-
-
Notifications
You must be signed in to change notification settings - Fork 26
Country Picker View
Harsh B. Bhakta edited this page Sep 2, 2020
·
5 revisions
Country picker (CP) view is an easy way to integrate country picker in your project.
Here is the simplest XML
<com.hbb20.CountryPickerView
android:id="@+id/countryPicker"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<com.hbb20.CountryPickerView
android:id="@+id/countryPicker"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:cp_initialSelectionMode="empty/specificCountry/autoDetectCounty"
app:cp_initialSpecificCountry="alpha2/alpha3"
app:cp_autoDetectSources="LOCALE_NETWORK_SIM"
app:cpDialog_allowClearSelection="true/false"
app:cpDialog_allowSearch="true/false"
app:cpDialog_showFullScreen="true/false"
app:cpDialog_showTitle="true/false"
app:cpList_preferredCountryCodes="comma separated alpha2/alpha3"
/>
CPViewConfig Source
initialSelection
- Initial selection value can be
EmptySelection
,AutoDetectCountry()
,SpecificCountry(alphaCode)
-
XML
Add following attributes to configure initial selection-
Empty (empty selection) - Default
- Add following
app:cp_initialSelectionMode="empty"
to XML -
- Initial selected country will be
null
and place holder text will be shown - To override this placeholder text, add a string resource with name=
cp_selection_placeholder
(Default value = "Country") -
- Add following
-
AutoDetect (Detect country)
- Add following
app:cp_initialSelectionMode="autoDetectCounty"
to XML - CP can detect country using SIM / NETWORK / LOCALE.
- Default order for detection is SIM_NETWORK_LOCALE (Means first it will try to detect country using SIM. If it fails, then try from NETWORK. If it fails, then try from LOCALE.)
-
- Detection source order can be changed using
app:cp_autoDetectSources="SIM_ONLY"
(Any combination can be selected)
- Add following
-
Specific Country (A particular country)
- If you want to set a specific country initially then add following
XML that will set the initial mode and specify alpha2 / alpha3 code of the country
app:cp_initialSelectionMode="specificCountry" app:cp_initialSpecificCountry="IN"
-
- If you want to set a specific country initially then add following
-
Empty (empty selection) - Default
viewTextGenerator
- Lambda that takes
CPCountry
in and returns aString
to show on CountryPickerView - By default it returns
cpCountry.name
cpFlagProvider
- This is the flag provider for country. If you want to use custom flag images, pass your customFlagProvider (extended from
CPFlagImageProvider
) for this property -
DefaultEmojiFlagProvider
is the default flag provider. - Flag is hidden when
flagProvider = null
private fun setupCountryPickerView() {
val countryPicker = findViewById<CountryPickerView>(R.id.countryPicker)
// Modify CPViewConfig if you need. Access cpViewConfig through `cpViewHelper`
countryPicker.cpViewHelper.cpViewConfig.viewTextGenerator = { cpCountry: CPCountry ->
"${cpCountry.name} (${cpCountry.alpha2})"
}
// make sure to refresh view once view configuration is changed
countryPicker.cpViewHelper.refreshView()
// Modify CPDialogConfig if you need. Access cpDialogConfig through `countryPicker.cpViewHelper`
// countryPicker.cpViewHelper.cpDialogConfig.
// Modify CPListConfig if you need. Access cpListConfig through `countryPicker.cpViewHelper`
// countryPicker.cpViewHelper.cpListConfig.
// Modify CPRowConfig if you need. Access cpRowConfig through `countryPicker.cpViewHelper`
// countryPicker.cpViewHelper.cpRowConfig.
