-
Notifications
You must be signed in to change notification settings - Fork 1.7k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Replace gtk_combo_box_text_insert_text with direct model insertion #23443
Conversation
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Thanks for improving this!
We can definitely merge this already or you could address the 2 minor remarks below if you'd like.
Concerning the API breakage: functions prefixed with GTK
are specifically meant to be private and not part of the public API and so we can remove it without problem. Unfortunately we do need to keep it in 3.2 to avoid breaking the ABI...
Thanks for clarifying this. I have gone ahead and removed it from the class then, collapsing everything into |
Thanks for the updates! I think it would be better if you rebased the commits here, so that I could cherry-pick just a single commit to 3.2. |
There is a lot of overhead in the gtk_combo_box_text_insert_text function, so adding a lot of items to a choicebox can be an expensive operation when it is used. Instead directly access the underlying data model and add the items to it. Experiments show that for adding 10000 items to a wxChoice, the amount of time spent in wxChoice::DoInsertItems for each method are: gtk_combo_box_text_insert_text: 6.75s gtk_list_store_insert_with_values: 438.2ms
The base function now has this exact functionality, but is faster.
Instead of having a special function for this, collapse it down into the DoInsertItems function to allow for only querying the model once from the combobox. This function is considered private because of the GTK prefix, so its removal isn't API-breaking.
Ok, I have rebased everything so now only 1 commit (the first) needs to be cherry-picked, everything else is master-only. |
Great, thanks! Will merge (and backport) soon. |
There is a lot of overhead in the gtk_combo_box_text_insert_text function, so adding a lot of items to a choicebox can be an expensive operation when it is used. Instead directly access the underlying data model and add the items to it. Experiments show that for adding 10000 items to a wxChoice, the amount of time spent in wxChoice::DoInsertItems for each method are: gtk_combo_box_text_insert_text: 6.75s gtk_list_store_insert_with_values: 438.2ms (cherry picked from commit 983608c) See wxWidgets#23443.
There is a lot of overhead in the
gtk_combo_box_text_insert_text
function, so adding a lot of items to a choicebox can be an expensive operation when it is used. We have encountered this in KiCad when trying to use a wxChoiceBox as a font chooser on systems that have a lot of fonts installed (https://gitlab.com/kicad/code/kicad/-/issues/14277).I traced back some of the slow performance on creation of the wxChoiceBox to the method that was being used to add the items to the combobox. Experiments showed that adding 10000 items to the choicebox takes 6.75s in the current implementation using
gtk_combo_box_text_insert_text
. The other way of adding items is to directly access the underlying data model usinggtk_list_store_insert_with_values
. Using the data model access, experiments showed the time needed to add 10000 items dropped to 438.2ms.It turns out, that was already the method used in
wxBitmapComboBox
, so this actually collapses that down into the basewxChoice
class, removing the overriden function fromwxBitmapComboBox
. I tried using the same 2-step insertion pattern fromwxBitmapComboBox
(callinggtk_list_store_insert
followed bygtk_list_store_set_value
, but it turns out this is just as slow as the original method, and using the 1-step method by callinggtk_list_store_insert_with_values
was much faster).I have split this into two separate commits. The first is ABI-compatible, so it would be good to backport it to 3.2. The second removes the now redundant function from
wxBitmapComboBox
, so it changes the ABI (but leaves the API). I would have preferred to just collapse outGTKInsertComboBoxTextItem
entirely so each item didn't have to query for the model, but that would remove that from the API, and it could theoretically be being overriden by user's in their own implementations currently.This doesn't completely solve the performance issues for the large choicebox though, there is still a large performance hit when computing the text size and showing the menu, but that needs to be handled separately (and I haven't quite figured out the best way to do that yet).
These experiments can be seen using the minimal sample with this diff:
The flamegraph from the original implementation is:
The flamegraph from the proposed implementation is (note the different scale on the time axis):
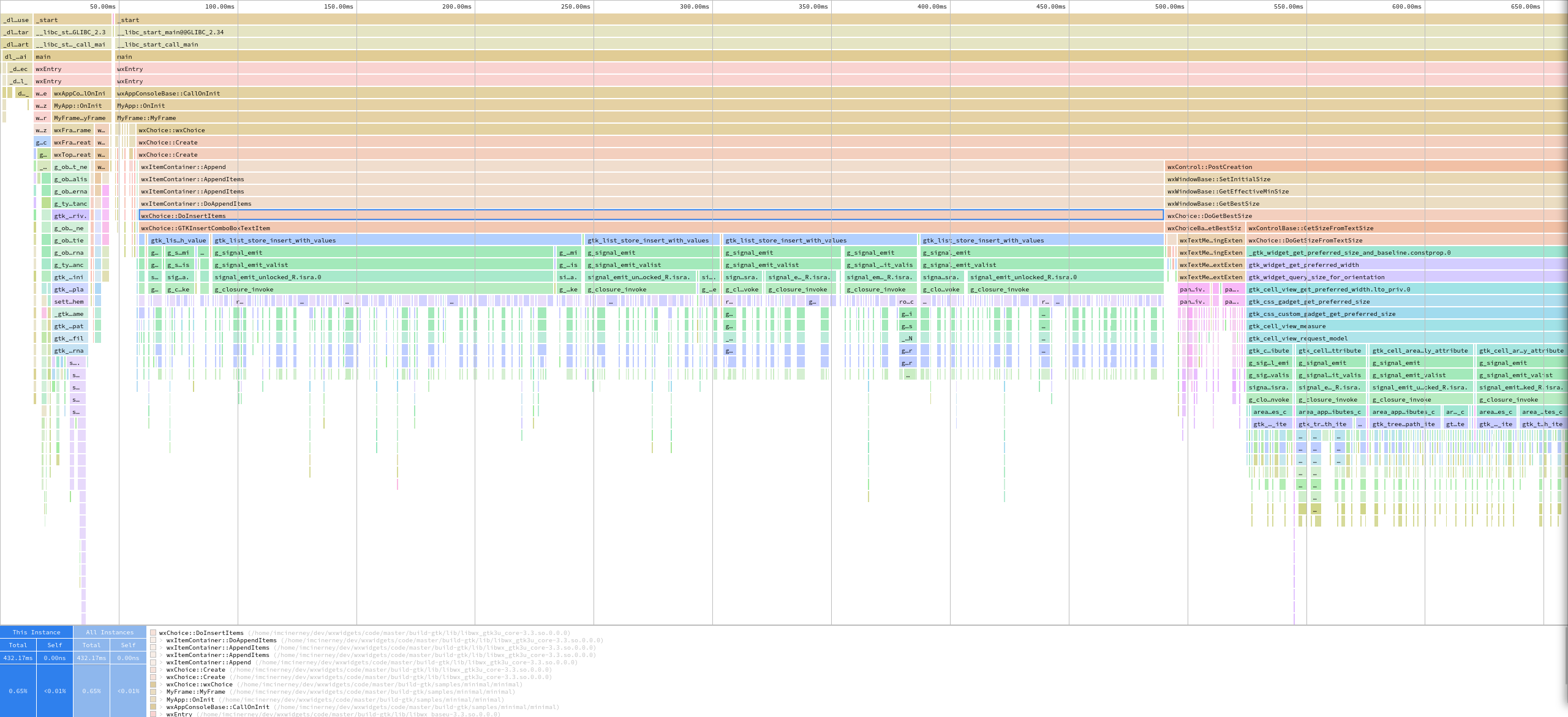