-
Notifications
You must be signed in to change notification settings - Fork 31
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
0 parents
commit 7639683
Showing
7 changed files
with
447 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,115 @@ | ||
/* FreqMeasure Library, for measuring relatively low frequencies | ||
* http://www.pjrc.com/teensy/td_libs_FreqMeasure.html | ||
* Copyright (c) 2011 PJRC.COM, LLC - Paul Stoffregen <paul@pjrc.com> | ||
* | ||
* Version 1.0 | ||
* | ||
* Permission is hereby granted, free of charge, to any person obtaining a copy | ||
* of this software and associated documentation files (the "Software"), to deal | ||
* in the Software without restriction, including without limitation the rights | ||
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
* copies of the Software, and to permit persons to whom the Software is | ||
* furnished to do so, subject to the following conditions: | ||
* | ||
* The above copyright notice and this permission notice shall be included in | ||
* all copies or substantial portions of the Software. | ||
* | ||
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN | ||
* THE SOFTWARE. | ||
*/ | ||
|
||
#include "FreqMeasure.h" | ||
#include "util/capture.h" | ||
|
||
#define FREQMEASURE_BUFFER_LEN 12 | ||
static volatile uint32_t buffer_value[FREQMEASURE_BUFFER_LEN]; | ||
static volatile uint8_t buffer_head; | ||
static volatile uint8_t buffer_tail; | ||
static uint16_t capture_msw; | ||
static uint32_t capture_previous; | ||
|
||
|
||
void FreqMeasureClass::begin(void) | ||
{ | ||
capture_init(); | ||
capture_msw = 0; | ||
capture_previous = 0; | ||
buffer_head = 0; | ||
buffer_tail = 0; | ||
capture_start(); | ||
} | ||
|
||
uint8_t FreqMeasureClass::available(void) | ||
{ | ||
uint8_t head, tail; | ||
|
||
head = buffer_head; | ||
tail = buffer_tail; | ||
if (head >= tail) return head - tail; | ||
return FREQMEASURE_BUFFER_LEN + head - tail; | ||
} | ||
|
||
uint32_t FreqMeasureClass::read(void) | ||
{ | ||
uint8_t head, tail; | ||
uint32_t value; | ||
|
||
head = buffer_head; | ||
tail = buffer_tail; | ||
if (head == tail) return 0xFFFFFFFF; | ||
tail = tail + 1; | ||
if (tail >= FREQMEASURE_BUFFER_LEN) tail = 0; | ||
value = buffer_value[tail]; | ||
buffer_tail = tail; | ||
return value; | ||
} | ||
|
||
void FreqMeasureClass::end(void) | ||
{ | ||
capture_shutdown(); | ||
} | ||
|
||
|
||
ISR(TIMER_OVERFLOW_VECTOR) | ||
{ | ||
capture_msw++; | ||
} | ||
|
||
ISR(TIMER_CAPTURE_VECTOR) | ||
{ | ||
uint16_t capture_lsw; | ||
uint32_t capture, period; | ||
uint8_t i; | ||
|
||
// get the timer capture | ||
capture_lsw = capture_read(); | ||
// Handle the case where but capture and overflow interrupts were pending | ||
// (eg, interrupts were disabled for a while), or where the overflow occurred | ||
// while this ISR was starting up. However, if we read a 16 bit number that | ||
// is very close to overflow, then ignore any overflow since it probably | ||
