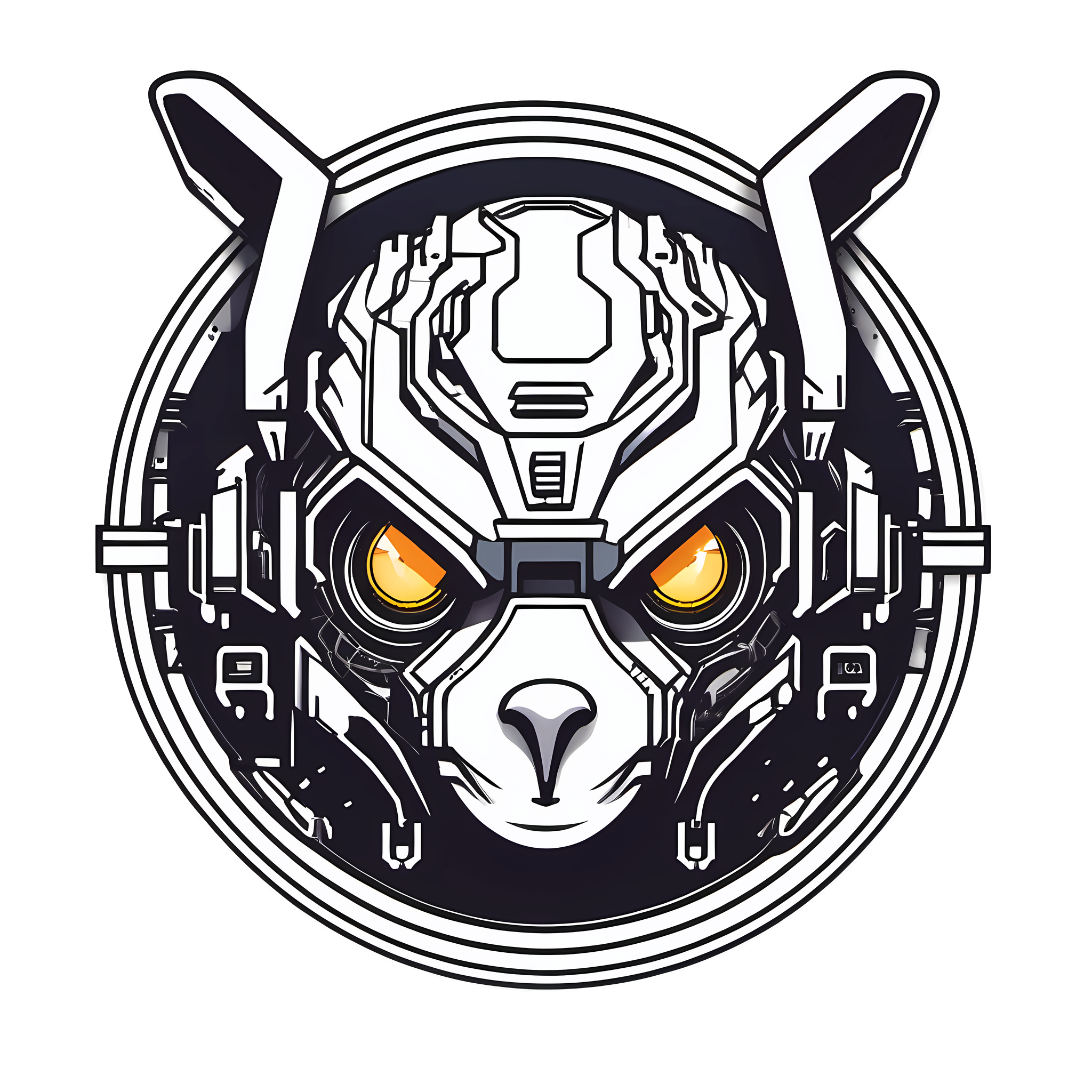
Golang SDK for stable-diffusion-webui's API
Among the contributors is AUTOMATIC1111, a little joke on my part😂.
Here,sincerely thank AUTOMATIC1111 for its great contribution to AIGC
This is a Go language version of the SDK based on stable-diffusion-webui. In your code, you can directly use the API interfaces of stable-diffusion-webui through object-oriented operations, instead of dealing with cumbersome JSON.
Support extensions API !
You can check the support list for intersvc in this wiki page.
There are two methods to use the SDK.
-
Using 'intersvc', which provides a highly encapsulated API interface for 'sd-webui'. Using this method feels like directly using the Go language version of 'sd-webui'.
