-
-
Notifications
You must be signed in to change notification settings - Fork 34
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
add semanticHighlighting support #27
Conversation
Hey @fannheyward, this is awesome, the highlighting looks really good already. So about the missing fields:
|
This is awesome!
OOC, what's the intended semantics here? All occurrences of a var get the same ID to allow each var to be a different color? We discussed adding this as an extension to clangd, and could do this if it seems popular.
Our read of the highlighting proposal we implemented was: imagine an infinite array containing the highlightings for each line number. Initially, each line has no highlightings: This means:
Note that the LSP 3.16 proposal has a different delta scheme that seems a lot more sensible (delta-encoding for lines/chars of successive tokens).
Outside the scope of this patch, but my read of the LSP 3.16 proposal is that it actually enables clients to only do work proportional to the tokens actually changed in most cases. I.e. given a small edit, |
In case it's interesting/useful, I've prepared a very simple implementation of the LSP 3.16 protocol for clangd: https://reviews.llvm.org/D76663 (No support yet for sending deltas only) |
Are you referring to the new proposal or the current one? For Vim/Neovim the current proposal is supported by vim-lsp and LanguageClient-neovim (implemented by me). For servers the only ones that I know of are clangd (9+) and eclipse.jdt.ls. For In terms of performance, currently its not a concern as often it takes significantly longer for the language server to perform analysis than it takes for vim-lsp-cxx-highlight to apply highlighing. But I may revisit this after the new standard is ready. |
Oh nice, I wasn't aware other clients were using it. OK, then we may have to keep around support for both protocols for a while, as I don't think it's safe to assume everyone has autoupdate for their extensions.
Great, no rush for us to worry about these then. Protocol-wise, I'll try to get basic support for the new LSP protocol landed soon, since it's the future. It's missed the cut for clangd 10. I'd expect clangd 11 to support both, and clangd 12 to maybe drop support for the old/theia extension. |
I'd like to add this old protocol |
fix skipped ranges
sorry to spam this closed PR - @fannheyward do you know if the current implementation supports clangd-10 for inactive regions in particular ? The release notes for clangd indicate that inactive region support is included, but I can't seem to get it to work. If I enable ccls then inactive regions are shown, so I'm thinking there might be a subtle protocol difference. Thoughts? |
cc @sam-mccall |
the extension seems to be sending necessary notifications in https://github.com/clangd/coc-clangd/pull/27/files#diff-95f1bf2920f2b2f4cd79be925f7acae9R99 and inactive region support is included in clangd-10 as release notes indicate (it was landed in late september, and release was in Feb). |
I'm working on
semanticHighlighting
on coc-clangd, here is a demo:test.cpp
Without semantic highlighting:
With semantic highlighting + vim-lsp-cxx-highlight
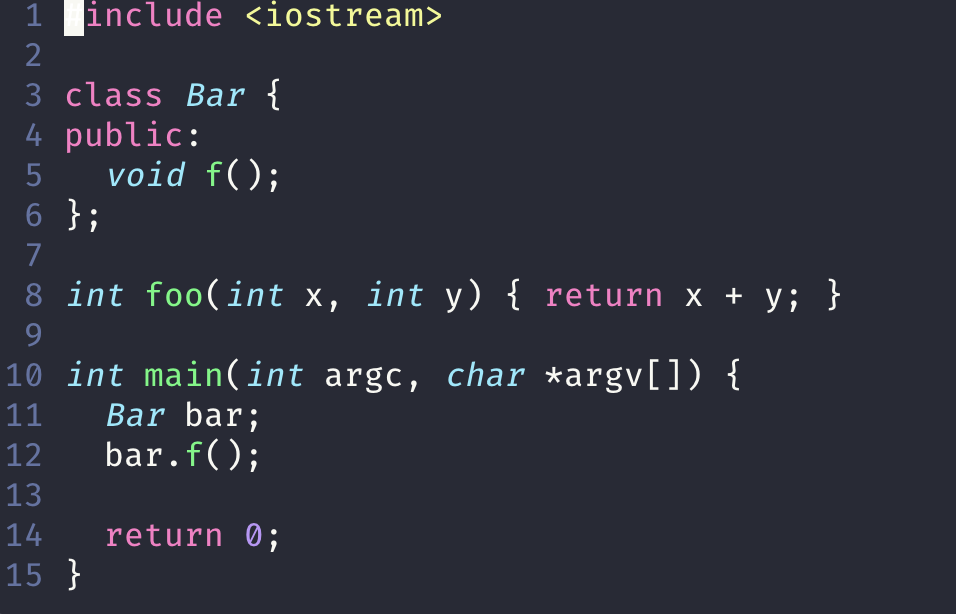
Still needs some improvement: currently, I just parse semantic tokens from clangd to vim-lsp-cxx-highlight's symbol format, then call
lsp_cxx_hl#hl#notify_symbols
to do highlight. For example,clangd
's token:to
But I can't get
id
,storage
andparentKind
from clangd's token, I just set toUnknown
and None. Anyone can help me to correct the token format?