// just happened. | ||
if (capture_overflow() && capture_lsw < 0xFF00) { | ||
capture_overflow_reset(); | ||
capture_msw++; | ||
} | ||
// compute the waveform period | ||
capture = ((uint32_t)capture_msw << 16) | capture_lsw; | ||
period = capture - capture_previous; | ||
capture_previous = capture; | ||
// store it into the buffer | ||
i = buffer_head + 1; | ||
if (i >= FREQMEASURE_BUFFER_LEN) i = 0; | ||
if (i != buffer_tail) { | ||
buffer_value[i] = period; | ||
buffer_head = i; | ||
} | ||
} | ||
|
||
|
||
FreqMeasureClass FreqMeasure; | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
#ifndef FreqMeasure_h | ||
#define FreqMeasure_h | ||
|
||
#include <inttypes.h> | ||
|
||
class FreqMeasureClass { | ||
public: | ||
static void begin(void); | ||
static uint8_t available(void); | ||
static uint32_t read(void); | ||
static void end(void); | ||
}; | ||
|
||
extern FreqMeasureClass FreqMeasure; | ||
|
||
#endif | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
#FreqMeasure Library# | ||
|
||
FreqMeasure measures the elapsed time during each cycle of an input frequency. | ||
|
||
http://www.pjrc.com/teensy/td_libs_FreqMeasure.html | ||
|
||
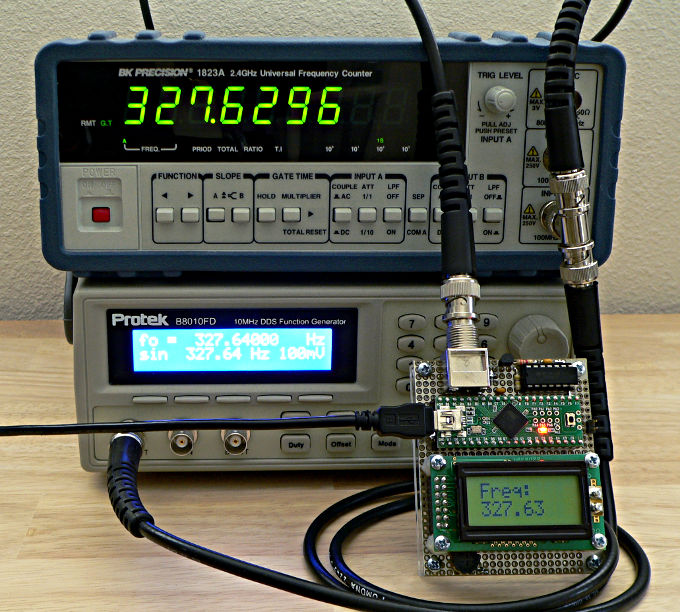 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,36 @@ | ||
/* FreqMeasure - Example with LCD output | ||
* http://www.pjrc.com/teensy/td_libs_FreqMeasure.html | ||
* | ||
* This example code is in the public domain. | ||
*/ | ||
#include <FreqMeasure.h> | ||
#include <LiquidCrystal.h> | ||
|
||
LiquidCrystal lcd(5, 4, 3, 2, 1, 0); | ||
|
||
void setup() { | ||
Serial.begin(57600); | ||
lcd.begin(8, 2); | ||
lcd.print("Freq:"); | ||
FreqMeasure.begin(); | ||
} | ||
|
||
double sum=0; | ||
int count=0; | ||
|
||
void loop() { | ||
if (FreqMeasure.available()) { | ||
// average several reading together | ||
sum = sum + FreqMeasure.read(); | ||
count = count + 1; | ||
if (count > 30) { | ||
double frequency = F_CPU / (sum / count); | ||
lcd.setCursor(0, 1); | ||
lcd.print(frequency); | ||
lcd.print(" "); | ||
sum = 0; | ||
count = 0; | ||
} | ||
} | ||
} | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
/* FreqMeasure - Example with serial output | ||
* http://www.pjrc.com/teensy/td_libs_FreqMeasure.html | ||
* | ||
* This example code is in the public domain. | ||
*/ | ||
#include <FreqMeasure.h> | ||
|
||
void setup() { | ||
Serial.begin(57600); | ||
FreqMeasure.begin(); | ||
} | ||
|
||
double sum=0; | ||
int count=0; | ||
|
||
void loop() { | ||
if (FreqMeasure.available()) { | ||
// average several reading together | ||
sum = sum + FreqMeasure.read(); | ||
count = count + 1; | ||
if (count > 30) { | ||
double frequency = F_CPU / (sum / count); | ||
Serial.println(frequency); | ||
sum = 0; | ||
count = 0; | ||
} | ||
} | ||
} | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,5 @@ | ||
FreqMeasure KEYWORD1 | ||
begin KEYWORD2 | ||
available KEYWORD2 | ||
read KEYWORD2 | ||
end KEYWORD2 |
Oops, something went wrong.