Country List (RecyclerView)
Harsh B. Bhakta edited this page Aug 6, 2020
·
5 revisions
If Country Picker View or Country Picker Dialog is not enough for you and you want to load country list in your RecyclerView then you can do it using extension function (todo: add link) or CPRecyclerViewHelper (todo: add link).
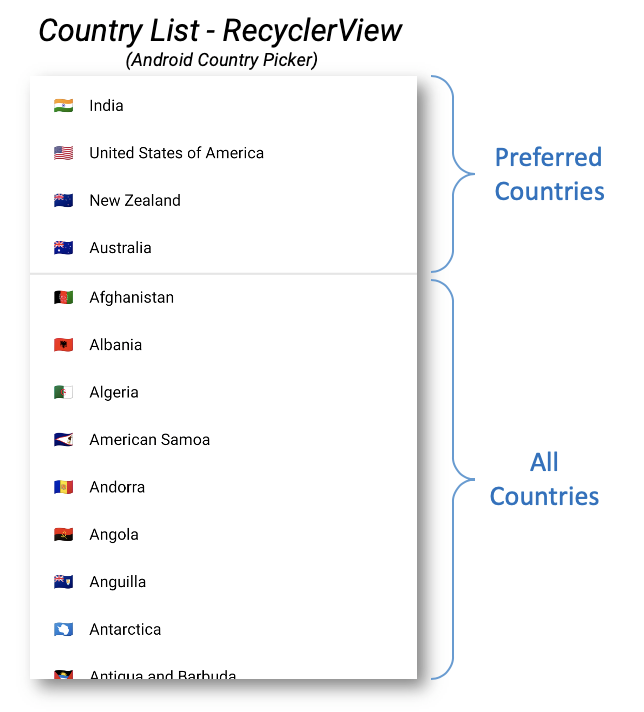
Todo: add table
Launch using Extension function (source)
With Kotlin in action, you can load list of countries in your recycler view by simply adding,
recyclerView.loadCountries { selectedCountry: CPCountry ->
// your code to handle selected country
}
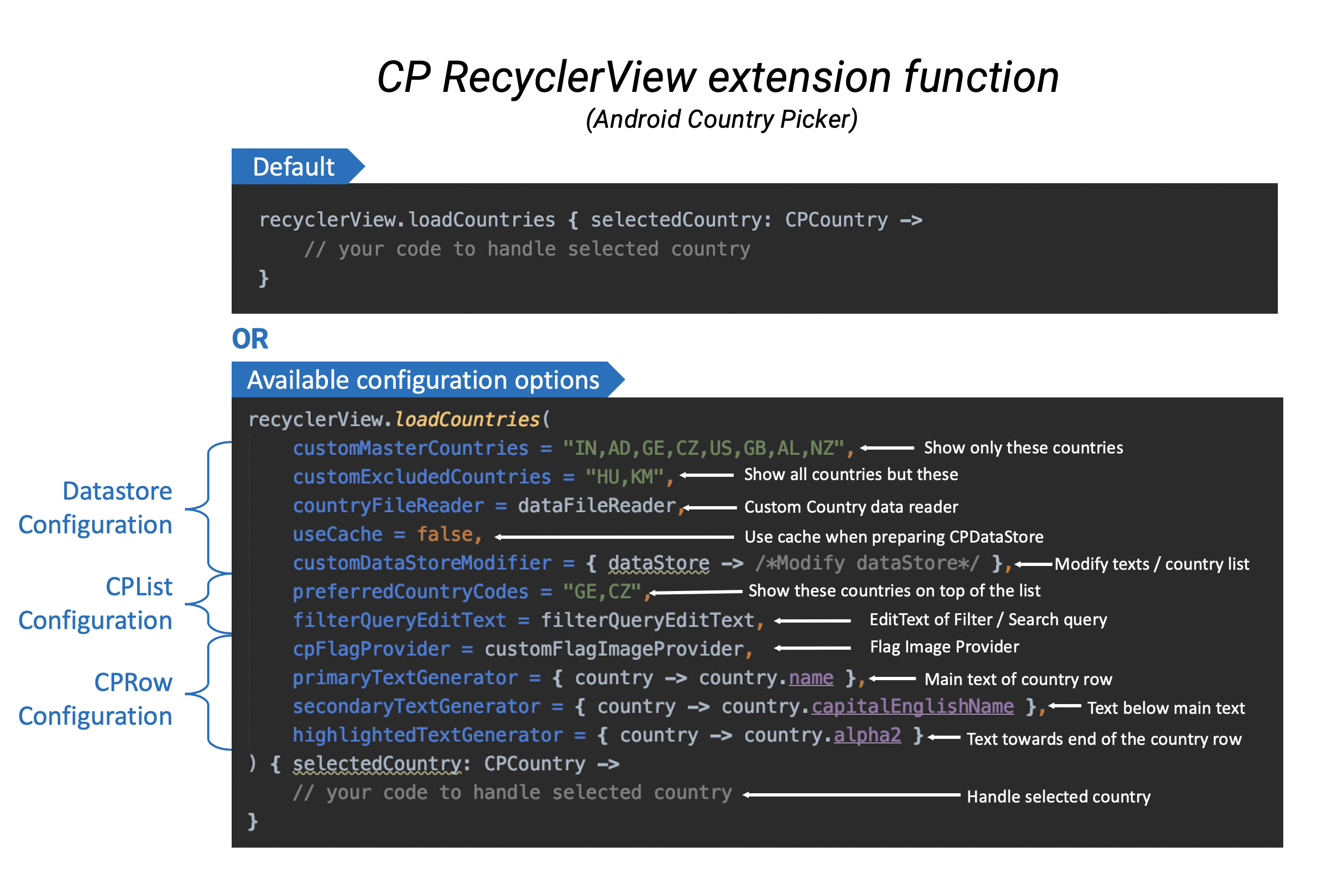
Configuration - CPListConfig
(source)
- Preferred Countries
- These are the countries shown on top of the list. If you know your users are coming from specific country/countries or they are likely to select from specific countries then it's a good idea to put them on top of the list.
- By default, preferred countries is
null
so no country is shown on top of the regular list. - Preferred countries can be passed using their Alpha2 / Alpha3 codes.
- Example: **
- to put India (IN), United States of America (US), New Zealand (NZ) and Austrelia (AU) in Preferred Country List add following:
val cpListConfig = CPListConfig( preferredCountryCodes = "IN,US,NZ,AU" )
- See CountryDataStore (todo: add link) to configure list of "All Countries"
- See Country Row page to configure individual row of country
Launch using CPRecyclerViewHelper
(source)
To load country list using Extension function (todo: add link) keeps your code clean. If you want more granular control over instance creation, then you can do following:
// Check CPDataStoreGenerator for available configuration
val cpDataStore = CPDataStoreGenerator.generate(context)
// Check CPListConfig for available configuration
val cpListConfig = CPListConfig()
// Check CPRowConfig for available configuration
val cpRowConfig: CPRowConfig = CPRowConfig()
val cpRecyclerViewHelper = CPRecyclerViewHelper(
cpDataStore = cpDataStore, // required
cpListConfig = cpListConfig, // Default: CPListConfig()
cpRowConfig = cpRowConfig // Default: CPRowConfig()
){ selectedCountry: CPCountry ->
// required: handle selected country
}
// attach recyclerView to show list in recyclerView
cpRecyclerViewHelper.attachRecyclerView(recyclerView)
// attach query edit text (optional) to update filtered list when query updated
cpRecyclerViewHelper.attachFilterQueryEditText(filterQueryEditText)