-
Notifications
You must be signed in to change notification settings - Fork 1
attachable_text3d.scad
OpenSCAD module for creating blocks of 3D text that are attachable with BOSL2.
To use, add the following lines to the beginning of your file:
include <openscad_attachable_text3d/attachable_text3d.scad>
You must additionally have the fontmetrics.scad
and fontmetricsdata.scad
libraries by Alexander Pruss installed. Source these from
https://www.thingiverse.com/thing:3004457.
These libraries are available under a CC-BY-4.0 license.
-
Section: Attachable Text Modules
-
attachable_text3d()
– Creates an attachable 3D model of text -
attachable_text3d_multisize()
– Creates an attachable 3D model of text with multiple sizes
-
-
-
attachable_text3d_boundary()
– Returns a size listing of a given block of attachable text -
attachable_text3d_multisize_boundary()
– Returns a size listing of a given block of attachable multi-sized text
-
-
-
AT3D_DEFAULT_FONT
– Default font name: Liberation Sans -
AT3D_DEFAULT_SIZE
– Default font size: 10 -
AT3D_DEFAULT_HEIGHT
– Default font height: 1 -
AT3D_DEFAULT_PAD
– Default padding to surround attachable text: 0 -
AT3D_DEFAULT_LINE_SPACING
– Default spacing between lines: 0.5 -
AT3D_DEFAULT_ALIGNMENT
– Default alignment: LEFT -
AT3D_DEFAULT_SPACING
– Default spacing: 1 -
AT3D_DEFAULT_DIRECTION
– Default text direction: ltr (left-to-right) -
AT3D_DEFAULT_LANGUAGE
– Default text language: en -
AT3D_DEFAULT_SCRIPT
– Default text script: latin -
AT3D_DEFAULT_ANCHOR
– Default attachable positioning anchor: CENTER -
AT3D_DEFAULT_SPIN
– Default attachable spin, in degrees: 0 -
AT3D_DEFAULT_ORIENT
– Default attachable orientation: UP -
AT3D_ATTACHABLE_FONTS
– Sorted list of known fonts
-
Synopsis: Creates an attachable 3D model of text
Usage:
- attachable_text3d(text);
- attachable_text3d(text, <font="Liberation Sans">, <size=10>, <h=1>, <pad=0>, <align=LEFT>, <spacing=1>, <direction="ltr">, <language="en">, <script="latin">, <anchor=CENTER>, <spin=0>, <orient=UP>);
Description:
Given a string of text text
, or a list of strings (also text
), create a single 3D model of that text. The resulting model will have BOSL2 attachable anchor points on it, and can be positioned and attached to as needed.
font
must be a font-name and style listed in AT3D_ATTACHABLE_FONTS
, because those are the fonts for which accurate measurements are available. Font families, or families and styles, may be specified; examples: font="Times New Roman"
, font="Liberation Serif:style=Italic"
, font="Arial:style=Bold Italic"
. When not specified, font
defaults to whatever AT3D_DEFAULT_FONT
is set.
All text is by default aligned to the left. Horizontal alignment can be adjusted by setting align
to one of LEFT
, CENTER
, or RIGHT
.
The anchor bounding box constructed for the text is as wide as the longest single text element; and, as deep as the sum of text heights of each text element; and, the height of h
used. The bounding box for all strings represented within text
can be exposed by setting debug_bounding
to true
.
Arguments:
By Position | What it does |
---|---|
text |
A text string to produce a model of. No default. |
By Name | What it does |
---|---|
font |
The name and style of the font to use. Default: Liberation Sans
|
size |
The font size to produce text at. Default: 10
|
h |
The height (thickness) of the text produced. Default: 1
|
line_spacing |
Sets the spacing between individual lines of text; this is similar (but not identical) to leading. Default: 0.5
|
pad |
Padding applied to the boundary anchor box surrounding the generated text. Default: 0
|
align |
Horizontally align text to one of LEFT , CENTER , or RIGHT . Default: LEFT
|
spacing |
The relative spacing multiplier between characters. Default: 1
|
direction |
The text direction. ltr for left to right. rtl for right to left. ttb for top to bottom. btt for bottom to top. Default: ltr
|
language |
The language the text is in. Default: en
|
script |
The script the text is in. Default: latin
|
debug_bounding |
If set to true , the text model's bounding box will be inscribed around the produced text. Default: false
|
anchor |
Translate so anchor point is at origin [0,0,0] . Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchoring. Default: 0
|
orient |
Vector direction to which the model should point after spin. Default: UP
|
Named Anchors: In addition to the cardinal anchor points provided by BOSL2, attachable_text3d()
vends the following six additional named anchors:
Anchor Name | Position Description |
---|---|
text-left-back |
The back-left most corner, oriented backwards |
text-left-fwd |
The forward-left most corner, oriented forwards |
text-center-back |
The back-center face, oriented backwards |
text-center-fwd |
The forward-center face, oriented forwards |
text-right-back |
The top-right most corner, oriented backwards |
text-right-fwd |
The forward-right most corner, oriented forwards |
Figure 1: Available named anchors:
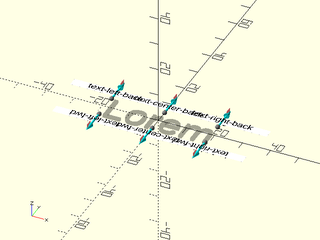
Todo:
- There are no vertical alignment options. I'm not thinking of CSS-level of alignment options, just the basic "bottom/center/top" would do.
Example 1: A single line of attachable text:

include <openscad_attachable_text3d/attachable_text3d.scad>
attachable_text3d("Lorem Ipsum");
Example 2: Multiple lines of attachable text; these all are a single attachable model
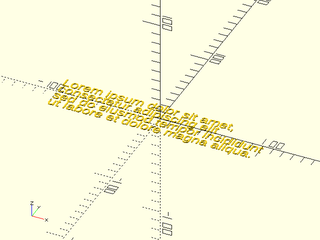
include <openscad_attachable_text3d/attachable_text3d.scad>
attachable_text3d(["Lorem ipsum dolor sit amet,",
"consectetur adipiscing elit",
"sed do eiusmod tempor incididunt",
"ut labore et dolore magna aliqua."]);
Example 3: attaching multiple text blocks together:
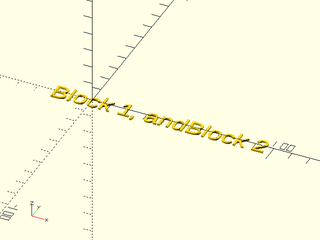
include <openscad_attachable_text3d/attachable_text3d.scad>
attachable_text3d("Block 1")
attach(RIGHT, LEFT)
attachable_text3d(", and ")
attach(RIGHT, LEFT)
attachable_text3d("Block 2");
Example 4: non-default fonts and sizes:

include <openscad_attachable_text3d/attachable_text3d.scad>
attachable_text3d("Be Alert", font="Verdana:style=Bold", size=15)
attach("text-left-fwd", "text-left-back")
attachable_text3d(["The world needs", "more Lerts."], font="Verdana", size=10);
Synopsis: Creates an attachable 3D model of text with multiple sizes
Usage:
- attachable_text3d_multisize(text_and_sizes);
- attachable_text3d_multisize(text_and_sizes, <font="Liberation Sans">, <h=1>, <pad=0>, <align=LEFT>, <spacing=1>, <direction="ltr">, <language="en">, <script="latin">, <anchor=CENTER>, <spin=0>, <orient=UP>);
Description:
Given a list of text and sizing pairings text_and_sizes
, create a single 3D model of that text. Each [text, size]
pairing within text_and_sizes
will be shown in the specified size
, aligned as specified by align
. text
may be a list with multiple elements, all of which will have that pairing's size
applied. The resulting model will have BOSL2 attachable anchor points on it, and can be positioned and attached to as needed.
font
must be a font-name and style listed in AT3D_ATTACHABLE_FONTS
, because those are the fonts for which accurate measurements are available. Font families, or families and styles, may be specified; examples: font="Times New Roman"
, font="Liberation Serif:style=Italic"
, font="Arial:style=Bold Italic"
. When not specified, font
defaults to whatever AT3D_DEFAULT_FONT
is set.
All text is by default aligned to the left. Horizontal alignment can be adjusted by setting align
to one of LEFT
, CENTER
, or RIGHT
.
The anchor bounding box constructed for the text is as wide as the longest single text element; and, as deep as the sum of text heights of each text element; and, the height of h
used. The bounding box for all strings represented within text
can be exposed by setting debug_bounding
to true
.
