-
Notifications
You must be signed in to change notification settings - Fork 11
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[Request] Some additional stylings #4
Comments
Thanks for the feedback. I'll try to respond to all your comments:
This is not something that the formatter should do by default as the goal is formatting without altering text as much as possible. That said, you have the option to set
This is a fair request and I would look into it, but it will complicate the implementation so I don't think this will happen any time soon.
The uppercase "usage" can be done now by overriding the
This is not related to the formatter. The formatter just uses whatever group names you pass to it. The solution IMO is to create your own group with the name you want (see example below). Another solution is to ask CPython to change the default in argparse (If I remember correctly, someone already did it when they change the default group name from
This is considered altering the structure of the help text, so I can't have the formatter do that. The formatter is only meant to style the help text in a better way than the default formatters provided by argparse without altering its structure. However, what you want is also available by overriding the import argparse
from rich_argparse import RichHelpFormatter
prog_name = 'substoforced'
prog_version = '1.0.1'
version_text = f'[bold cyan]{prog_name}[/bold cyan] [not bold white]{prog_version}[/not bold white]'
class CustomHelpFormatter(RichHelpFormatter):
def add_usage(self, usage, actions, groups, prefix=None) -> None:
self.add_text(version_text) # <-- print version before help
if prefix is None:
prefix = 'USAGE: ' # <-- "usage" prefix with same formatting as the groups
return super().add_usage(usage, actions, groups, prefix=prefix)
CustomHelpFormatter.group_name_formatter = lambda name: name.upper() + ':' # <-- custom group name formatting
CustomHelpFormatter.styles['argparse.text'] = 'default' # <-- if you don't want the default (italic)
parser = argparse.ArgumentParser(
prog_name,
add_help=False,
formatter_class=CustomHelpFormatter
)
flags_group = parser.add_argument_group('flags') # <-- custom group replacing "options"
flags_group.add_argument('-h', '--help',
action='help',
default=argparse.SUPPRESS,
help='show this help message.')
flags_group.add_argument('-v', '--version',
action='version',
version=version_text,
help='show version.')
flags_group.add_argument('-s', '--sub',
default=argparse.SUPPRESS,
help='specifies srt input')
flags_group.add_argument('-f', '--folder',
metavar='DIR',
default=None,
help='specifies a folder where [bold color(231)]SubsMask2Img[/bold color(231)] generated timecodes (optional)\nyou should remove the junk from there manually')
args = parser.parse_args() |
thanks to @ pcroland for the feedback in #4
I'm looking forward for the updates. Wouldn't it be possible that the library was a replacement for argparse instead of a replacement for it's helpformatter? It would give the users more control over what can be customized and probably easier syntaxes. For example you could do something like this: parser = rparse.ArgumentParser(
prog_name,
add_help=False,
syntax_color=xy,
metavar_color=etc
...
) I also have another feature idea. I know it's a little over the top but it would be visually pleasing. The idea is to allow the long options to align with other long options when you have short options with different length. For example you could align them by allowing extra spaces. If you were to set the allowed extra spaces to one, then this:
would become this:
|
No, I wouldn't add yet another library for CLI argument parsing.
Sorry but I don't think I will add this. I would like to keep the implementation as simple as possible and this would complicate things. Also, using multiple chars in short options is against the posix conventions as it messes with stacking short options together (i.e. somthing like P.S. I might have some free time this weekend to look into your request regarding metavar formatting |
I got the metavar coloring adjustable with |
i've been doing some kind of crazy stuff with These are just opinions and they're mostly about the default config. I know i can adjust it but a) first impressions are important and b) most potential users will not adjust the defaults. Springboarding them with some good design would probably go a long way towards improving the usefulness of this project as well as its adoption.
i'll try to come up with a screenshot of tweaks to the defaults along these lines; easier to discuss visual stuff w/visuals Footnotes
|
Thanks for the feedback @michelcrypt4d4mus. To answer your questions:
|
before i respond to any individual points i will throw out there that my python and beyond that just to offer some more feedback/ideas that you should feel free to ignore:
|
Thanks, probably the easiest way to get a dev env is using
|
been messing around with some color themes/tweaks; feel free to take a look (i think this one is my favorite) occurred to me that offering a couple of default options as far as theming could be cool |
@pcroland I have implemented a bunch of stuff that may interest you, It is still not published on PyPI yet but you can test it with These are all now implemented (and the default)
With this version, your example roughly becomes import argparse
from rich_argparse import RichHelpFormatter
version = "1.0.1"
version_text = f"[bold cyan]%(prog)s[/] {version}"
class CustomHelpFormatter(RichHelpFormatter):
def add_usage(self, usage, actions, groups, prefix=None) -> None:
self.add_text(version_text)
return super().add_usage(usage, actions, groups, prefix=prefix)
CustomHelpFormatter.styles["argparse.args"] = "green"
CustomHelpFormatter.styles["argparse.groups"] = "yellow"
CustomHelpFormatter.styles["argparse.metavar"] = "bold color(231)"
parser = argparse.ArgumentParser(
"substoforced", add_help=False, formatter_class=CustomHelpFormatter
)
flags_group = parser.add_argument_group("flags")
flags_group.add_argument("-h", "--help", action="help", help="show this help message.")
flags_group.add_argument(
"-v", "--version", action="version", version=version_text, help="show version."
)
flags_group.add_argument("-s", "--sub", default=argparse.SUPPRESS, help="specifies srt input")
flags_group.add_argument(
"-f",
"--folder",
metavar="DIR",
default=None,
help=(
"specifies a folder where [bold color(231)]SubsMask2Img[/] generated timecodes (optional)"
"\nyou should remove the junk from there manually"
),
)
args = parser.parse_args() I'll close the issue but feel free to reopen it if needed; Thanks for the feedback |
Nice, thanks for the update, it works great. I'm going to update my stuff with it once it's on PyPI. |
Hi!
First of all, thank you for this library. It's really promising. This is what I currently use:
With this I get a result like this:
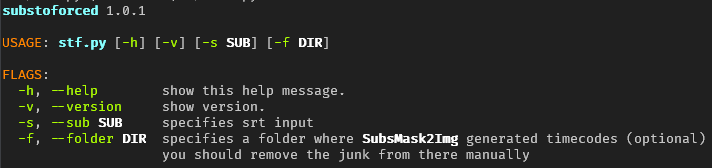
I don't think all of these is currently possible with rich-argparse, but it would be great. These are the missing pieces compared to this (at least I think):
The text was updated successfully, but these errors were encountered: