-
Notifications
You must be signed in to change notification settings - Fork 5.9k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
[Windows] Don't block raster thread until v-blank (#41231)
Currently the Windows raster thread blocks until the v-blank. As a result, on Windows a frame can spend 17ms on the raster thread even if rasterization completes in under 1ms. This behavior prevents us from rendering multiple views on the same raster thread on Windows. This change does not make rasterization faster. Instead, it allows the raster thread to do more work. Addresses flutter/flutter#124903 ### Results Here are the performance graphs if I hover on and off the counter app's button repeatedly. #### Before The raster thread spends ~17ms per frame consistently: 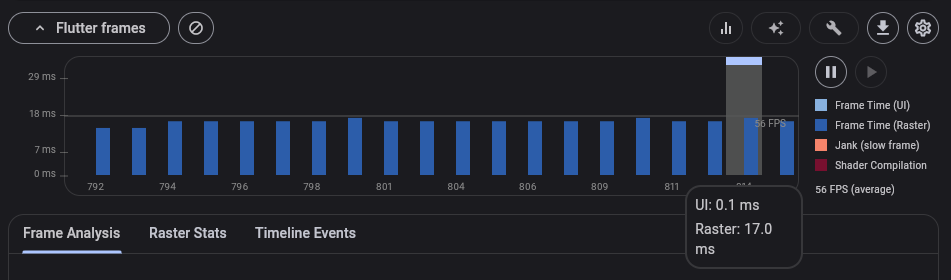 #### After After this change, the raster thread spends less than 1ms per frame consistently: 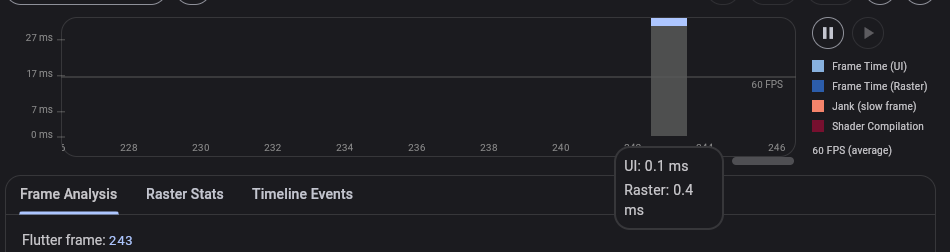 ### Background Blocking until the v-blank prevents screen tearing if the OS does not synchronize to the vsync. Windows does synchronize if the Desktop Windows Manager composition is enabled, which is required on Windows 8 and newer. In other words, this blocking behavior is unnecessary for Windows 8 and newer. For Windows 7, screen tearing is possible if DWM composition is disabled (either by the user or by an app). In this scenario, the Flutter app should block until the v-blank to synchronize with the vsync and prevent screen tearing. We can detect if DWM composition is disabled using [`DwmIsCompositionEnabled`](https://learn.microsoft.com/en-us/windows/win32/api/dwmapi/nf-dwmapi-dwmiscompositionenabled). We can detect changes to DWM composition using the top-level [`WM_DWMCOMPOSITIONCHANGED`](https://learn.microsoft.com/en-us/windows/win32/dwm/wm-dwmcompositionchanged) Windows message. Useful resources: 1. https://learn.microsoft.com/en-us/windows/win32/dwm/composition-ovw 2. https://www.khronos.org/opengl/wiki/Swap_Interval 3. https://forums.imgtec.com/t/eglswapinterval-0-tearing-problem/2356/5 4. https://stackoverflow.com/questions/32282252/can-eglswapinterval0-cause-screen-tearing 5. https://bugs.chromium.org/p/chromium/issues/detail?id=480361
- Loading branch information
1 parent
a2b02b8
commit 122c3b3
Showing
13 changed files
with
214 additions
and
25 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.