Arguments:
By Position | What it does |
---|---|
text_and_sizes |
A list of one or more [text, size] pairings to produce a model of. No default. |
By Name | What it does |
---|---|
font |
The name and style of the font to use. Default: Liberation Sans
|
h |
The height (thickness) of the text produced. Default: 1
|
line_spacing |
Sets the spacing between individual lines of text; this is similar (but not identical) to leading. Default: 0.5
|
pad |
Padding applied to the boundary anchor box surrounding the generated text. Default: 0
|
align |
Horizontally align text to one of LEFT , CENTER , or RIGHT . Default: LEFT
|
spacing |
The relative spacing multiplier between characters. Default: 1
|
direction |
The text direction. ltr for left to right. rtl for right to left. ttb for top to bottom. btt for bottom to top. Default: ltr
|
language |
The language the text is in. Default: en
|
script |
The script the text is in. Default: latin
|
debug_bounding |
If set to true , the text model's bounding box will be inscribed around the produced text. Default: false
|
anchor |
Translate so anchor point is at origin [0,0,0] . Default: CENTER
|
spin |
Rotate this many degrees around the Z axis after anchoring. Default: 0
|
orient |
Vector direction to which the model should point after spin. Default: UP
|
Named Anchors: In addition to the cardinal anchor points provided by BOSL2, attachable_text3d_multisize()
vends the following six additional named anchors:
Anchor Name | Position Description |
---|---|
text-left-back |
The back-left most corner, oriented backwards |
text-left-fwd |
The forward-left most corner, oriented forwards |
text-center-back |
The back-center face, oriented backwards |
text-center-fwd |
The forward-center face, oriented forwards |
text-right-back |
The top-right most corner, oriented backwards |
text-right-fwd |
The forward-right most corner, oriented forwards |
Figure 1: Available named anchors:
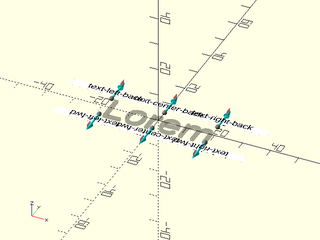
Todo:
- assert the geometry of texts_and_sizes; and make sure all [0] are texts or lists and all [1] are numbers
Example 1: A single line of attachable text:
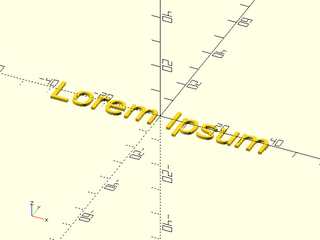
include <openscad_attachable_text3d/attachable_text3d.scad>
attachable_text3d_multisize([[["Lorem Ipsum"], 10]]);
Example 2: Multiple lines of text at various sizes:
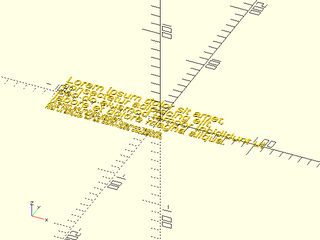
include <openscad_attachable_text3d/attachable_text3d.scad>
v = [
[["Lorem ipsum dolor sit amet,",
"consectetur adipiscing elit,",
"sed do eiusmod tempor incididunt ut",
"labore et dolore magna aliqua."], 10],
[["Ut enim ad minim veniam,",
"quis nostrud exercitation ullamco laboris",
"nisi ut aliquip ex ea commodo consequat."], 5],
];
attachable_text3d_multisize(v);
Synopsis: Returns a size listing of a given block of attachable text
Usage:
- boundary = attachable_text3d_boundary(text);
- boundary = attachable_text3d_boundary(texts);
- boundary = attachable_text3d_boundary(texts, <font="Liberation Sans">, <size=10>, <h=1>, <line_spacing=0.5>, <pad=0>);
Description:
Given a list of strings texts
, calculate the boundary sizing of all string elements in texts
and return them as a sizing boundary
, a [x-width, y-depth, h-height]
dimension. attachable_text3d_boundary()
optionally takes arguments for sizing, height, line spacing, and padding to inform the dimension returned.
font
must be a font-name and style listed in AT3D_ATTACHABLE_FONTS
.
Arguments:
By Position | What it does |
---|---|
texts |
A list of one or more text strings, or one single text string. No default. |
By Name | What it does |
---|---|
font |
The name and style of the font to use. Default: Liberation Sans
|
size |
The font size to produce text at. Default: 10
|
h |
The height (thickness) of the text produced. Default: 1
|
line_spacing |
Sets the spacing between individual lines of text; this is similar (but not identical) to leading. Default: 0.5
|
pad |
Padding applied to the boundary anchor box surrounding the generated text. Default: 0
|
spacing |
The relative spacing multiplier between characters. Default: 1
|
Example 1:
include <openscad_attachable_text3d/attachable_text3d.scad>
boundary = attachable_text3d_boundary("Ipsum");
// boundary == [36.2821, 9.56907, 1]
Synopsis: Returns a size listing of a given block of attachable multi-sized text
Usage:
- boundary_and_bounds = attachable_text3d_multisize_boundary(text_and_sizes);
- boundary_and_bounds = attachable_text3d_multisize_boundary(text_and_sizes, <font="Liberation Sans">, <h=1>, <line_spacing=0.5>, <pad=0>);
Description:
Given a list of text and sizing pairings text_and_sizes
, calculate the dimensional sizing of those pairs and return it as a list containing both sizing boundary
(a [x-width, y-depth, z-height]
dimension list), and the individual boundary elements of each entry found in text_and_sizes
. Each [text, size]
pairing within text_and_sizes
will have its boundary calculated similar to attachable_text3d_boundary()
, and the returned boundary
will have them incorporated with any line_spacing
inbetween each pairing as needed. attachable_text3d_multisize_boundary()
optionally takes arguments for height, line spacing, and padding to inform the dimension returned.
