-
Notifications
You must be signed in to change notification settings - Fork 761
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Proj 6.1.1 to 8.0.1 performance issues and regressions #2785
Comments
Are the longitude, latitude covering the whole world/a large extent or a small extent ? And if covering a large extent, are consecutive points in "random order" or are they generally grouped by location ? I'm asking this since the us_nga_egm96_15.tif uses 256x256 tiles (18 tiles for the whole world), and PROJ has a cache for 12 tiles per file. Could you also expand the vTune profile for GTiffVGrid::valueAt ? |
Here's a summary of the point-count, south-west, and north-east bounds for each data group: It's a small region near 37 latitude, -119 longitude. It should be scanning west to east, south to north. |
With this commit, and the 2 previous ones, given mytest.cpp ``` int main() { PJ* pj = proj_create(nullptr, "+proj=vgridshift +grids=us_nga_egm96_15.tif"); for( int i = 0; i < 5*1000*1000; i++) { PJ_COORD coord; coord.lpz.lam = 0; coord.lpz.phi = 0; coord.lpz.z = 0; proj_trans(pj, PJ_FWD, coord); } return 0; } ``` we get a x2 speedup Before: ``` $ PROJ_LIB=data:$HOME/proj/PROJ-data/us_nga LD_LIBRARY_PATH=src/.libs hyperfine --warmup 1 'taskset -c 11 ./mytest' Benchmark #1: taskset -c 11 ./mytest Time (mean ± σ): 1.950 s ± 0.014 s [User: 1.945 s, System: 0.005 s] Range (min … max): 1.937 s … 1.971 s ``` After: ``` $ PROJ_LIB=data:$HOME/proj/PROJ-data/us_nga LD_LIBRARY_PATH=src/.libs hyperfine --warmup 1 'taskset -c 11 ./mytest' Benchmark #1: taskset -c 11 ./mytest Time (mean ± σ): 984.4 ms ± 3.1 ms [User: 977.0 ms, System: 7.2 ms] Range (min … max): 979.3 ms … 990.5 ms ```
With this commit, and the 2 previous ones, given mytest.cpp ``` int main() { PJ* pj = proj_create(nullptr, "+proj=vgridshift +grids=us_nga_egm96_15.tif"); for( int i = 0; i < 5*1000*1000; i++) { PJ_COORD coord; coord.lpz.lam = 0; coord.lpz.phi = 0; coord.lpz.z = 0; proj_trans(pj, PJ_FWD, coord); } return 0; } ``` we get a x2 speedup Before: ``` $ PROJ_LIB=data:$HOME/proj/PROJ-data/us_nga LD_LIBRARY_PATH=src/.libs hyperfine --warmup 1 'taskset -c 11 ./mytest' Benchmark #1: taskset -c 11 ./mytest Time (mean ± σ): 1.950 s ± 0.014 s [User: 1.945 s, System: 0.005 s] Range (min … max): 1.937 s … 1.971 s ``` After: ``` $ PROJ_LIB=data:$HOME/proj/PROJ-data/us_nga LD_LIBRARY_PATH=src/.libs hyperfine --warmup 1 'taskset -c 11 ./mytest' Benchmark #1: taskset -c 11 ./mytest Time (mean ± σ): 984.4 ms ± 3.1 ms [User: 977.0 ms, System: 7.2 ms] Range (min … max): 979.3 ms … 990.5 ms ```
GeoTIFF grid reading: perf improvements (fixes #2785)
GeoTIFF grid reading: perf improvements (fixes #2785)
[Backport 8.1] GeoTIFF grid reading: perf improvements (fixes #2785)
GeoTIFF grid reading: perf improvements (fixes OSGeo#2785)
Example of problem
Problem description
I have compiled and run the above example on visual studio 2019 with a preprocessed data file (~500 mb of lat/lon/elevation data). I run it against gdal/proj 3.0.1/6.1.1 and 3.2.1/8.0.1.
The vTune profile from 6.1.1 is:
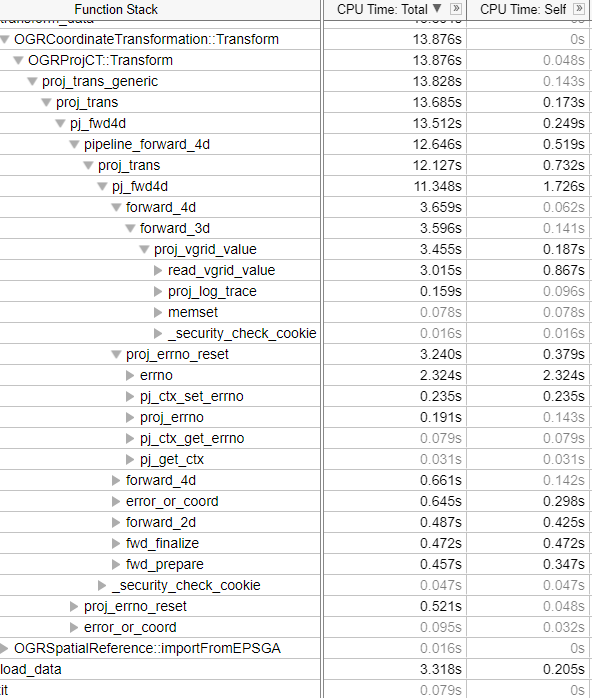
The vTune profile from 8.0.1 is:
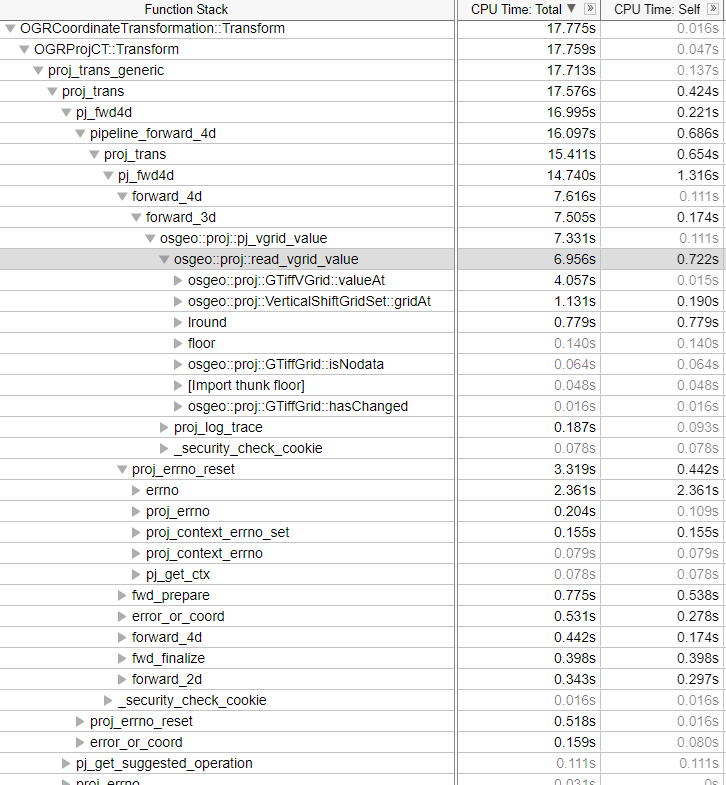
Besides the performance regression, it is notable that about 25% of the runtime in both cases is spent just calling proj_errno_reset...not sure if anything can be done about that.
Environment Information
proj
) : 6.1.1/8.0.1Installation method
The text was updated successfully, but these errors were encountered: