-
-
Notifications
You must be signed in to change notification settings - Fork 188
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Browse files
Browse the repository at this point in the history
Closes #2437 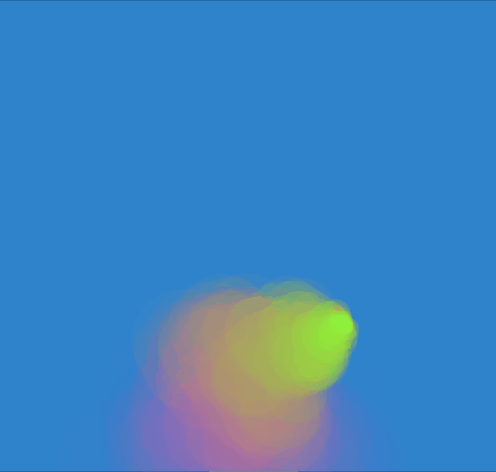 ## Changes: - Adds a `particleTransform` parameter either `Global` or `Local`, `Global` was the implicit behavioral default before these changes and is not the explicit default. `Local` generates particles as children of the emitter which can be useful. - Uses the global position when generating particles by default
- Loading branch information
Showing
8 changed files
with
232 additions
and
5 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,13 @@ | ||
<!DOCTYPE html> | ||
<html lang="en"> | ||
<head> | ||
<meta charset="UTF-8"> | ||
<meta http-equiv="X-UA-Compatible" content="IE=edge"> | ||
<meta name="viewport" content="width=device-width, initial-scale=1.0"> | ||
<title>Emitter</title> | ||
</head> | ||
<body> | ||
<script src="../../lib/excalibur.js"></script> | ||
<script src="./index.js"></script> | ||
</body> | ||
</html> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,49 @@ | ||
|
||
var game = new ex.Engine({ | ||
width: 400, | ||
height: 400 | ||
}); | ||
|
||
var actor = new ex.Actor({ | ||
anchor: ex.vec(0.5, 0.5), | ||
pos: game.screen.center, | ||
color: ex.Color.Red, | ||
radius: 5, | ||
}); | ||
|
||
actor.actions.repeatForever(ctx => { | ||
ctx.moveBy(ex.vec(100, 100), 100); | ||
ctx.moveBy(ex.vec(-100, -100), 100); | ||
}); | ||
|
||
var emitter = new ex.ParticleEmitter({ | ||
width: 10, | ||
height: 10, | ||
emitterType: ex.EmitterType.Rectangle, | ||
particleTransform: ex.ParticleTransform.Global, | ||
radius: 5, | ||
minVel: 100, | ||
maxVel: 200, | ||
minAngle: 5.1, | ||
maxAngle: 6.2, | ||
emitRate: 300, | ||
opacity: 0.5, | ||
fadeFlag: true, | ||
particleLife: 1000, | ||
maxSize: 10, | ||
minSize: 1, | ||
startSize: 5, | ||
endSize: 100, | ||
acceleration: ex.vec(10, 80), | ||
beginColor: ex.Color.Chartreuse, | ||
endColor: ex.Color.Magenta | ||
}); | ||
emitter.isEmitting = true; | ||
|
||
game.start(); | ||
game.add(actor); | ||
|
||
actor.angularVelocity = 2; | ||
|
||
// doesn't work | ||
actor.addChild(emitter); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.