font
must be a font-name and style listed in AT3D_ATTACHABLE_FONTS
.
Arguments:
By Position | What it does |
---|---|
text_and_sizes |
A list of one or more [text, size] pairings to produce a model of. No default. |
By Name | What it does |
---|---|
font |
The name and style of the font to use. Default: Liberation Sans
|
h |
The height (thickness) of the text produced. Default: 1
|
line_spacing |
Sets the spacing between individual lines of text, and between pairings; this is similar (but not identical) to leading. Default: 0.5
|
pad |
Padding applied to the boundary anchor box surrounding the generated text. Default: 0
|
spacing |
The relative spacing multiplier between characters. Default: 1
|
Todo:
- assert the geometry of texts_and_sizes; and make sure all [0] are texts or lists and all [1] are numbers
Example 1: a multisize boundary call. Note that each individual block has its own dimension available:
include <openscad_attachable_text3d/attachable_text3d.scad>
v = [
[["Lorem Ipsum"], 10],
[["dolor sit amet", "consectetur adipiscing elit"], 5]
];
b = attachable_text3d_multisize(v);
// b == [
// [78.494, 20.6475, 1], // full boundary
// [
// [78.494, 9.56907, 1], // first block
// [77.6468, 10.5784, 1] // second block
// ]
// ]
Synopsis: Default font name: Liberation Sans
Description:
Default font for attachable_text3d
modules and functions when no font is specified.
Currently: Liberation Sans
Synopsis: Default font size: 10
Description:
Default size for attachable_text3d
modules and functions when no size is specified.
Currently: 10
Synopsis: Default font height: 1
Description:
Default height for attachable_text3d
modules and functions when no height is specified. Height is the thickness of the generated model, into the z-axis.
Currently: 1
Synopsis: Default padding to surround attachable text: 0
Description:
Default padding for attachable_text3d
modules and functions when no padding is specified. Padding is extra spacing that surrounds the entirety of a text block.
Currently: 0
Synopsis: Default spacing between lines: 0.5
Description:
Default line spacing for attachable_text3d
modules and functions when no line spacing is specified. Leading (in this library) is the spacing between multiple lines of text within the same block. It is a constant value, and not subject to change based on the specified font size, or the measured dimensions of the font face.
This is similar, though not identical, to typographical leading.
Currently: 0.5
Synopsis: Default alignment: LEFT
Description:
Default horizontal alignment for attachable_text3d
modules when no alignment is specified.
Currently: LEFT
Synopsis: Default spacing: 1
Description:
Default spacing for attachable_text3d
modules and functions when no spacing is specified.
This default is pulled from the built-intext()
, BOSL2's text3d()
, and from fontmetric.scad
's measureTextBounds()
.
Currently: 1
Synopsis: Default text direction: ltr (left-to-right)
Description:
Default direction for attachable_text3d
modules and functions when no direction is specified.
This default is pulled from the built-intext()
and BOSL2's text3d()
.
Currently: ltr
Synopsis: Default text language: en
Description:
Default language for attachable_text3d
modules and functions when no language is specified.
This default is pulled from the built-intext()
and BOSL2's text3d()
.
Currently: en
Synopsis: Default text script: latin
Description:
Default script for attachable_text3d
modules and functions when no script is specified.
This default is pulled from the built-intext()
and BOSL2's text3d()
.
Currently: latin
Synopsis: Default attachable positioning anchor: CENTER
Description:
Default anchor for attachable_text3d
modules and functions when no anchor is specified.
Currently: CENTER
Synopsis: Default attachable spin, in degrees: 0
Description:
Description:
Default spin for attachable_text3d
modules and functions when no spin is specified.
Currently: 0
Synopsis: Default attachable orientation: UP
Description:
Default orientation for attachable_text3d
modules and functions when no orient is specified.
Currently: UP
Synopsis: Sorted list of known fonts
Description:
A sorted list of all known fonts within fontmetricsdata.scad
that have sufficient measurement information so as to use them in attachable modules.
This list is dynamically generated at runtime.