New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Fix incorrect glyph rect for text outline #1827
Conversation
Thanks a lot for the issue with immediate PR! I'll try to have a look at it on Windows this week. |
Summoning our fonts expert, @oomek 😄 |
Sure, on it. |
There was indeed funny business going on with outline and style flags applied. @cschreib commit seems to fix the rendering with every font, style flag and outline combination I tested so far. Forced autohint advance compensation is still working as before. Thanks for spotting it and fixing. SFML/src/SFML/Graphics/Font.cpp Line 634 in 40a0584
From what I see the root.advance.x is now of long type and a multiplier of 65536 so you could replace it with just:
glyph.advance = static_cast<float>(bitmapGlyph->root.advance.x >> 16); Update: a little explanation why we can just |
Since auto-hinting is enabled, the advance will always be an integer number of pixels. The actual fractional advance is handled by bearings. SFML#1827 (comment)
Thanks for the explanation, that makes sense. I work in parallel on my own FreeType+OpenGL renderer, which does not use hinting, and it needed I have pushed a commit to apply this suggestion.
It sounds like it may be a fundamental problem with the Freetype stroker: When the outline thickness becomes large enough compared to the font size, it is probably best to simply convolve the non-outlined glyph with a circular mask (of radius = outline thickness). This could quickly become a can of worms, so I'm not sure this should be done in this PR. |
If the two issues aren't directly related, then I too suggest to separate these. If it's really something that SFML can fix/improve then feel free to create another issue, so it can be tracked. |
I agree, the guy from freetype repo just confirmed that limitation of the stroker algorithm, I'll keep researching the subject. |
Thanks a lot for finding this, reporting and fixing it, highly appreciated! 🙂 |
After porting this change to my own code, I noticed that it removes the outline around the underline when sf::Text::Underlined is included in the text style. The same is probably true for strikethrough. |
Oh oh 😅 So if only addGlyphQuad is adjusted, does it then still fix the original issue, @cschreib? |
Anyone willing to provide a fix for the fix, otherwise I'll try to pick it up when I get around to it... |
Sorry, I didn't test strikethrough or underline when making the original changes... I will have a look tomorrow morning, or later this week. |
Yep my bad, I did not look closely enough at the code. I thought |
@texus assuming this fixed it for you as well? |
@eXpl0it3r Yes, #1836 fixed it. |
Description
This PR fixes the glyph rect calculation in
sf::Font
to use the most accurate information from the FreeType rendered bitmap data, rather than the glyph metrics. The returned rect is guaranteed to contain the full rendered glyph with no stretching. It also simplifies the positioning insf::Text
, since the offsetting from the outline is now correctly taken into account in the returned rect fromsf::Font
.As a side effect, it seems to have "fixed" an unintended stretching for bold fonts:
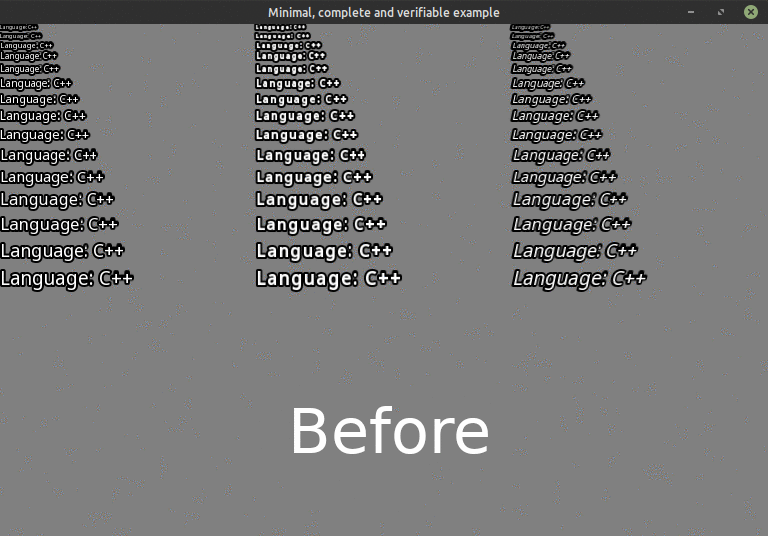
This PR is related to the issue #1826
Tasks
How to test this PR?
Describe how to best test these changes. Please provide a minimal, complete and verifiable example if possible, you can use the follow template as a start: