This repository has been archived by the owner on Jun 26, 2020. It is now read-only.
-
Notifications
You must be signed in to change notification settings - Fork 40
I/6528: Faster paths concatenation #1835
Merged
Changes from 12 commits
Commits
Show all changes
13 commits
Select commit
Hold shift + click to select a range
4386a68
Use spread operator for concatenating paths in Position constructor.
jodator a5e9280
Use for loops to concatenate paths in model/Position.
jodator abf6d1f
Add docs to helper concatenatePaths() helper method.
jodator 75a53ec
Do not use library method in Position#offset.
jodator e49c55c
Add simple manual test for Position's path concatenation.
jodator eae2b48
Use position class to test performance.
jodator f8e5c8f
Use separate position classes.
jodator 5708ba2
Fix test performance.
jodator 184cb3d
Merge branch 'master' into i/6528
jodator dada66d
Add note about times in tests.
jodator 5485dbc
Merge branch 'master' into i/6528
Reinmar 3c7ea63
The root is passed most commonly and it has an empty path, so we can …
Reinmar e63694a
Removed a test that's unnecessary now.
Reinmar File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
<div id="test-controls"> | ||
<button id="run">Run</button> | ||
</div> | ||
|
||
<hr> | ||
|
||
<div id="output"></div> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,161 @@ | ||
/** | ||
* @license Copyright (c) 2003-2020, CKSource - Frederico Knabben. All rights reserved. | ||
* For licensing, see LICENSE.md or https://ckeditor.com/legal/ckeditor-oss-license | ||
*/ | ||
|
||
/* globals window, document, setTimeout */ | ||
|
||
document.getElementById( 'run' ).addEventListener( 'click', () => { | ||
log( 'Running tests...' ); | ||
|
||
setTimeout( async () => { | ||
await runTest( 'concat', testConcat ); | ||
await runTest( 'spread operator', testSpread ); | ||
await runTest( 'for-loop', testForLoop ); | ||
await runTest( 'ultra-loop', testUltraForLoop ); | ||
|
||
log( 'done' ); | ||
} ); | ||
} ); | ||
|
||
window.cache = []; | ||
|
||
const output = document.getElementById( 'output' ); | ||
|
||
function log( line ) { | ||
const paragraphElement = document.createElement( 'p' ); | ||
paragraphElement.innerText = line; | ||
output.appendChild( paragraphElement ); | ||
} | ||
|
||
function runTest( name, callback ) { | ||
return new Promise( resolve => { | ||
const start = new Date(); | ||
|
||
const repetitions = 10000000; | ||
|
||
const root = { | ||
root: 'foo', | ||
path: [ 0 ] | ||
}; | ||
const path = [ 0, 2 ]; | ||
|
||
for ( let i = 0; i < repetitions; i++ ) { | ||
const newPath = callback( root, path ); | ||
window.cache.push( newPath.length ); | ||
} | ||
|
||
const end = new Date(); | ||
|
||
log( ` > ${ name } took ${ end - start }ms` ); | ||
|
||
setTimeout( () => { | ||
resolve(); | ||
}, 50 ); | ||
} ); | ||
} | ||
|
||
class PositionConcat { | ||
constructor( root, path, stickiness = 'left' ) { | ||
if ( !( path instanceof Array ) || path.length === 0 ) { | ||
throw new Error( 'model-position-path-incorrect-format' ); | ||
} | ||
|
||
path = root.path.concat( path ); | ||
root = root.root; | ||
|
||
this.root = root; | ||
this.path = path; | ||
this.stickiness = stickiness; | ||
} | ||
} | ||
|
||
class PositionSpread { | ||
constructor( root, path, stickiness = 'left' ) { | ||
if ( !( path instanceof Array ) || path.length === 0 ) { | ||
throw new Error( 'model-position-path-incorrect-format' ); | ||
