-
-
Notifications
You must be signed in to change notification settings - Fork 2.8k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Strongly Typed Formik, v3 #3152
base: milestone/v3
Are you sure you want to change the base?
Strongly Typed Formik, v3 #3152
Conversation
Added Ref State. Added useSelectorComparer. Starting to build subscriptions.
…ohnrom/subscriber
use-subscriptions might not work in React 17? Seems returning the previous value doesn't bail out the render.
Sync up formik-native and formik for v3.
…te will do. If we call our own reducer then use useReducer dispatch, `state.values !== getState().values`.
…ormikReducerState + FormikComputedState. Add Fixtures and Tutorial code to /app.
Consolidate State and Add Tutorial + Fixtures.
For some reason, the tests for remove only worked when they were async or render was moved from beforeEach to the individual tests.
Published |
Seems that recently yup moved to have built-in typscript support https://github.com/jquense/yup/blob/HEAD/docs/typescript.md https://www.npmjs.com/package/yup shows as typescript package: 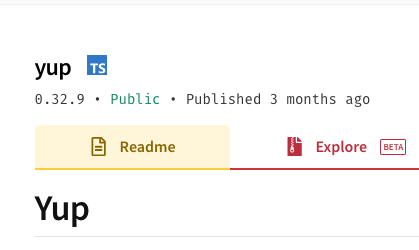
Co-authored-by: github-actions[bot] <github-actions[bot]@users.noreply.github.com>
setError is setFieldError bound to specific field. Therefore the setError value should have same type as setFieldError message
Co-authored-by: github-actions[bot] <github-actions[bot]@users.noreply.github.com>
Adds `yarn.lock` and `changeset` to jaredpalmer#3081 closes jaredpalmer#3062 closes jaredpalmer#3225
Co-authored-by: github-actions[bot] <github-actions[bot]@users.noreply.github.com>
…pdate it instead.
# Conflicts: # packages/formik/src/Formik.tsx # packages/formik/src/types.tsx
This pull request is stale because it has been open 30 days with no activity. Remove stale label or comment or this will be closed in 60 days |
# Conflicts: # packages/formik/src/Formik.tsx # packages/formik/src/types.tsx
…in one place between field.onChange and Formik.
Parsing Refactor
This pull request is stale because it has been open 30 days with no activity. Remove stale label or comment or this will be closed in 60 days |
…types-v3 # Conflicts: # app/package.json # packages/formik-native/package.json # packages/formik/package.json # packages/formik/src/Formik.tsx # packages/formik/src/hooks/hooks.ts # packages/formik/src/types.tsx
Adds strong types to Formik by typing Fields with
Field<Value, FormValues>
. This is an extremely breaking change. You can view only the changes related to this PR here: THESE CHANGESOpting-in to Types
createTypedField<YourFormValuesType>()
(anywhere)useTypedField<YourFormValuesType>()
(in component) (ok they're the same but you'll feel safer if you use a hook in your component, plus we could optimize later)<Field<number, YourFormValuesType> />
Using *TypedField()
Using useTypedField or createTypedField above, you can let the component know which Values object to look through so you can get automatic inference when passing a component or path to your field, like:
A Custom Field
Alternatively, you can create a custom field which accepts
FieldConfig<number, Values>
, like this. I find this to be the easiest to use and most flexible.Use both!
MyNumberField can call
<Field as={MyNumberAsComponent} />
It's a little bit confusing from there, but can be useful if you use your custom field as a "connector" between Formik and your raw input component.Examples
Check out every possible variant here: https://codesandbox.io/s/serene-napier-4wjxs?file=/src/App.tsx
How does it work
This PR separates the different types of possible Field configs into separate types, then tries to match
name
based on theValue
configured for a given Field. It also allows the Value to be inferred from thename
ifFormValues
is known and there are no explicit usages ofValue
. The different Field configurations are:Todo
ExtraProps
.type oneOf = interface IA extends A, B, C {} | interface IB extends A, B, D {}
is fast, buttype oneOf = type (A & B & C) | type (A & B & D)
is quite slow.PathMatchingValue<number, Values>
is not extensible to paths matchingnumber | ""
.setFieldValue<number>(field: PathMatchingValue<number | "">, value: number)
cannot be inferred, must manually callsetFieldValue<number | "">
.rows
. Previously this would be inferred by ExtraProps butas="textarea"
doesn't have extra props.ExtraProps
The following can be worked around by simply using untyped variants of these complex fields
${props.path}.${index}
withValueMatchingPath<number, ValueMatchingPath<Path, Values>>
which is a very complicated way of saying(infer Value)[]
.select multiple
works.