-
Using 'go-swagger', which still involves object-oriented operations but is slightly more complex than 'intersvc'.
Almost all interfaces are supported by the second method, while the first one is gradually being supported.
In fact, most of the interfaces can be used with 'intersvc', but it requires defining the Response. The API documentation of 'sd-webui' does not provide the content of the Response, so it needs to be defined manually. You can refer to the "Participating" section below for specific instructions.
package main
import (
"encoding/base64"
"fmt"
SdClient "github.com/SpenserCai/sd-webui-go"
SdApiOperation "github.com/SpenserCai/sd-webui-go/stablediffusion/client/operations"
SdApiModel "github.com/SpenserCai/sd-webui-go/stablediffusion/models"
)
func MustBeNil(err error) {
if err != nil {
panic(err)
}
}
var URL = "127.0.0.1:7860"
func main() {
sdClient := SdClient.NewStableDiffInterface(URL)
rd := SdApiOperation.NewText2imgapiSdapiV1Txt2imgPostParams()
rd.Body = &SdApiModel.StableDiffusionProcessingTxt2Img{
Prompt: "dog",
NegativePrompt: "ugly",
ScriptArgs: []interface{}{},
}
resp, err := sdClient.Client.Operations.Text2imgapiSdapiV1Txt2imgPost(rd)
MustBeNil(err)
for i, s := range resp.Payload.Images {
b, err := base64.StdEncoding.DecodeString(s)
MustBeNil(err)
err = os.WriteFile(fmt.Sprintf("%d.png", i), b, 0644)
MustBeNil(err)
}
}
import (
SdClient "github.com/SpenserCai/sd-webui-go"
"github.com/SpenserCai/sd-webui-go/intersvc"
)
func main() {
// Create a client
sdClient := SdClient.NewStableDiffInterface("127.0.0.1:7860")
var f_factor int64 = 20
var artistic bool = false
// Set Request
deoldify_inter := &intersvc.DeoldifyImage{
RequestItem: &intersvc.DeoldifyImageRequest{
InputImage: "https://media.discordapp.net/attachments/1138408545277190237/1138508881635577947i7krs1njekla1.jpg",
RenderFactor: &f_factor,
Artistic: &artistic,
},
}
deoldify_inter.Action(sdClient)
if deoldify_inter.Error != nil {
panic(deoldify_inter.Error)
}
response := deoldify_inter.GetResponse()
}
Full example code: intersvc_example
import (
"encoding/base64"
"os"
SdClient "github.com/SpenserCai/sd-webui-go"
intersvc "github.com/SpenserCai/sd-webui-go/intersvc"
SdApiOperation "github.com/SpenserCai/sd-webui-go/stablediffusion/client/operations"
SdApiModel "github.com/SpenserCai/sd-webui-go/stablediffusion/models"
)
type DeoldifyResponse struct {
Image string `json:"image"`
}
func main() {
var (
err error
)
// init client
sdClient := SdClient.NewStableDiffInterface("127.0.0.1:7860")
var f_factor int64 = 20
var artistic bool = false
// set request data
RequestData := SdApiOperation.NewDeoldifyImageDeoldifyImagePostParams()
RequestData.Body = &SdApiModel.BodyDeoldifyImageDeoldifyImagePost{
InputImage: "https://media.discordapp.net/attachments/1138408545277190237/1138508881635577947/i7krs1njekla1.jpg",
RenderFactor: &f_factor,
Artistic: &artistic,
}
// send request
Response, err := sdClient.Client.Operations.DeoldifyImageDeoldifyImagePost(RequestData)
if err != nil {
panic(err)
}
// convert response
deoldifyRep, err := intersvc.ConvertResponse(Response.Payload, &DeoldifyResponse{})
if err != nil {
panic(err)
}
response := deoldifyRep.(*DeoldifyResponse)
}
Full example code: go-swagger_example
Most of the code for intersvc has been generated using a code generator. However, due to the lack of response information in the API documentation for sd-webui, it needs to be manually written.
You need to fork the code of the dev branch, make the necessary code updates, create a branch named dev-[model filename] in your own repository, and then submit a pull request to the dev branch of this repository.
In sd-webui-go/intersvc
,you can see some file like ***_model.go
. These files define the Response Model. You can refer to the following example to define the Response Model.
From
type DeoldifyImageResponse struct {
}
To
type DeoldifyImageResponse struct {
Image string `json:"image"`
}