Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
[Impeller] Fix division by zero for transparent shadows (flutter#41391)
A division by zero happens if the shadow color is fully transparent, and the NaNs are getting assigned to the RGB values of the paint color being handed to the rrect shadow draw. This doesn't cause a problem when wide gamut is off because NaN output ends up being interpreted as zero (thus making the shadow output fully transparent black, which happens to be the expected and correct result). However, when a wide gamut attachment is present, the NaN output ends up being interpreted as a negative value. Reproduction app: ```dart import 'package:flutter/material.dart'; void main() => runApp(const GeneralDialogApp()); class EvilPainter extends CustomPainter { @OverRide void paint(Canvas canvas, Size size) { final Rect rect = Offset.zero & size; canvas.drawPaint(Paint()..color = Colors.white); canvas.saveLayer(null, Paint()..blendMode = BlendMode.srcOver); canvas.drawShadow(Path()..addRect(Rect.fromLTRB(100, 100, 300, 300)), Colors.black54, 15, false); canvas.drawShadow(Path()..addRect(Rect.fromLTRB(100, 100, 300, 300)), Colors.black54, 15, false); canvas.drawShadow(Path()..addRect(Rect.fromLTRB(100, 100, 300, 300)), Colors.transparent, 15, false); canvas.restore(); } @OverRide bool shouldRepaint(EvilPainter oldDelegate) => false; @OverRide bool shouldRebuildSemantics(EvilPainter oldDelegate) => false; } class GeneralDialogApp extends StatelessWidget { const GeneralDialogApp({super.key}); @OverRide Widget build(BuildContext context) { return MaterialApp( restorationScopeId: 'app', home: CustomPaint(painter: EvilPainter()), ); } } ``` Before: 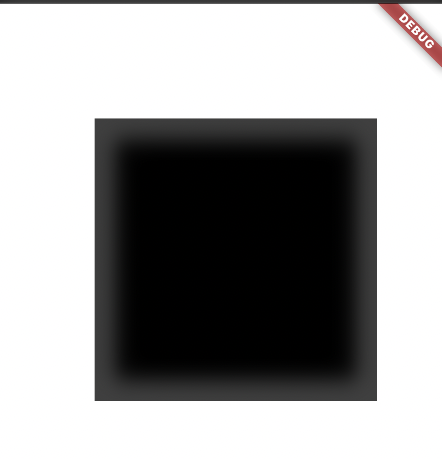 After: 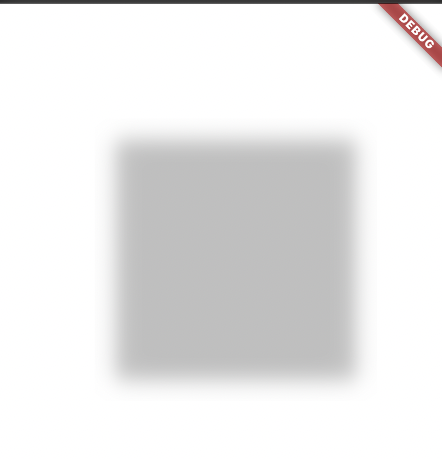
- Loading branch information