-
Notifications
You must be signed in to change notification settings - Fork 526
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
AndroidClientHandler TLS 1.1 and 1.2 support on Android 4.1 to 4.4 #1615
Comments
grendello
added a commit
to grendello/xamarin-android
that referenced
this issue
Sep 14, 2018
Context: dotnet#1615 It turns out that the older platforms do support TLS 1.1 and 1.2 protocols but that they don't enable it by default. Thanks to code provided by https://github.com/gameleon-dev in the issue linked to above we now support it too. Updated the TLS 1.2 tests to enable them on platforms >= 16
PR incorporating the code from OP is up, thanks @gameleon-dev! |
grendello
added a commit
to grendello/xamarin-android
that referenced
this issue
Sep 14, 2018
Context: dotnet#1615 It turns out that the older platforms do support TLS 1.1 and 1.2 protocols but that they don't enable it by default. Thanks to code provided by https://github.com/gameleon-dev in the issue linked to above we now support it too. Updated the TLS 1.2 tests to enable them on platforms >= 16
grendello
added a commit
to grendello/xamarin-android
that referenced
this issue
Sep 14, 2018
Context: dotnet#1615 It turns out that the older platforms do support TLS 1.1 and 1.2 protocols but that they don't enable it by default. Thanks to code provided by https://github.com/gameleon-dev in the issue linked to above we now support it too. Updated the TLS 1.2 tests to enable them on platforms >= 16
Fixed in: d8f1783 |
Sign up for free
to subscribe to this conversation on GitHub.
Already have an account?
Sign in.
Currently the
AndroidClientHandler
only supports TLS 1.1 and TLS 1.2 on API Level 20 or up.However, the
SslSocket
instances thatJava.Net.HttpURLConnection
(and by extensionAndroidClientHandler
) utilizes do have support for TLS 1.1 and 1.2, but they are disabled by default on API Level 16 to 19 (Android 4.1 to 4.4).This is shown in the following screenshot when such a socket is examined during runtime on Android 4.1 to 4.4 (screenshot is API Level 17/Android 4.2):
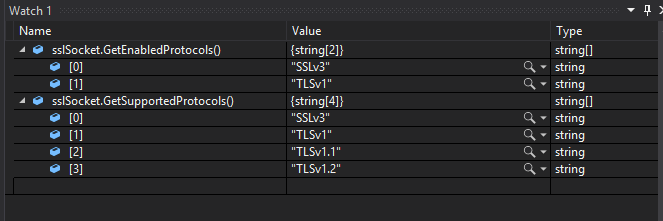
Currently I solved this by subclassing
AndroidClientHandler
and implementing a customSSLSocketFactory
that builts upon the default implementation.It's based on a solution originally provided for ModernHttpClient
This allows the AndroidClientHandler to support TLS 1.1 and TLS 1.2 on pre-API 20 Android versions.
Does this solution have any major caveats to it, or could this possibly be implemented in AndroidClientHandler itself?
The text was updated successfully, but these errors were encountered: