-
Notifications
You must be signed in to change notification settings - Fork 24.1k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Export the DevSettings module, add
addMenuItem
method (#25848)
Summary: I wanted to configure the RN dev menu without having to write native code. This is pretty useful in a greenfield app since it avoids having to write a custom native module for both platforms (and might enable the feature for expo too). This ended up a bit more involved than planned since callbacks can only be called once. I needed to convert the `DevSettings` module to a `NativeEventEmitter` and use events when buttons are clicked. This means creating a JS wrapper for it. Currently it does not export all methods, they can be added in follow ups as needed. ## Changelog [General] [Added] - Export the DevSettings module, add `addMenuItem` method Pull Request resolved: #25848 Test Plan: Tested in an app using the following code. ```js if (__DEV__) { DevSettings.addMenuItem('Show Dev Screen', () => { dispatchNavigationAction( NavigationActions.navigate({ routeName: 'dev', }), ); }); } ``` Added an example in RN tester 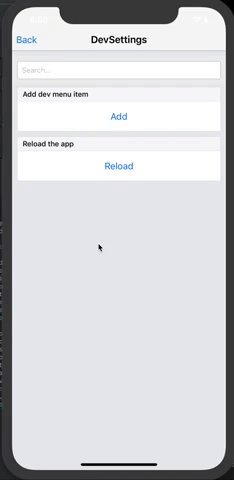 Differential Revision: D17394916 Pulled By: cpojer fbshipit-source-id: f9d2c548b09821c594189d1436a27b97cf5a5737
- Loading branch information
1 parent
1534386
commit cc068b0
Showing
15 changed files
with
190 additions
and
22 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,52 @@ | ||
/** | ||
* Copyright (c) Facebook, Inc. and its affiliates. | ||
* | ||
* @format | ||
*/ | ||
|
||
import NativeDevSettings from '../NativeModules/specs/NativeDevSettings'; | ||
import NativeEventEmitter from '../EventEmitter/NativeEventEmitter'; | ||
|
||
class DevSettings extends NativeEventEmitter { | ||
_menuItems: Map<string, () => mixed>; | ||
|
||
constructor() { | ||
super(NativeDevSettings); | ||
|
||
this._menuItems = new Map(); | ||
} | ||
|
||
addMenuItem(title: string, handler: () => mixed) { | ||
// Make sure items are not added multiple times. This can | ||
// happen when hot reloading the module that registers the | ||
// menu items. The title is used as the id which means we | ||
// don't support multiple items with the same name. | ||
const oldHandler = this._menuItems.get(title); | ||
if (oldHandler != null) { | ||
this.removeListener('didPressMenuItem', oldHandler); | ||
} else { | ||
NativeDevSettings.addMenuItem(title); | ||
} | ||
|
||
this._menuItems.set(title, handler); | ||
this.addListener('didPressMenuItem', event => { | ||
if (event.title === title) { | ||
handler(); | ||
} | ||
}); | ||
} | ||
|
||
reload() { | ||
NativeDevSettings.reload(); | ||
} | ||
|
||
// TODO: Add other dev setting methods exposed by the native module. | ||
} | ||
|
||
// Avoid including the full `NativeDevSettings` class in prod. | ||
class NoopDevSettings { | ||
addMenuItem(title: string, handler: () => mixed) {} | ||
reload() {} | ||
} | ||
|
||
module.exports = __DEV__ ? new DevSettings() : new NoopDevSettings(); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,47 @@ | ||
/** | ||
* Copyright (c) Facebook, Inc. and its affiliates. | ||
* | ||
* This source code is licensed under the MIT license found in the | ||
* LICENSE file in the root directory of this source tree. | ||
* | ||
* @format | ||
* @flow | ||
*/ | ||
|
||
'use strict'; | ||
|
||
import * as React from 'react'; | ||
import {Alert, Button, DevSettings} from 'react-native'; | ||
|
||
exports.title = 'DevSettings'; | ||
exports.description = 'Customize the development settings'; | ||
exports.examples = [ | ||
{ | ||
title: 'Add dev menu item', | ||
render(): React.Element<any> { | ||
return ( | ||
<Button | ||
title="Add" | ||
onPress={() => { | ||
DevSettings.addMenuItem('Show Secret Dev Screen', () => { | ||
Alert.alert('Showing secret dev screen!'); | ||
}); | ||
}} | ||
/> | ||
); | ||
}, | ||
}, | ||
{ | ||
title: 'Reload the app', | ||
render(): React.Element<any> { | ||
return ( | ||
<Button | ||
title="Reload" | ||
onPress={() => { | ||
DevSettings.reload(); | ||
}} | ||
/> | ||
); | ||
}, | ||
}, | ||
]; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.