-
Notifications
You must be signed in to change notification settings - Fork 125
Raycasting the map
Using the MapRenderer's Raycast API, it is possible to detect the point and location (LatLonAlt) where a ray intersects the map surface.
If a ray intersects the map, the following information is provided about the hit:
- The point in world space
- The triangle normal in world space
- The distance from the ray origin to the hit point (in world space)
- The LatLonAlt of the hit point
The raycast API enables various features and scenarios. A basic example would be handling tap and mouse-clicks to determine what LatLon a user is clicking on.
Examples of more complex scenarios are those that enable map manipulations like dragging and panning the map with a mouse, or the pointer of a mixed reality controller:
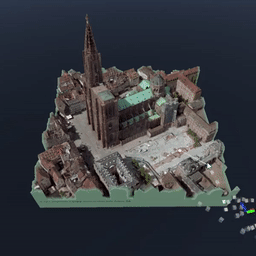
Generate a ray first. Unity provides helper methods like ScreenPointToRay
to generate rays based on a pixel position and camera. Likewise, the Mixed Reality Toolkit allows you to query the ray associated with a given pointer.
Once a ray has been acquired, use that along with optional parameters like max distance, to perform the raycast on a specified MapRenderer.
var ray = camera.ScreenPointToRay(Input.mousePosition);
if (mapRenderer.Raycast(ray, out MapRendererRaycastHit hitInfo))
{
var hitPoint = hitInfo.Point;
// Write your raycast hit logic here.
}
To build functionality that leverages the raycast API, like map interactions, it may also be useful to convert points from Unity space to LatLon relative to the map. The MapRendererTransformExtensions provide the methods for these conversions.
Raycasting takes a short amount of time to execute, but this is done on the Unity thread where time is critical, especially for mixed reality scenarios where frame targets can be upwards of 90 FPS.
It is best to minimize the raycast API usage: Don't call the API unnecessarily. Also, avoid cases where the API is called with the same arguments in a given frame. In this case, the original result can be cached and reused.
Furthermore, if it makes sense, use the overload API that provides a max distance. This may reduce the overall time of the raycast by avoiding testing portions of the map that may be along the ray direction but exceed the maximum distance.
- Configuring the map
- Attaching GameObjects
- Adding labels
- Animating the map
- Raycasting the map
- Displaying copyrights
- Customizing map data
- Displaying contour lines
- Microsoft.Geospatial
- Microsoft.Geospatial.VectorMath
-
Microsoft.Maps.Unity
- ClippingVolumeDistanceTextureResolution
- ClusterMapPin
- CoordinateClamping
- DefaultElevationTileLayer
- DefaultTextureTileLayer
- DefaultTrafficTextureTileLayer
- ElevationTile
- ElevationTileLayer
- ElevationTileLayerList
- FontStyle
- FontWeight
- HttpTextureTileLayer
- IMapSceneAnimationController
- Intersection
- IntersectionType
- IPinnable
- LanguageChangedEvent
- LatLonAltUnityEvent
- LatLonUnityEvent
- LatLonWrapper
- MapColliderType
- MapConstants
- MapContourLineLayer
- MapCopyrightAlignment
- MapCopyrightLayer
- MapDataCache
- MapDataCacheBase
- MapDeveloperKeySource
- MapImageryStyle
- MapImageryType
- MapInteractionController
- MapInteractionHandler
- MapLabel
- MapLabelLayer
- MapLayer
- MapMouseInteractionHandler
- MapPin
- MapPinLayer
- MapRenderer
- MapRendererBase
- MapRendererRaycastHit
- MapRendererTransformExtensions
- MapScaleRatioExtensions
- MapScene
- MapSceneAnimationController
- MapSceneAnimationKind
- MapSceneOfBoundingBox
- MapSceneOfLabelAndZoomLevel
- MapSceneOfLocationAndZoomLevel
- MapSession
- MapShape
- MapTerrainType
- MapTouchInteractionHandler
- ObservableList
- ObservableMapPinList
- ServiceOptions
- Style
- SystemLangaugeConverter
- TextureTile
- TextureTileLayer
- TextureTileLayerList
- TileLayer
- TileLayerList
- UnityTaskFactory
- UnityWebRequestAwaiter
- UnityWebRequestAwaiterExtensionMethods
- WaitForMapLoaded
- WaitForMapSceneAnimation
- Microsoft.Maps.Unity.Search