} | ||
|
||
path = [ ...root.path, ...path ]; | ||
root = root.root; | ||
|
||
this.root = root; | ||
this.path = path; | ||
this.stickiness = stickiness; | ||
} | ||
} | ||
|
||
class PositionForLoop { | ||
constructor( root, path, stickiness = 'left' ) { | ||
if ( !( path instanceof Array ) || path.length === 0 ) { | ||
throw new Error( 'model-position-path-incorrect-format' ); | ||
} | ||
|
||
path = forLoop( root.path, path ); | ||
root = root.root; | ||
|
||
this.root = root; | ||
this.path = path; | ||
this.stickiness = stickiness; | ||
} | ||
} | ||
|
||
class PositionUltraForLoop { | ||
constructor( root, path, stickiness = 'left' ) { | ||
if ( !( path instanceof Array ) || path.length === 0 ) { | ||
throw new Error( 'model-position-path-incorrect-format' ); | ||
} | ||
|
||
path = ultraForLoop( root.path, path ); | ||
root = root.root; | ||
|
||
this.root = root; | ||
this.path = path; | ||
this.stickiness = stickiness; | ||
} | ||
} | ||
|
||
function testConcat( root, path ) { | ||
return new PositionConcat( root, path ); | ||
} | ||
|
||
function testSpread( root, path ) { | ||
return new PositionSpread( root, path ); | ||
} | ||
|
||
function testForLoop( root, path ) { | ||
return new PositionForLoop( root, path ); | ||
} | ||
|
||
function testUltraForLoop( root, path ) { | ||
return new PositionUltraForLoop( root, path ); | ||
} | ||
|
||
function forLoop( rootPath, path ) { | ||
const newPath = []; | ||
|
||
for ( let i = 0; i < rootPath.length; i++ ) { | ||
newPath.push( rootPath[ i ] ); | ||
} | ||
|
||
for ( let i = 0; i < path.length; i++ ) { | ||
newPath.push( path[ i ] ); | ||
} | ||
|
||
return newPath; | ||
} | ||
|
||
function ultraForLoop( rootPath, path ) { | ||
const fullLength = rootPath.length + path.length; | ||
const newPath = new Array( fullLength ); | ||
|
||
for ( let i = 0; i < rootPath.length; i++ ) { | ||
newPath[ i ] = rootPath[ i ]; | ||
} | ||
|
||
for ( let i = 0; i < path.length; i++ ) { | ||
newPath[ rootPath.length + i ] = path[ i ]; | ||
} | ||
|
||
return newPath; | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
# Performance: pasting data | ||
|
||
1. Begin performance recording in devtools. | ||
1. Click a button for test. | ||
1. Stop performance recording. | ||
|
||
Note that times reported in web page are total time for a test function (including other operations). In order to verify which methods is fastest always look in on the "total time" of each Position constructor stubs. |
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Why this change? I thought myself that
last()
is probably slow, but I didn't see any change in results.There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I don't see a need here for using an external library which involves loading an external library for this. Also for other path operations, we do not use libs for simple tasks in
Position
elsewhere.The
last
helper does:and we should never have
Position
withoutthis.path
.Calling external method will be slower. It is arguable if this is an important gain. Here I get 195.7ms (1.1%) down to 2.3 ms (0.0%). In a total of ~20s (long semantic + undo). So it is arguable if this change is necessary - I'm for this change.
Current:
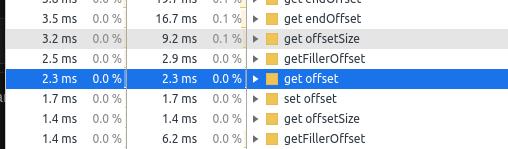
Current with reverting to
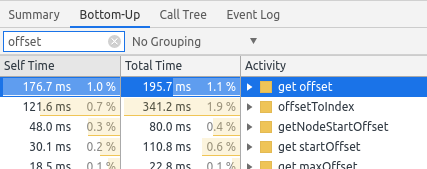
last
.TL;DR: Let's keep it - using
last()
makes no sense.