclosing them in any public forums. The security team will be more than happy to assist you in such efforts. For more information on vulnerabilities and how they are handled, visit https://wso2.com/security.
Make sure the IDE is configured properly according to WSO2 code style by looking at the PR, and if not help to configure IDEA properly . Following are some of the hints that can be used to check whether IDE is configured or not.
For Java code
- Size of the line.
- Availability of license header.
- Format of the license header.
- Format of the method signature and exception handling style.
Checklist for 5 mins sanity test
The objective of this checklist is to enhance productivity of the code review process detecting very basic and obvious mistakes within 5 minutes time.
- Code without adequate level of test cases (unless a valid reason is provided )
- Catching/throwing/handling java.lang.Exception without commenting the reason.
- Non descriptive class comment.
- No API comments in public APIs
- Magic numbers/values exists in the code instead of proper constants.
- Long methods, long classes, long and complex blocks exists in the code ( in average 25 lines and maximum 40 lines).
- Duplicate code segments found in the code.
- Debug logs are not sufficient and/or doesn’t provide context information.
- Deprecating without providing a link to new implementation or reason for removal
Checklist for detailed review
The objective of this checklist is to make a final call about whether the PR should be merged or need to be reviewed again.
- Unclear algorithms in the code.
- Use of “public” field.
- Modifiable collection exists.
- Improper naming of APIs