}
-
Listen through
LiveData
countryPickerView.cpViewHelper.selectedCountry.observe( lifecycleOwner, Observer { selectedCountry: CPCountry? -> // your code to handle selected country } )
-
Listen through
callback
cpViewHelper.onCountryChangedListener = { selectedCountry: CPCountry? -> // your code to handle selected country }
- Access child views to modify them for your needs
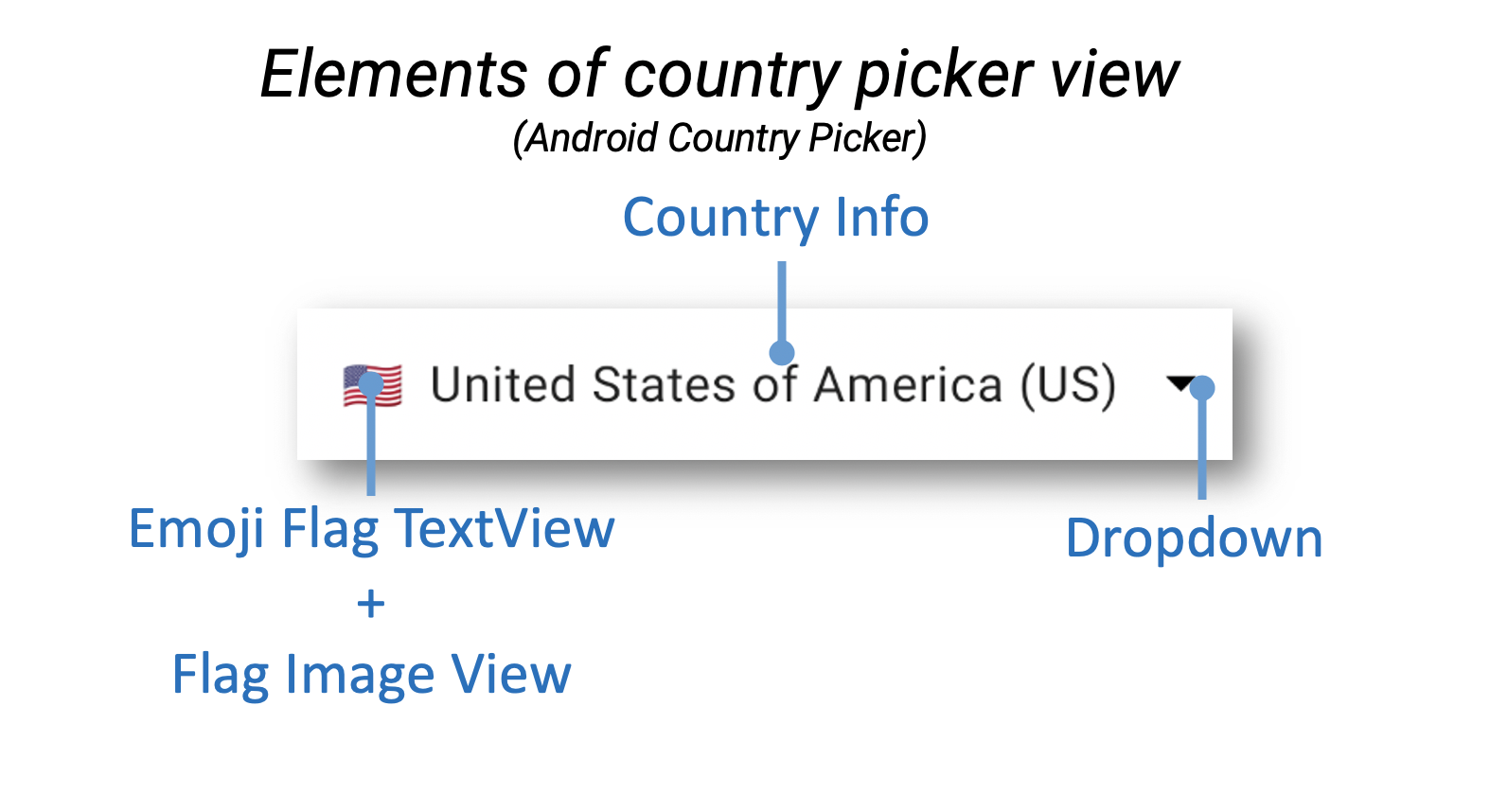
- Country Info TextView :
countryPickerView.tvCountryInfo
- Dropdown ImageView :
countyPickerView.imgDropDownIcon
- Emoji Flag TextView (For Emoji Flags):
countyPickerView.tvEmojiFlag
- Flag ImageView (For Flag Images):
countyPickerView.imgFlag